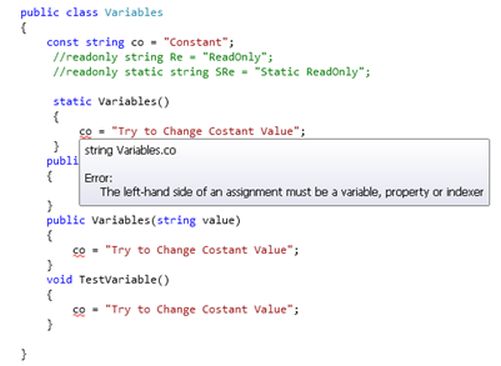
So just in case you missed the differences, here they are:
- var declarations are globally scoped or function scoped while let and const are block scoped.
- var variables can be updated and re-declared within its scope; let variables can be updated but not re-declared; const variables can neither be updated nor re-declared.
- They are all hoisted to the top of their scope. ...
What is the difference between const and let in JavaScript?
As a general rule, you should always declare variables with const, if you realize that the value of the variable needs to change, go back and change it to let. The scope of a var variable is functional scope. The scope of a let variable is block scope.
What is the difference between let and const in Python?
64 The difference between letand constis that once you bind a value/object to a variable using const, you can't reassign to that variable. In other words Example:
What is the difference between Var and let and const?
But while var variables are initialized with undefined, let and const variables are not initialized. While var and let can be declared without being initialized, const must be initialized during declaration. Got any question or additions?
What is the scope of a let and const variable?
The scope of a let variable is block scope. The scope of a const variable is block scope. It can be updated and re-declared into the scope. It can be updated but cannot be re-declared into the scope. It cannot be updated or re-declared into the scope.
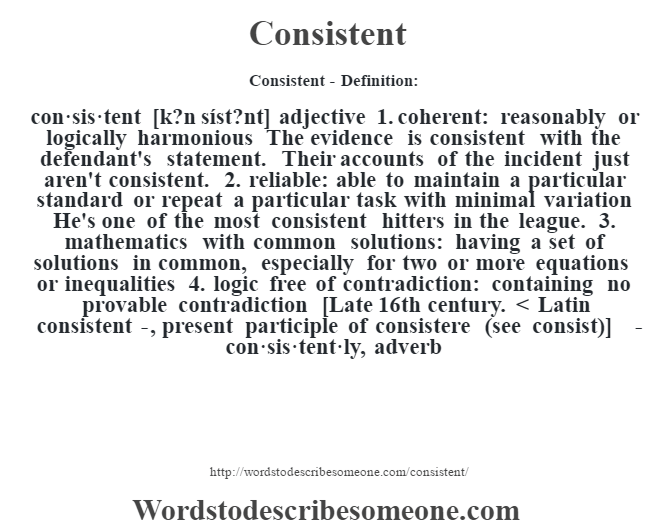
What is the difference between Let & const?
It means variables defined outside the function can be accessed globally, and variables defined inside a particular function can be accessed within the function....Javascript.varletconstIt can be declared without initialization.It can be declared without initialization.It cannot be declared without initialization.3 more rows•Dec 15, 2021
Should I use let or const?
As a general rule, you should always declare variables with const, if you realize that the value of the variable needs to change, go back and change it to let. Use let when you know that the value of a variable will change. Use const for every other variable.
Is const faster than let?
The execution context underlying how the JavaScript interpreter runs the code is basically the same when you use var compared to when you use let and const . That results in the same execution speed.
What is == and === in JavaScript?
The main difference between the == and === operator in javascript is that the == operator does the type conversion of the operands before comparison, whereas the === operator compares the values as well as the data types of the operands.
Which is better let or VAR?
let allows you to declare variables that are limited in scope to the block, statement, or expression on which it is used. This is unlike the var keyword, which defines a variable globally, or locally to an entire function regardless of block scope.
Why is var better than const?
var declarations are globally scoped or function scoped while let and const are block scoped. var variables can be updated and re-declared within its scope; let variables can be updated but not re-declared; const variables can neither be updated nor re-declared. They are all hoisted to the top of their scope.
What is the difference between LET and VAR?
let is block-scoped. var is function scoped. let does not allow to redeclare variables. var allows to redeclare variables.
Can var be changed?
In the global context, a variable declared using var is added as a non-configurable property of the global object. This means its property descriptor cannot be changed and it cannot be deleted using delete .
When to use let and const in JavaScript?
`const` is a signal that the identifier won't be reassigned. `let` is a signal that the variable may be reassigned, such as a counter in a loop, or a value swap in an algorithm. It also signals that the variable will be used only in the block it's defined in, which is not always the entire containing function.
Should I use const on objects?
let and const are meant for type safety. There is no situation where you must use them, but they can be handy and reduce hard to spot bugs. One example of a situation where const would be useful for an object that you don't want to turn into another type.
Is let necessary in JavaScript?
The first thing we've learnt is that for most purposes, var and let aren't strictly necessary in JavaScript. Roughly speaking, scoping constructs with lexical scope can be mechanically transformed into functional arguments.
When to use let and VAR in JavaScript?
let allows you to declare variables that are limited to the scope of a block statement, or expression on which it is used, unlike the var keyword, which declares a variable globally, or locally to an entire function regardless of block scope.
How are let and const similar?
The JavaScript let and const keywords are quite similar , in that they create block-level scope. However, they do differ a bit in the way that they behave. For example, the JavaScript let keyword is similar to the var keyword in that assignments can be changed. On the other hand, the JavaScript const keyword differs in that assignments can not be ...
What is the difference between a let and a const in JavaScript?
What is the difference between LET and CONST in JavaScript? The JavaScript let and const keywords provide block-level scope, but there is a slight difference in how they behave. With const, you can not re-assign a value to the variable.
