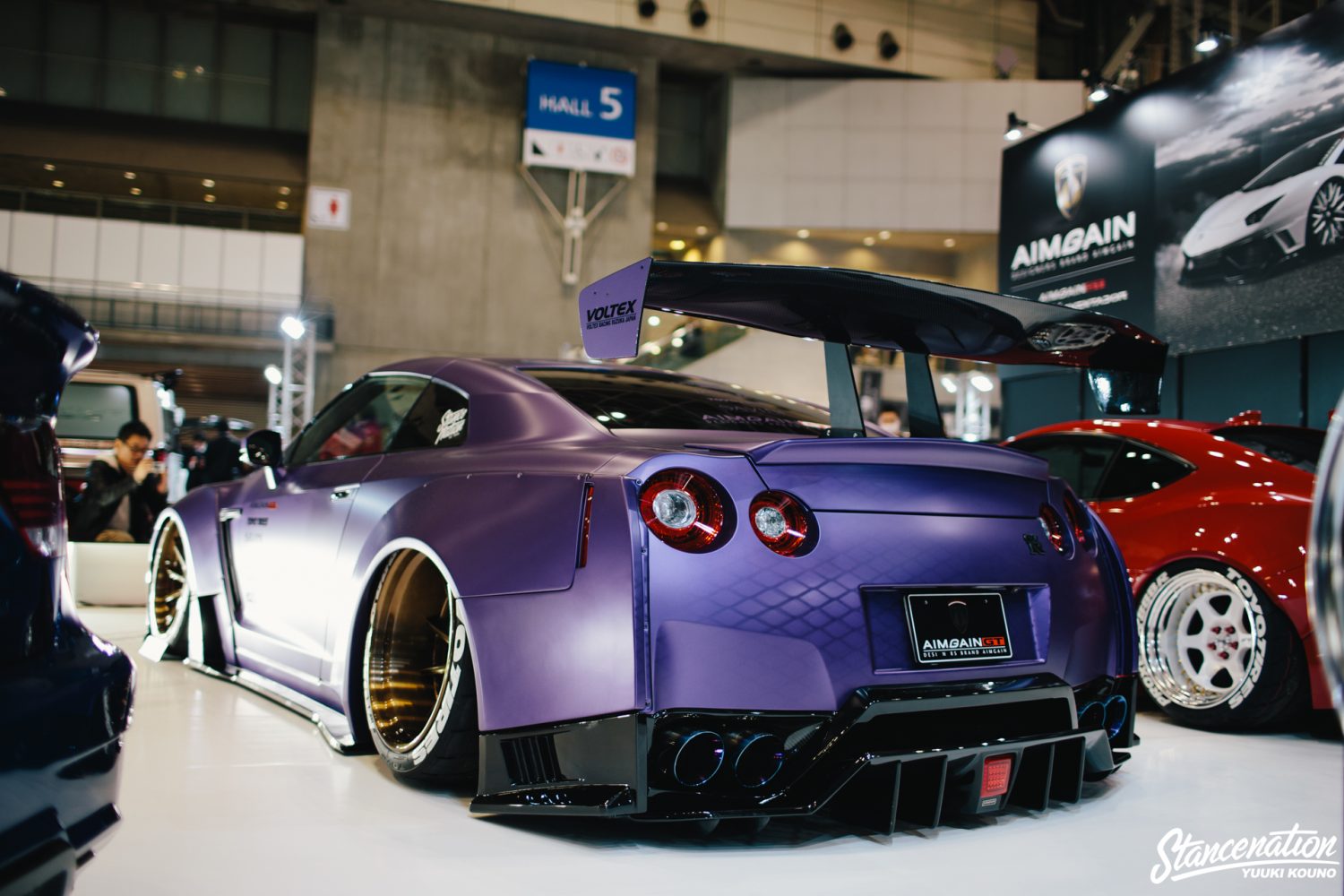
What are the disadvantages of function in C?
The primary disadvantage of using functions is that using functions means that you’re writing code that might actually work instead of forever talking about writing in C and never actually doing so. is a function. Complexity of the program increases. The complexity of the program increases. execution speed decreases.
What are the different types of functions in C?
There are four types of user-defined functions in C++:
- No return type, with no Arguments.
- Return type, with no Arguments.
- No return type, with Arguments.
- Return type, with Arguments.
How to write a function in C?
Simple example program for C function:
- As you know, functions should be declared and defined before calling in a C program.
- In the below program, function “square” is called from main function.
- The value of “m” is passed as argument to the function “square”. ...
What are the functions inside function in C?
lambda expressions
- Naming. Local functions are explicitly named like methods. ...
- Function signatures and lambda expression types. Lambda expressions rely on the type of the Action / Func variable that they're assigned to determine the argument and return types.
