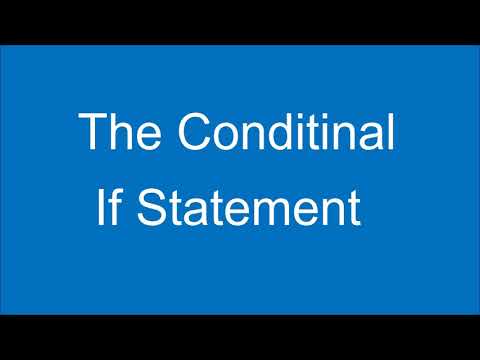
What is if statement in C language?
- In the above program, we have initialized two variables with num1, num2 with value as 1, 2 respectively.
- Then, we have used if with a test-expression to check which number is the smallest and which number is the largest. We have used a relational expression in if construct. ...
- Thus it will print the statement inside the block of If. ...
How to write a while statement in C?
While...End While Statement (Visual Basic)
- Syntax
- Parts. Boolean expression. ...
- Remarks. Use a While...End While structure when you want to repeat a set of statements an indefinite number of times, as long as a condition remains True.
- Exit While. The Exit While statement can provide another way to exit a While loop. ...
- Example 1. ...
- Example 2. ...
- Example 3. ...
How do you terminate statements in C?
Some of the common ways to terminate a program in C are:
- exit
- _Exit ()
- quick_exit
- abort
- at_quick_exit
How to write IF THEN statements?
- Equal (=)
- Unequal (<>)
- Less than (<)
- Greater than (>)
- Less than or equal to (<=)
- Greater than or equal to (>=)
What is IF statement explain with example?
An if statement is a programming conditional statement that, if proved true, performs a function or displays information. Below is a general example of an if statement, not specific to any particular programming language. if (X < 10) { print "Hello John"; }
What is IF statement and write syntax?
Syntax. In both forms of the if statement, the expressions, which can have any value except a structure, are evaluated, including all side effects. In the first form of the syntax, if expression is true (nonzero), statement is executed. If expression is false, statement is ignored.
What is if-else statement in C example?
In C programming language, if-else statement is used to perform the operations based on some specific condition. If the given condition is true, then the code inside if block is executed, otherwise else block code is executed. It specifies an order in which the statements are to be executed.
What is if condition syntax?
The syntax for if statement is as follows: if (condition) instruction; The condition evaluates to either true or false. True is always a non-zero value, and false is a value that contains zero.
Why if statement is used?
In Excel, the IF statement is used in evaluating a logical or mathematical expression and getting the desired output based on the specified criteria. The IF statement works by checking the expression to see whether a condition is met and returns a value based on the output obtained.
How do you end an if statement in C?
C – break statement It is used to come out of the loop instantly. When a break statement is encountered inside a loop, the control directly comes out of loop and the loop gets terminated. It is used with if statement, whenever used inside loop. 2.
What is the if-else statement?
The if/else statement executes a block of code if a specified condition is true. If the condition is false, another block of code can be executed. The if/else statement is a part of JavaScript's "Conditional" Statements, which are used to perform different actions based on different conditions.
Which are logical operators in C?
C Logical OperatorsOperatorMeaning&&Logical AND. True only if all operands are true||Logical OR. True only if either one operand is true!Logical NOT. True only if the operand is 0
What is the difference between if and if-else statement?
The if statement is a decision-making structure that consists of an expression followed by one or more statements. The if else is a decision-making structure in which the if statement can be followed by an optional else statement that executes when the expression is false.
How do you use if or?
When you combine each one of them with an IF statement, they read like this:AND – =IF(AND(Something is True, Something else is True), Value if True, Value if False)OR – =IF(OR(Something is True, Something else is True), Value if True, Value if False)NOT – =IF(NOT(Something is True), Value if True, Value if False)
What is the purpose of the if statement?
The ability to control the flow of your program, letting it make decisions on what code to execute, is valuable to the programmer. The if statement allows you to control if a program enters a section of code or not based on whether a given condition is true or false. One of the important functions of the if statement is ...
How to have more than one statement execute after an if statement?
To have more than one statement execute after an if statement that evaluates to true, use braces, like we did with the body of the main function. Anything inside braces is called a compound statement, or a block. When using if statements, the code that depends on the if statement is called the "body" of the if statement. For example:
What happens if the else statement is true?
If the if statement was true the else statement will not be checked. It is possible to use numerous else if statements to ensure that only one block of code is executed. Let's look at a simple program for you to try out on your own. 1.
What does "else" mean in if?
The "else" statement effectively says that whatever code after it (whether a single line or code between brackets) is executed if the if statement is FALSE.
When to use "other" in a conditional statement?
Another use of else is when there are multiple conditional statements that may all evaluate to true, yet you want only one if statement's body to execute. You can use an "else if" statement following an if statement and its body; that way, if the first statement is true, the "else if" will be ignored, but if the if statement is false, ...
What is the difference between boolean and not?
The boolean operators function in a similar way to the comparison operators: each returns 0 if evaluates to FALSE or 1 if it evaluates to TRUE. NOT: The NOT operator accepts one input. If that input is TRUE, it returns FALSE, and if that input is FALSE, it returns TRUE.
What does "if" mean in a statement?
The if statement evaluates the test expression inside the parenthesis (). If the test expression is evaluated to true, statements inside the body of if are executed. If the test expression is evaluated to false, statements inside the body of if are not executed.
How does if.else work?
How if...else statement works? 1 statements inside the body of else are executed 2 statements inside the body of if are skipped from execution.
What happens to statements inside the body of if?
statements inside the body of if are executed. statements inside the body of else are skipped from execution. If the test expression is evaluated to false, statements inside the body of else are executed. statements inside the body of if are skipped from execution.
