
Why is main public static void method in Java?
- public: The access modifier of the class is public.
- static: The scope of the method is made to be static which means that all the member variables and the return type will be within the scope of static.
- void: This keyword in the syntax flow represents that there is no return type handled in the current method.
What does 'public static void' mean in Java?
public means that the method is visible and can be called from other objects of other types. This means that you can call a static method without creating an object of the class. void means that the method has no return value. If the method returned an int you would write int instead of void. Click to see full answer.
Can We override a private or static method in Java?
You cannot override a private or static method in Java. If you create a similar method with same return type and same method arguments in child class then it will hide the super class method; this is known as method hiding. Similarly, you cannot override a private method in sub class because it’s not accessible there.
Can we write static public void main in Java?
Yes, we can change the order of public static void main () to static public void main () in Java, the compiler doesn't throw any compile-time or runtime error. In Java, we can declare access modifiers in any order, the method name comes last, the return type comes second to last and then after it's our choice.
See more
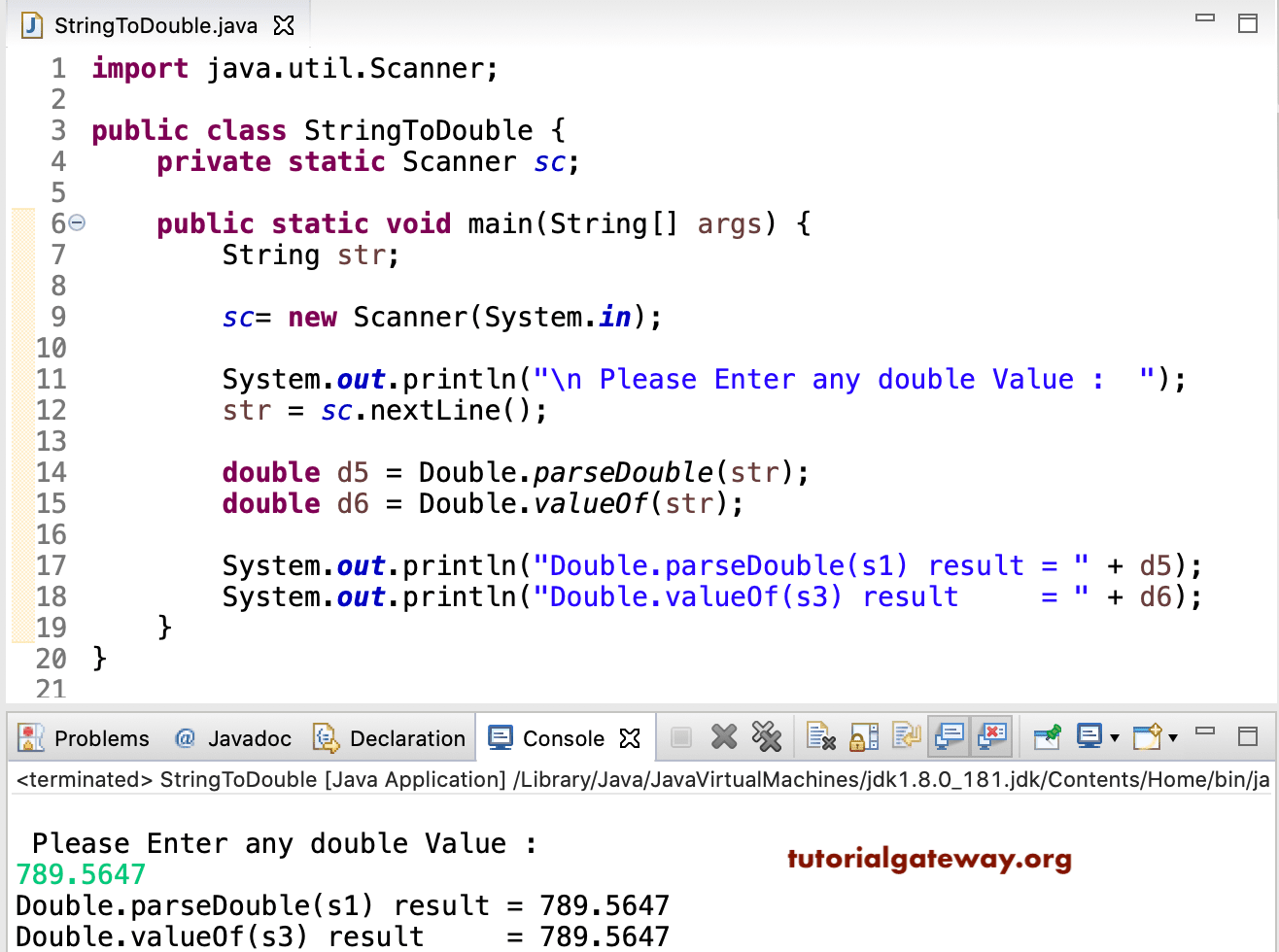
What is public void used for?
Public void : Used when you don't have to create an object and have no return. Public static void: Used when you need to create an object in the class itself . Public 'return Type' (Public int, Public String, Public double): This is used when you need to return something.