What is let and const in JavaScript?
So to recap, we now know that the JavaScript let and const keywords allow you to create block-level scope for variables , which, in turn, negates the need to always use functions to create scope. With block-level scope, all you need are the curly braces “ { }”, and within that block, any variable created using let or const is private (or local) to that block. This is particularly helpful with for-loops. And a very important thing to keep in mind: with const, you cannot re-assign values to a variable. In other words, any variable created with the const keyword is a constant and the assignment cannot be changed.
What is the output of a second console.log statement?
Take a look at Example # 1 B. Notice how, in the second console.log () statement, the output is 100. This is because that second console.log () statement is in the global scope, and in that scope the “i” variable is equal to 100. So, there we have it: two different scopes without even having used a function.
Can you re-assign a variable with const?
And a very important thing to keep in mind: with const, you cannot re-assign values to a variable. In other words, any variable created with the const keyword is a constant and the assignment cannot be changed.
Can you mutate a variable using the const keyword?
On the other hand, the JavaScript const keyword differs in that assignments can not be changed. So, once you declare a variable using the const keyword, you cannot re-assign a value to that variable. This does not mean that the variable is immutable. If you assign an object to a variable using the const keyword, you can mutate that object.
What keyword to use to declare a variable in JavaScript?
So, users should use the let and const keyword to declare a variable in JavaScript.
What is scope in a function?
Scope: Global scoped or function scoped. The scope of the var keyword is the global or function scope. It means variables defined outside the function can be accessed globally, and variables defined inside a particular function can be accessed within the function.
What is the oldest keyword in JavaScript?
var keyword in JavaScript: The var is the oldest keyword to declare a variable in JavaScript.
What are the three keywords used in JavaScript?
In JavaScript, users can declare a variable using 3 keywords that are var, let, and const. In this article, we will see the differences between the var, let, and const keywords. We will discuss the scope and other required concepts about each keyword.
What is scope in let?
Scope: block scoped: The scope of a let variable is only block scoped. It can’t be accessible outside the particular block ( {block}). Let’s see the below example.
Can you declare the same variable in different blocks?
Example 4: Users can declare the variable with the same name in different blocks using the let keyword.
Can a user re-declare a variable?
Example 3: User can re-declare variable using var and user can update var variable . The output is shown in the console.
Variable Declaration in JavaScript
In the early days of JavaScript, there was only one way to declare variables and that was using the var keyword. However, there were some issues associated with variables declared using the var keyword so it was necessary to evolve new ways for variable declaration.
var, let, and const Variable Declaration in JavaScript
The differences between var, let, and const variable declaration in JavaScript include: Variables declared with var and const are scoped to the immediate function body. Variables declared with the var keyword are hoisted.
How to Use the var Keyword in JavaScript
The var keyword has traditional variable declaration syntax. It is optional to initialize a variable with a value if it is declared with the var keyword. If developers do not initialize it with a value, JavaScript will automatically assign undefined to it, as shown in the following code snippet:
The let Keyword in JavaScript
let is the improved version of var. let eliminates all the issues of var that we have talked about in the previous section. let has a similar syntax to var but has a more strict scope.
The const Keyword in JavaScript
The const keyword follows the same rules as the let keyword. The only difference with const is that const is used to define only constant values in JavaScript programs.
What is Scope?
Scope determines the accessibility or visibility of variables to JavaScript. There are three types of scope in JavaScript:
const
Const is quite similar to Let with the only difference that once the variable has been defined then its value cannot be changed. Like if the user tries to change the value of the const variable, then they will get an error.
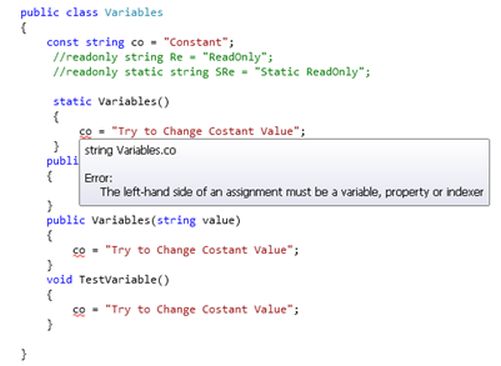
Example # 1 B
Example # 2 B
- Now, in Example # 2 A, there are two“j” variables. The first “j” variable is a global, equal to 100, and the second is defined inside of the for loop. And because it’s defined inside of a block, it has block-level scope. Now look at example # 2 B. Because “i” is global, the “i” variable increments, just as we would expect. But notice that the“j” variable is always 50 in each console.log() statem…
Example # 3 B
- In Examples # 3 A and # 3 B you’ll see a similarity to Examples # 1 A and # 1 B, the only difference being the use of the use of the const keyword instead of let when declaring our block-level version of the“i”variable.
Example # 4 B
- Now here in Example # 4 A, we’ve run into a problem. We tried to take the same approach as Example # 2 A, that is, we tried to increment the “j” variable declared on line # 6. The problem, though, is that when you use the JavaScript const keyword, you cannot re-assign a new value to a variable. So when you look at Example # 4 B, you’ll see that we never see the full output of the f…
Example # 5 B
- Now Example # 5 A is virtually identical to Example # 4 A, except that we have not tried to increment the“j” variable. And when you look at Example # 5 B, you’ll see that we no longer have an error. In the console, the value of “j” is 50every time.
Summary
- So to recap, we now know that the JavaScript let and const keywords allow you to create block-level scope for variables, which, in turn, negates the need to always use functions to create scope. With block-level scope, all you need are the curly braces “{ }”, and within that block, any variable created using let or const is private (or local) to th...