What is C comparison?
C is very light and close to the hardware it's running on . With hardware it's easy to check if something is 0 or false, but anything else is much more difficult.
What is conditional flow?
Conditional code flow is the ability to change the way a piece of code behaves based on certain conditions. In such situations you can use if statements. The if statement is also known as a decision making statement, as it makes a decision on the basis of a given condition or expression. The block of code inside the if statement is executed is ...
Can you use true and false in C?
That's okay because true and false aren' t being used like in the first example. In C, like in other programming languages, you can use statements that evaluate to true or false rather than using the boolean values true or false directly. Also notice the condition in the parenthesis of the if statement: n == 3.
What is the shorthand of if else?
Short Hand If...Else (Ternary Operator) There is also a short-hand if else, which is known as the ternary operator because it consists of three operands. It can be used to replace multiple lines of code with a single line. It is often used to replace simple if else statements:
When to use "other" in Java?
Use else to specify a block of code to be executed, if the same condition is false. Use else if to specify a new condition to test, if the first condition is false. Use switch to specify many alternative blocks of code to be executed.
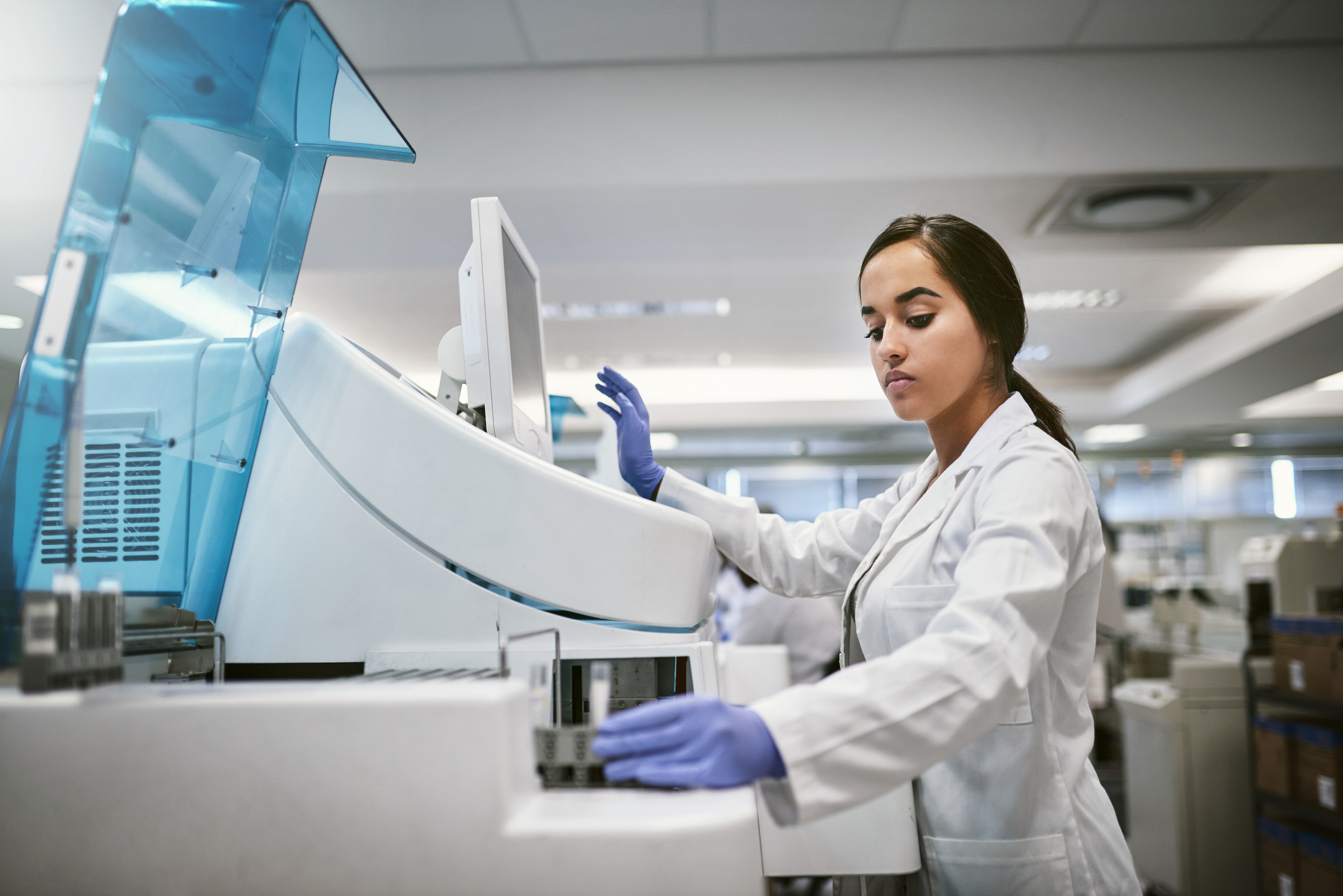
Explanation of Syntax
Different Types of If Statement
- These are different types of If Statement. Let us explain in-depth with syntax. 1. If-else statement 2. If-elseif-else statement
Conclusion
- C is a programming language where there are lots of concepts that one needs to study. Suppose the statement is one of those. These operators basically execute the code to check whether the expression value is true or not. Based on the expression evaluation, it executes the code. And if the statement is widely used in anyprogramming languageto vario...
Recommended Articles
- This is a guide to If Statement in C. Here we discuss the different types of If Statement with the appropriate explanation of the Syntax along with sample code. You may also have a look at the following articles to learn more – 1. C# if Statement 2. If-else Statement in C 3. If Else Statement in Python 4. If Statement in Python