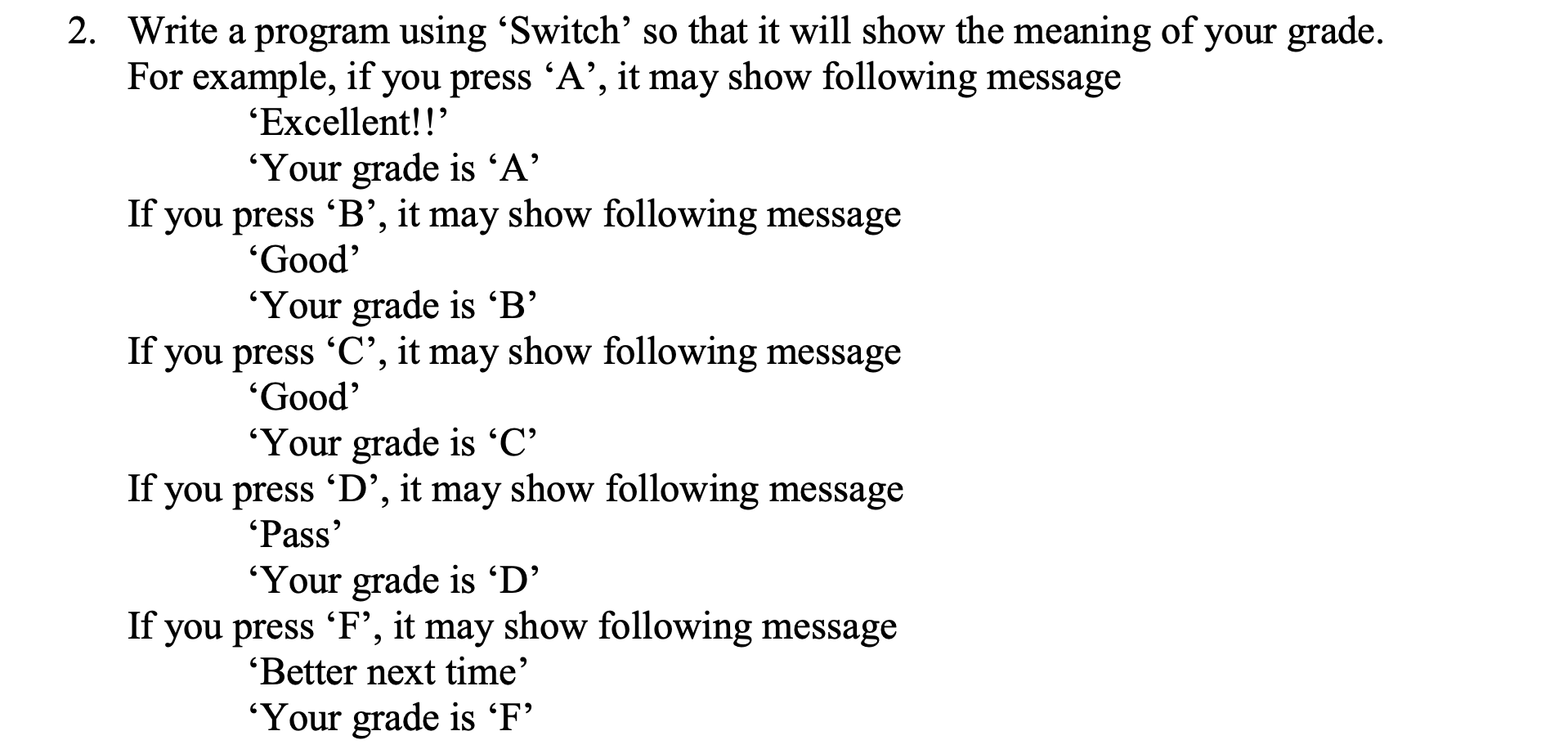
Explanation:
- It is known that “std” (abbreviation for the standard) is a namespace whose members are used in the program.
- So the members of the “std” namespace are cout, cin, endl, etc.
- This namespace is present in the iostream.h header file.
- Below is the code snippet in C++ showing content written inside iostream.h:
What is the relationship between iostream and namespace std?
Feb 16, 2018 · The using namespace statement just means that in the scope it is present, make all the things under the std namespace available without having to prefix std:: before each of them. While this practice is okay for short example code or trivial programs, pulling in the entire std namespace into the global namespace is not a good habit as it defeats the purpose of …
Why using namespace std is necessary here?
Feb 24, 2021 · It is known that “std” (abbreviation for the standard) is a namespace whose members are used in the program. So the members of the “std” namespace are cout, cin, endl, etc. This namespace is present in the iostream.h header file. Below is the code snippet in C++ showing content written inside iostream.h: C++ namespace std { ostream cout;
What STD is connected to needle use?
May 19, 2020 · What is the meaning of using namespace std? using namespace std means that you are going to use classes or functions (if any) from "std" namespace, so you don't have to explicitly call the namespace to access them. example using "using namespace std" Click to see full answer. Likewise, why do you use using namespace std?
