
The vars () is a built-in function in Python that returns the dictionary representation (__dict__) for a module, a class, an instance, or any other object. Python vars Function: An object such as modules and instances have __dict__ attribute that can be updated, while other objects have to write restrictions.
How to initialize variables in Python?
- Method #1 : Using * operator. We can enlist all the required list comma separated and then initialize them with an empty list and multiply that empty list using the ...
- Method #2 : Using loop.
- Method #3 : Using defaultdict ()
How to create Python NumPy array with zeros?
np.zeros
- Creating Empty Arrays in Numpy. There will be times when you will have to create an empty array. ...
- Syntax
- Parameters. It takes three parameters, out of which one parameter is optional. ...
- Return Value. The np zeros () function returns an array with element values as zeros.
- Example programs on numpy.zeros () method in Python. ...
How to find type of a variable in Python?
How to find type of a variable in Python
- The type () function in Python. The type () function in Python takes an object as a parameter and returns the class type of the object.
- Finding the type of a variable. Let us first declare a few variables/objects to find their class types. ...
- Python code to check data type of a variable. ...
How do I declare a function in Python?
In Python, by convention, you should name a function using lowercase letters with words separated by an underscore, such as do_something(). These conventions are described in PEP 8 , which is Python’s style guide.

What do VAR () functions do?
Estimates variance based on a sample.
What is Getattr Python?
Python getattr() function is used to access the attribute value of an object and also gives an option of executing the default value in case of unavailability of the key. Syntax : getattr(obj, key, def)
What does vars do in R?
Description. Just like aes() , vars() is a quoting function that takes inputs to be evaluated in the context of a dataset. These inputs can be: variable names.
What is the use of __ dict __ in Python?
The __dict__ in Python represents a dictionary or any mapping object that is used to store the attributes of the object. They are also known as mappingproxy objects. To put it simply, every object in Python has an attribute that is denoted by __dict__.
Is Getattr slow?
Yes, compared to the direct conventional method of accessing an attribute of a given object, the performance of getattr() is slower.
What are Python magic methods?
Magic methods in Python are the special methods that start and end with the double underscores. They are also called dunder methods. Magic methods are not meant to be invoked directly by you, but the invocation happens internally from the class on a certain action.
How do you call a variable in R?
To call a variable from a data set, you have two options:You can call var1 by first specifying the name of the data set (e.g., mydata ): > mydata$var1 > mydata[,"var1"]Alternatively, use the function attach() , which attaches the data set to the R search path. You can now refer to variables by name.
How do I create a variable in R?
Rules for R variables are:A variable name must start with a letter and can be a combination of letters, digits, period(.) ... A variable name cannot start with a number or underscore (_)Variable names are case-sensitive (age, Age and AGE are three different variables)More items...
How do you set a variable in R?
In R, assigning values to a variable can be specified or achieved using the syntax of left angular brackets signifying the syntax of an arrow. After assigning values to a variable, these values can be printed using the predefined cat() function and print() function.
What is __ slots __?
The __slots__ declaration allows us to explicitly declare data members, causes Python to reserve space for them in memory, and prevents the creation of __dict__ and __weakref__ attributes. It also prevents the creation of any variables that aren't declared in __slots__.
What is __ init __ in Python?
The __init__ method is the Python equivalent of the C++ constructor in an object-oriented approach. The __init__ function is called every time an object is created from a class. The __init__ method lets the class initialize the object's attributes and serves no other purpose. It is only used within classes.
What is __ call __ Python?
__call__ in Python Python has a set of built-in methods and __call__ is one of them. The __call__ method enables Python programmers to write classes where the instances behave like functions and can be called like a function.
What does the __ Init__ function do in Python?
The __init__ function is called every time an object is created from a class. The __init__ method lets the class initialize the object's attributes and serves no other purpose. It is only used within classes.
Does Getattr return methods?
Python getattr() The getattr() method returns the value of the named attribute of an object. If not found, it returns the default value provided to the function.
Is Isinstance a Python?
isinstance() is a built-in Python method that allows you to verify a particular value's data type. For example, you can use isinstance() to check if a value is a string or a list.
What is Hasattr OBJ name used for?
