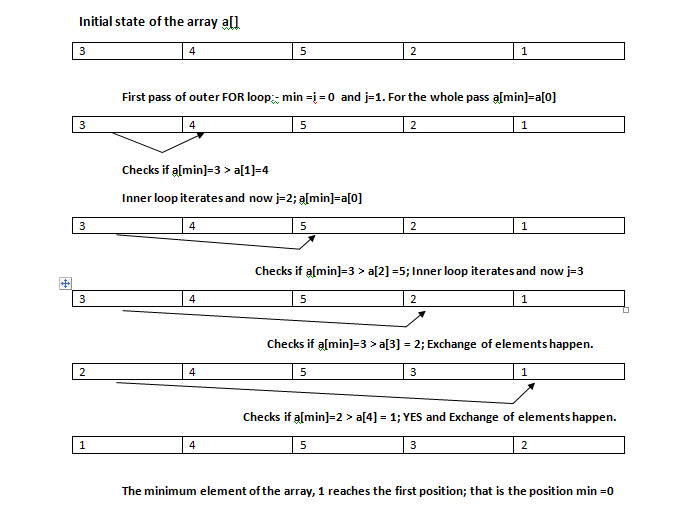
What is a selection sort?
Selection Sort in Java with examples of fibonacci series, armstrong number, prime number, palindrome number, factorial number, bubble sort, selection …
What is selection sort method?
Jan 31, 2014 · The selection sort algorithm sorts an array by repeatedly finding the minimum element (considering ascending order) from unsorted part and putting it at the beginning. The algorithm maintains two subarrays in a given array. 1) The subarray which is already sorted. 2) Remaining subarray which is unsorted.
How does the selection sort work?
Jun 26, 2020 · What is selection sort in Java with example? Selection sort is an in-place comparison sort . It loops and find the first smallest value, swaps it with the first element; loop and find the second smallest value again, swaps it with the second element, repeats third, fourth, fifth smallest values and swaps it, until everything is in correct order.
What is sort algorithm in Java?
What is selection sort with example? Selection sort is a simple sorting algorithm. The smallest element is selected from the unsorted array and swapped with the leftmost element, and that element becomes a part of the sorted array. This process continues moving unsorted array boundary by one element to the right.
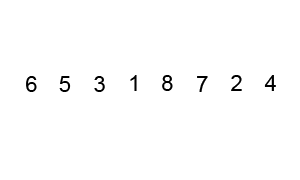
What is selection sort with an example?
Example of Selection Sort The smallest number from 5 2 and 1 is 1. So, we replace 10 by 1. The new array is [1,5,2,10] Again, this process is repeated. Finally, we get the sorted array as [1,2,5,10].Mar 29, 2022
What is a selection sort in Java?
Selection sort is a simple sorting algorithm. This sorting algorithm is an in-place comparison-based algorithm in which the list is divided into two parts, the sorted part at the left end and the unsorted part at the right end. Initially, the sorted part is empty and the unsorted part is the entire list.Apr 25, 2018
How do you write a selection sort in Java?
Selection Sort Method in Javaint n = array.length; // Loop to increase the boundary of the sorted array. ... { // Finding the smallest element in the unsorted array. ... for ( int j = i+ 1 ; j < n; j++) ... /* Swap the smallest element from the unsorted array with the last element of the sorted array */ ... array[i] = temp;Jun 17, 2021
What are the steps for selection sort?
Algorithm for Selection SortStep 1: For i = 1 to n-1.step 2: Set min = arr[i]step 3: Set position = i.step 4: For j = i+1 to n-1 repeat:if (min > arr[j])Set min = arr[j]Set position = j.[end of if]More items...
What is meant by selection sort?
Selection sort works by taking the smallest element in an unsorted array and bringing it to the front. You'll go through each item (from left to right) until you find the smallest one. The first item in the array is now sorted, while the rest of the array is unsorted.Oct 19, 2021
Is selection sort divide and conquer?
Bubble sort may also be viewed as a k = 2 divide- and-conquer sorting method. Insertion sort, selection sort and bubble sort divide a large instance into one smaller instance of size n - 1 and another one of size 1. All three sort methods take O(n2) time.
Why is selection sort O n 2?
Because it treats all data sets the same and has no ability to short-circuit the rest of the sort if it ever comes across a sorted list before the algorithm is complete, insertion sort has no best or worst cases. Selection sort always takes O(n2) operations, regardless of the characteristics of the data being sorted.
Is a selection sort stable?
NoSelection sort / Stable
What is selection sort and bubble sort?
Definition. Bubble sort is a simple sorting algorithm that continuously steps through the list and compares the adjacent pairs to sort the elements. In contrast, selection sort is a sorting algorithm that takes the smallest value (considering ascending order) in the list and moves it to the proper position in the array ...Dec 10, 2018
Is selection sort faster than bubble sort?
Selection sort is faster than Bubble sort because Selection sort swaps elements "n" times in worst case, but Bubble sort swaps almost n*(n-1) times.May 20, 2017
Is selection sort greedy?