- Definite assignment. ...
- Implementation as a delegate. ...
- Variable capture. ...
- Heap allocations. ...
- Usage of the yield keyword. ...
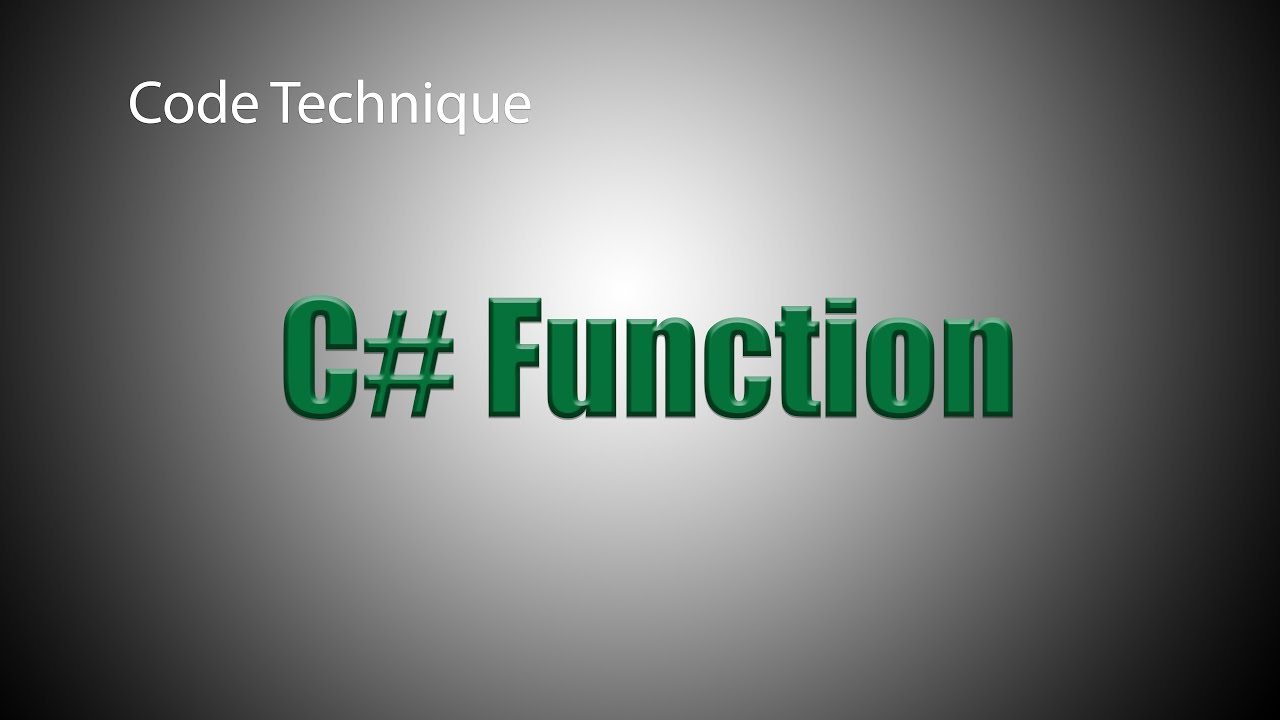
What is the main function of C?
The Main Function In C, the "main" function is treated the same as every function, it has a return type (and in some cases accepts inputs via parameters). The only difference is that the main function is "called" by the operating system when the user runs the program.
What is function in C with example?
Function AspectsSNC function aspectsSyntax1Function declarationreturn_type function_name (argument list);2Function callfunction_name (argument_list)3Function definitionreturn_type function_name (argument list) {function body;}
What are the 4 types of functions in C?
There are 4 types of functions:Functions with arguments and return values. This function has arguments and returns a value: ... Functions with arguments and without return values. ... Functions without arguments and with return values. ... Functions without arguments and without return values.
What are the 4 types of functions?
The types of functions can be broadly classified into four types. Based on Element: One to one Function, many to one function, onto function, one to one and onto function, into function.
What is a function in C?
A function is a group of statements that together perform a task. Every C program has at least one function, which is main (), and all the most trivial programs can define additional functions.
How to use a function in C?
While creating a C function, you give a definition of what the function has to do. To use a function, you will have to call that function to perform the defined task. When a program calls a function, the program control is transferred to the called function. A called function performs a defined task and when its return statement is executed or ...
What is the parameter list in a function?
The parameter list refers to the type, order, and number of the parameters of a function. Parameters are optional; that is, a function may contain no parameters. Function Body − The function body contains a collection of statements that define what the function does.
What are formal parameters in a function?
These variables are called the formal parameters of the function. Formal parameters behave like other local variables inside the function and are created upon entry into the function and destroyed upon exit.
What is a function declaration?