Python hasattr() function is an inbuilt utility function, which is used to check if an object has the given named attribute and return true if present, else false.
Definition
The Python vars () function is used to retrieve the __dict__ attribute for the specified module, class, instance, or any other object with a __dict__ attribute.
Python vars ()
The Python vars () method is a part of Python’s standard library collection of built-in functions. It returns the name: value pair mappings for all names defined in the local scope or the optional object argument’s scope along with their associated values.
Python vars () parameters
The vars () method accepts only one parameter, which is also optional. It accepts an object as a parameter.
Example: Working of Python vars ()
Let us look at few examples where Python vars () is applicable and understand this inbuilt function in detail.
Frequently Asked Questions
The Python vars () function is used to retrieve the __dict__ attribute for the specified module, class, instance, or any other object with a __dict__ attribute. It returns the name: value pair mappings for all names defined in the local scope or the optional object argument’s scope along with their associated values i.e.
What is vars in Python?
Hello, programmers; in today’s article, we will discuss all you need to know about the vars () function in python. Python vars () is a function that returns the __dict__ attribute of an object. Before we go into the topic’s depth, let me just brief you on what are __dict__ and object in the vars () function.
What is the math module passed as an argument?
As shown above, the math module is passed as an argument to the vars () function—the output prints all the math module’s built-in functions as its dictionary attributes.
Can you use vars in Python?
In this article, we learned to implement vars function in different cases in Python. The above algorithm and code snippets with the vars function can be used whenever needed.
What are the rules for creating a variable in Python?
Rules for creating variables in Python: A variable name must start with a letter or the underscore character. A variable name cannot start with a number. A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ ). Variable names are case-sensitive (name, Name and NAME are three different variables).
What does Python do when it looks at the first statement?
When Python looks at the first statement, what it does is that, first, it creates an object to represent the value 5. Then, it creates the variable x if it doesn’t exist and made it a reference to this new object 5. The second line causes Python to create the variable y, and it is not assigned with x, rather it is made to reference that object that x does. The net effect is that the variables x and y wind up referencing the same object. This situation, with multiple names referencing the same object, is called a
How does shared reference work in Python?
The second line causes Python to create the variable y, and it is not assigned with x, rather it is made to reference that object that x does. The net effect is that the variables x and y wind up referencing the same object. This situation, with multiple names referencing the same object, is called a Shared Reference in Python.#N#Now, if we write:
What is global keyword in Python?
Global keyword in Python: Global keyword is a keyword that allows a user to modify a variable outside of the current scope. It is used to create global variables from a non-global scope i.e inside a function. Global keyword is used inside a function only when we want to do assignments or when we want to change a variable.
What characters can be used in a variable name?
A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ ).
What is data type in Python?
Data types are the classification or categorization of data items. It represents the kind of value that tells what operations can be performed on a particular data. Since everything is an object in Python programming, data types are actually classes and variables are instance (object) of these classes.
Can you re-declare a Python variable?
We can re-declare the python variable once we have declared the variable already.
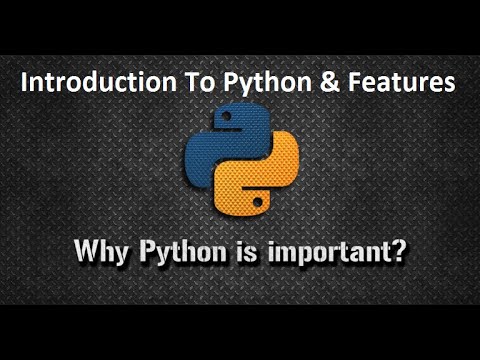
What Is A variable?
Python Variable
- A Python variable is a container that stores values. Python does not have any commands to declare variables. In Java, C++, or C#, first we have to define a variable and fix its data type. like int a, float b, string str, etc. However, in Python, we are not concerned with data types because variables choose their data types when we apply a value to ...
Variable Naming Rules
- A variable name must start with a letter or the underscore.
- A variable name can not start with a number.
- A variable name is case-sensitive. means Total and total are different.
- A variable only contains the alphabet, numbers, and underscore.
Variable Type
- We can know the datatype of a variable by using the type() function in Python. Example Output Hello World 25 <class 'str'> <class 'int'>