
What’s the difference between persist and merge? You should always use persist for new entities, while merge is required for detached entities. Because Hibernate automatically synchronizes the entity state with the underlying database record, you don’t need any save method for managed entities.
Full Answer
What is the difference between hibernate merge and update?
As with persist and save, the update method is an “original” Hibernate method that was present long before the merge method was added. Its semantics differs in several key points: it acts upon passed object (its return type is void); the update method transitions the passed object from detached to persistent state;
What is the difference between merge() and persist()?
merge (entity) should be used, to put entity back to persistence context if the entity was detached and was changed. Persist should be called only on new entities, while merge is meant to reattach detached entities. If you're using the assigned generator, using merge instead of persist can cause a redundant SQL statement.
What is the difference between hibernate’s Save and persist methods?
JPA’s persist method returns void and Hibernate’s save method returns the primary key of the entity. That might seem like a huge difference, especially when you take a closer look at Hibernate’s Javadoc and the JPA specification:
What is the difference between JPA and hibernate?
JPA and Hibernate provide different methods to persist new and to update existing entities. You can choose between JPA’s persist and merge and Hibernate’s save and update methods.
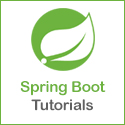
What is difference between persist and merge in hibernate?
ANSWER: Either way will add an entity to a PersistenceContext, the difference is in what you do with the entity afterwards. Merge creates a new instance of your entity, copies the state from the supplied entity, and makes the new copy managed.
When to Use merge and persist?
Persist takes an entity instance, adds it to the context and makes that instance managed (i.e. future updates to the entity will be tracked). Merge returns the managed instance that the state was merged with. It does return something that exists in PersistenceContext or creates a new instance of your entity.
What is the difference between Merge and persist in JPA?
Difference between persist() and merge() method. Using merge() method we can create/save a new record as well as we can update an existing record. 2. The return type of the persist() method is void whereas the merge() method returns a managed entity.
What is difference between Merge and update in hibernate?
Hibernate handles persisting any changes to objects in the session when the session is flushed. update can fail if an instance of the object is already in the session. Merge should be used in that case. It merges the changes of the detached object with an object in the session, if it exists.
What is the difference between Save () and persist () method in hibernate?
Difference between save and persist method in Hibernate First difference between save and persist is there return type. Similar to save method persist also INSERT records into database but return type of persist is void while return type of save is Serializable object.
What is difference between save and saveOrUpdate in hibernate?
Difference between save and saveOrUpdate in Hibernate The main difference between save and saveOrUpdate method is that save() generates a new identifier and INSERT record into the database while saveOrUpdate can either INSERT or UPDATE based upon the existence of a record.
What is lazy loading in hibernate?
Hibernate now can "lazy-load" the children, which means that it does not actually load all the children when loading the parent. Instead, it loads them when requested to do so. You can either request this explicitly or, and this is far more common, hibernate will load them automatically when you try to access a child.
What is dirty checking in hibernate?
Hibernate monitors all persistent objects. At the end of a unit of work, it knows which objects have been modified. Then it calls update statement on all updated objects. This process of monitoring and updating only objects that have been changed is called automatic dirty checking in hibernate.
What is difference between save and Saveflush?
On saveAndFlush , changes will be flushed to DB immediately in this command. With save , this is not necessarily true, and might stay just in memory, until flush or commit commands are issued.
What is difference between getCurrentSession () and openSession () in Hibernate?
openSession() always opens a new session that you have to close once you are done with the operations. SessionFactory. getCurrentSession() returns a session bound to a context - you don't need to close this.
What is the difference between lazy and eager loading in Hibernate?
Eager Loading is a design pattern in which data initialization occurs on the spot. Lazy Loading is a design pattern that we use to defer initialization of an object as long as it's possible.
What is MERGE () in Hibernate?
session. The merge() method is used when we want to change a detached entity into the persistent state again, and it will automatically update the database. The main aim of the merge() method is to update the changes in the database made by the persistent object.
What is difference between EntityManagerFactory and SessionFactory?
Using EntityManagerFactory approach allows us to use callback method annotations like @PrePersist, @PostPersist,@PreUpdate with no extra configuration. Using similar callbacks while using SessionFactory will require extra efforts.
What does EntityManager persist do?
The EntityManager. persist() operation is used to insert a new object into the database. persist does not directly insert the object into the database: it just registers it as new in the persistence context (transaction).
What is the benefit of using JPA over Hibernate API?
As you've seen, JPA provides an easy to use API to implement common CRUD use cases without writing any SQL. That makes the implementation of common use cases a lot faster, but it also provides another benefit: Your SQL statements are not spread all over your code.
How does merge work in JPA?
JPA's merge method copies the state of a detached entity to a managed instance of the same entity. Hibernate, therefore, executes an SQL SELECT statement to retrieve a managed entity from the database.
What is the difference between JPA and Hibernate?
Another obvious difference between these 2 methods is their return type. JPA’s persist method returns void and Hibernate’s save method returns the primary key of the entity.
When to use INSERT in Hibernate?
If you use the IDENTITY strategy to generate the primary key value, Hibernate needs to execute the INSERT statement when you call the save or persist method to retrieve the primary key value from the database.
What is the purpose of Hibernate update?
You can use Hibernate’s update or JPA’s merge method to associate a detached entity with a persistence context. After you’ve done that, Hibernate will update the database based on the entity attribute values.
Why does save and persist behave differently?
You could expect that the save and persist method behave differently because there are a few differences between the JPA specification and the Javadoc of Hibernate’s proprietary methods.
What is JPA and hibernate?
JPA and Hibernate provide different methods to persist new and to update existing entities. You can choose between JPA’s persist and merge and Hibernate’s save and update methods.
Why are entities in the detached lifecycle state no longer managed by the persistence context?
That can be the case because you closed the persistence context or you explicitly detached the entity from the current context. I will get into more details about how you can reattach these entities with JPA’s merge and Hibernate’s update methods in a later part of this post.
What is JPA merge?
JPA’s merge method. JPA’s merge method copies the state of a detached entity to a managed instance of the same entity. Hibernate, therefore, executes an SQL SELECT statement to retrieve a managed entity from the database.
Why do you need to stick to persist and merge?
If you don't have any special requirements, as a rule of thumb, you should stick to the persist and merge methods, because they are standardized and guaranteed to conform to the JPA specification.
What is the problem with Hibernate?
The notion of “persistence context” is Hibernate's solution to this problem. Persistence context can be thought of as a container or a first-level cache for all the objects that you loaded or saved to a database during a session.
What happens after deserializing an entity instance?
After deserializing this entity instance, you need to get a persistent entity instance from a persistence context and update its fields with new values from this detached instance. So the merge method does exactly that:
What type of return is the persist method?
Notice that the persist method has void return type. It operates on the passed object “in place”, changing its state. The person variable references the actual persisted object.
What is the main intention of the merge method?
The main intention of the merge method is to update a persistent entity instance with new field values from a detached entity instance.
What happens when an entity instance is in a persistent state?
When the entity instance is in the persistent state, all changes that you make to the mapped fields of this instance will be applied to the corresponding database records and fields upon flushing the Session. The persistent instance can be thought of as “online”, whereas the detached instance has gone “offline” and is not monitored for changes.
What is a transient instance?
transient — this instance is not, and never was, attached to a Session; this instance has no corresponding rows in the database; it's usually just a new object that you have created to save to the database;
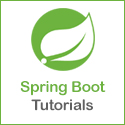