A function declaration tells the compiler about the number of parameters function takes, data-types of parameters and return type of function. Putting parameter names in function declaration is optional in the function declaration, but it is necessary to put them in the definition.
What is a set of statements that take inputs, do some specific computation and produces output?
A function is a set of statements that take inputs, do some specific computation and produces output. The idea is to put some commonly or repeatedly done task together and make a function so that instead of writing the same code again and again for different inputs, we can call the function .
Can you declare a function in C++?
In C, it is not a good idea to declare a function like fun (). To declare a function that can only be called without any parameter, we should use “void fun (void)”. As a side note, in C++, empty list means function can only be called without any parameter. In C++, both void fun () and void fun (void) are same.
Do changes in a function reflect the actual parameters of the caller?
So any changes made inside functions are not reflected in actual parameters of caller. Pass by Reference Both actual and formal parameters refer to same locations, so any changes made inside the function are actually reflected in actual parameters of caller. Parameters are always passed by value in C.
What are the two types of functions in C?
There are two types of functions in C programming: Library Functions: are the functions which are declared in the C header files such as scanf (), printf (), gets (), puts (), ceil (), floor () etc. User-defined functions: are the functions which are created by the C programmer, so that he/she can use it many times.
What is library function?
Library functions are the inbuilt function in C that are grouped and placed at a common place called the library. Such functions are used to perform some specific operations. For example, printf is a library function used to print on the console. The library functions are created by the designers of compilers.
Why do we call functions multiple times?
A function can be called multiple times to provide reusability and modularity to the C program. In other words, we can say that the collection of functions creates a program. The function is also known as procedure or subroutine in other programming languages.
Can a C function return a value?
A C function may or may not return a value from the function. If you don't have to return any value from the function, use void for the return type.
Predefined Functions
So it turns out you already know what a function is. You have been using it the whole time while studying this tutorial!
Create a Function
To create (often referred to as declare) your own function, specify the name of the function, followed by parentheses () and curly brackets {}:
Call a Function
Declared functions are not executed immediately. They are "saved for later use", and will be executed when they are called.
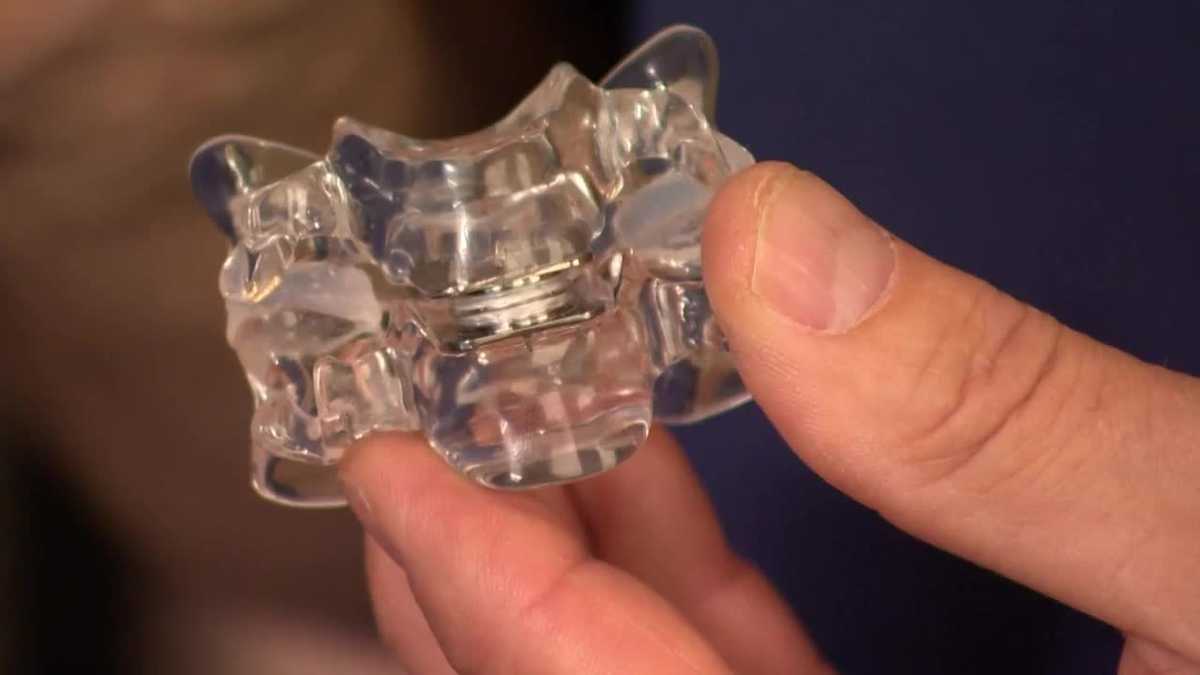