What is the difference between void and public void in Java?
public − This is the access specifier that states that the method can be accesses publically. static − Here, the object is not required to access static members. void − This states that the method doesn't return any value.
What is public void and private void?
A public method is one that can be called from outside the class in which it is declared. There are other designations -- private and protected -- that mean other things; private, for instance, designates a method that can only be called from within the class in which it is declared.
What is private void in Java?
In Java private methods are the methods having private access modifier and are restricted to be access in the defining class only and are not visible in their child class due to which are not eligible for overridden. However, we can define a method with the same name in the child class and could access in parent class.
What is static void?
static void method is a static method which does not return any thing. Static method can be called by directly by class name. void method is a method which also return nothing. But for calling simple method you have to create a class object and called method by object.
What is static in Java?
Definition and Usage. The static keyword is a non-access modifier used for methods and attributes. Static methods/attributes can be accessed without creating an object of a class.
Why private is used in Java?
The private keyword is an access modifier used for attributes, methods and constructors, making them only accessible within the declared class.
What is public/private in Java?
Public members can be accessed from child class of outside package. Private members cannot be accessed from child class of outside package. Public members can be accessed from non-child class of outside package. Private members cannot be accessed from non-child class of outside package.
Why we use static void main in Java?
When java runtime starts, there is no object of the class present. That's why the main method has to be static so that JVM can load the class into memory and call the main method. If the main method won't be static, JVM would not be able to call it because there is no object of the class is present.
Can we overload main method?
Yes, main method can be overloaded. Overloaded main method has to be called from inside the "public static void main(String args[])" as this is the entry point when the class is launched by the JVM.
What is Polymorphism in Java?
In Java, polymorphism refers to the ability of a class to provide different implementations of a method, depending on the type of object that is passed to the method. To put it simply, polymorphism in Java allows us to perform the same action in many different ways.
Can a class be static in Java?
Classes can be static which most developers are aware of, henceforth some classes can be made static in Java. Java supports Static Instance Variables, Static Methods, Static Block, and Static Classes. The class in which the nested class is defined is known as the Outer Class.
What is the difference of void and void?
The basic difference between both ( Void & void ) is that void is a primitive type while Void , a reference type that inherits from the Object .
What do public and void mean in public static void main?
public - it is access specifier means from every where we can access it. static - access modifier means we can call this method directly using class name without creating an object of it. void - its the return type. main - method name.
What is the difference between static and non static method in Java?
The static method uses compile-time or early binding. The non-static method uses runtime or dynamic binding. The static method cannot be overridden because of early binding. The non-static method can be overridden because of runtime binding.
What is private public and protected in Java?
Protected members can be accessed from the child class of the same package. Package members can be accessed from the child class of the same package. Public member can be accessed from non-child classes of the same package. Private members cannot be accessed from non-child classes of the same package.
Definition and Usage
The void keyword specifies that a method should not have a return value.
More Examples
Tip: If you want a method to return a value, you can use a primitive data type (such as int, char, etc.) instead of void, and use the return keyword inside the method:
When to use void in Java?
Void is a keyword used in many programming languages. In JAVA, void is used when you do not want your function to return anything.
When does the main method exit?
In our case the main method may exit before or after the main method has finished execution.
When can static methods be called?
Thus, static methods can be called even when the object of that class is not created.
Can public methods be called from outside classes?
You get the same effect with functions also, public methods can be called from outside the classes whereas private methods can be called only from within the classes.
Does JRE call the main method?
So JRE will call the main method, but JRE calls it from outside the class and hence public.
1. What does void mean in Java?
In Java, void keyword is used with the method declaration to specify that this particular method is not going to return any value after completing its execution. We cant assign the return type of a void method to any variable because void is not a data type.
3. When to use void method and method with return type
The most common use of void method in java is when we want to change the internal state of the object but do not require the updated state. For example in VoidWithReturnExample.java , the method setName and setAge are only used to change the name and age respectively, but they don’t return anything.
4. Download the source code
You can download the full source code of this example here: What does void mean in Java?
Where is the public keyword?
The public keyword is also found before variables (though hopefully not often), and in front of a class declaration, with similar meaning. public, private, and protected are referred to as "access modifiers", since they designate from where the affected part of a class may be accessed.
What is the difference between "active oldest votes" and "public"?
It is similar difference like between small and blue. Both words can be used together but they don't describe same property. public is access modifier - it describes where or who can use/access this portion of code. public means that this code can be used everywhere in Java applications.
What is public access?
public is access modifier - it describes where or who can use/access this portion of code. public means that this code can be used everywhere in Java applications. BTW it doesn't apply only to methods. You can specify type of access also for types (classes, enums, interfaces) and class fields (but you can't use them for local variables, that wound't make sense since their scope is limited to code-block in which they ware declared anyway)
Do variables and methods declare as public?
Variables and methods declared as public have access by other variables and methods
Can a method be declared as public void?
So it is quite common to have a method declared "public void method Name ()', for instance, indicating that it can be called from outside its own class, and that it does not return a value. "public Integer methodName ()' can be called from outside the class and returns an Integer instance, and so forth.