A selection sort could indeed be described as a greedy algorithm, in the sense that it: tries to choose an output (a permutation of its inputs) that optimizes a certain measure ("sortedness", which could be measured in various ways, e.g. by number of inversions), and.Nov 11, 2017
Selection sort complexity
Now, let's see the time complexity of selection sort in best case, average case, and in worst case. We will also see the space complexity of the selection sort.
Implementation of selection sort
Now, let's see the programs of selection sort in different programming languages.
How does selection sort work?
The selection sort algorithm sorts an array by repeatedly finding the minimum element (considering ascending order) from unsorted part and putting it at the beginning. The algorithm maintains two subarrays in a given array.
What is the subarray in selection sort?
In every iteration of selection sort, the minimum element (considering ascending order) from the unsorted subarray is picked and moved to the sorted subarray.
How to find the smallest element in a sorted array?
The elements in Bold Blue color will be as part of the sorted array. Step 1: Set the MIN pointer to the first location. So, the MIN pointer points to 15. Smallest: = 15. Step 2: Find the smallest element by comparing it with the rest of the elements. Comparing 15 and 21, 15 is the smallest.
How does selection sort work?
Selection sort works in a simple manner where it keeps two subarrays from the input array. They are: 1 Sorted subarray to keep the sorted elements 2 Unsorted subarray to keep the unsorted elements.
What is the minimum number of swaps in selection sort?
Answer: The selection sort technique takes the minimum number of swaps. For the best case, when the array is sorted, the number of swaps in the selection sort is 0.
How does selection sort work?
Answer: Selection sort works by maintaining two sub-arrays. The minimum element from the unsorted subarray is placed in its proper position in a sorted sub-array. Then the second-lowest element is placed in its proper position. This way, the entire array is sorted by selecting a minimum element during each iteration.
What is selection sort?
The selection sort technique is a method in which the smallest element in the array is selected and swapped with the first element of the array. Next, the second smallest element in the array is exchanged with the second element and vice versa.
What is the routine to find the smallest number?
As you can see, the routine to find the smallest number is called while traversing the data set. Once the smallest element is found, it is placed in its desired position.
What is selection sort?
Selection Sort is a simple and slow sorting algorithm that repeatedly selects the lowest or highest element from the un-sorted section and moves it to the end of the sorted section. Mostly, performance wise, it is slower than even Insertion Sort. It does not adapt to the data in any way so its runtime is always quadratic.
Is selection sort quadratic?
It does not adapt to the data in any way so its runtime is always quadratic. But you should not conclude that selection sort should never be used. Good thing is that selection sort has the property of minimizing the number of swaps with each iteration.
What is the subarray in selection sort?
2) Remaining subarray which is unsorted. In every iteration of selection sort, the minimum element (considering ascending order) from the unsorted subarray is picked and moved to the sorted subarray. Following example explains the above steps:
How does selection sort work?
The selection sort algorithm sorts an array by repeatedly finding the minimum element (considering ascending order) from unsorted part and putting it at the beginning. The algorithm maintains two subarrays in a given array.
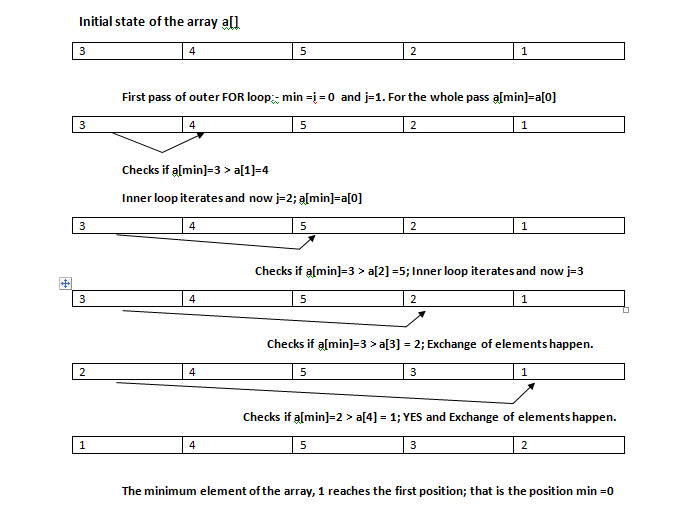
Working of Selection Sort Algorithm
- Now, let's see the working of the Selection sort Algorithm. To understand the working of the Selection sort algorithm, let's take an unsorted array. It will be easier to understand the Selection sort via an example. Let the elements of array are - Now, for the first position in the sorted array, the entire array is to be scanned sequentially. At pr...
Selection Sort Complexity
- Now, let's see the time complexity of selection sort in best case, average case, and in worst case. We will also see the space complexity of the selection sort.
Implementation of Selection Sort
- Now, let's see the programs of selection sort in different programming languages. Program:Write a program to implement selection sort in C language. Output: After the execution of above code, the output will be - Program:Write a program to implement selection sort in C++ language. Output: After the execution of above code, the output will be - Program:Write a program to implement se…
How Selection Sort Works in Java
Examples to Implement Selection Sort in Java
Performance of Selection Sort
Conclusion
- The selection sort does not work efficiently on large lists as it consumes more time for comparison. Selection sort is a method in which an input array will be divided into two subarrays in order to keep them sorted and unsorted elements. The minimum element in the array will be swapped with the element in the first position, and the process contin...
Recommended Articles