How to include the system namespace?
May 06, 2021 · std is an abbreviation of "standard". std is the "standard namespace". cout, cin and a lot of other functions are defined within it. The reason for using this: When you don't use the std namespace, the compiler will try to call cout or cin as if they aren't defined in a namespace (like most functions in your codes).
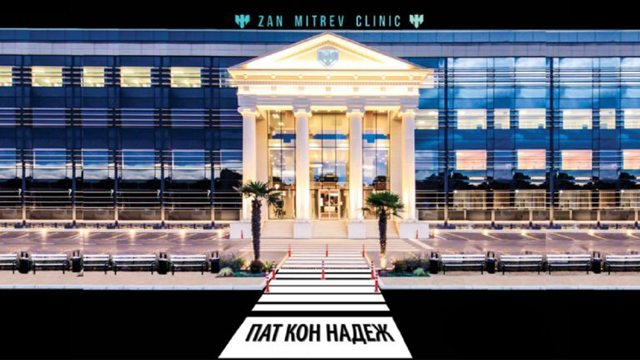
What is the purpose of using namespace std in C++?
Is it good to use using namespace std?
Who created C++?
What can I use instead of namespace std?
What is namespace?
It is a container for a set of identifi e rs. It provide a level of direction to specific identifiers, thus making it possible to distinguish between identifiers with the same exact name. For example, a surname could be thought of as a namespace that makes it possible to distinguish people who have the same given name.
Why we need namespaces?
Assume there is two libraries named A and B, both have get_data function in it. You included these two libraries in your code. Now you call the get_data function, then compiler won’t know which one to call, and you’ll get an error. Now how we can fix this issue.
Why we mention this in our code?
Now suppose in the above example, if you are going to use bunch of code of A library then its going to boring to add prefix every time while calling the functions of A library. Then what is the solution of this problem
What does "using namespace std" mean?
using namespace std means that you are going to use classes or functions (if any) from "std" namespace, so you don't have to explicitly call the namespace to access them. example using "using namespace std". Click to see full answer.
What is a namespace in C++?
Namespace is a feature added in C++ and not present in C. A namespace is a declarative region that provides a scope to the identifiers (names of the types, function, variables etc) inside it. Multiple namespace blocks with the same name are allowed. All declarations within those blocks are declared in the named scope.
What is a namespace in coding?
A namespace is a declarative region that provides a scope to the identifiers (the names of types, functions, variables, etc) inside it. Namespaces are used to organize code into logical groups and to prevent name collisions that can occur especially when your code base includes multiple libraries.
Can a namespace be nested?
Namespaces may be nested. An ordinary nested namespace has unqualified access to its parent's members, but the parent members do not have unqualified access to the nested namespace (unless it is declared as inline), as shown in the following example: C++.
Why do namespaces need to be unique?
Namespace names need to be unique, which means that often they should not be too short. If the length of a name makes code difficult to read, or is tedious to type in a header file where using directives can't be used, then you can make a namespace alias which serves as an abbreviation for the actual name. For example:
What happens if an identifier is not declared in an explicit namespace?
If an identifier is not declared in an explicit namespace, it is part of the implicit global namespace. In general, try to avoid making declarations at global scope when possible, except for the entry point main Function, which is required to be in the global namespace. To explicitly qualify a global identifier, use the scope resolution operator with no name, as in ::SomeFunction (x);. This will differentiate the identifier from anything with the same name in any other namespace, and it will also help to make your code easier for others to understand.
What is an inline namespace?
In contrast to an ordinary nested namespace, members of an inline namespace are treated as members of the parent namespace . This characteristic enables argument dependent lookup on overloaded functions to work on functions that have overloads in a parent and a nested inline namespace. It also enables you to declare a specialization in a parent namespace for a template that is declared in the inline namespace. The following example shows how external code binds to the inline namespace by default:
What is a using directive?
The using directive allows all the names in a namespace to be used without the namespace-name as an explicit qualifier. Use a using directive in an implementation file (i.e. *.cpp) if you are using several different identifiers in a namespace; if you are just using one or two identifiers, then consider a using declaration to only bring those identifiers into scope and not all the identifiers in the namespace. If a local variable has the same name as a namespace variable, the namespace variable is hidden. It is an error to have a namespace variable with the same name as a global variable.
Defining a Namespace
A namespace definition begins with the keyword namespace followed by the namespace name as follows −
The using directive
You can also avoid prepending of namespaces with the using namespace directive. This directive tells the compiler that the subsequent code is making use of names in the specified namespace. The namespace is thus implied for the following code −
Discontiguous Namespaces
A namespace can be defined in several parts and so a namespace is made up of the sum of its separately defined parts. The separate parts of a namespace can be spread over multiple files.
Nested Namespaces
Namespaces can be nested where you can define one namespace inside another name space as follows −
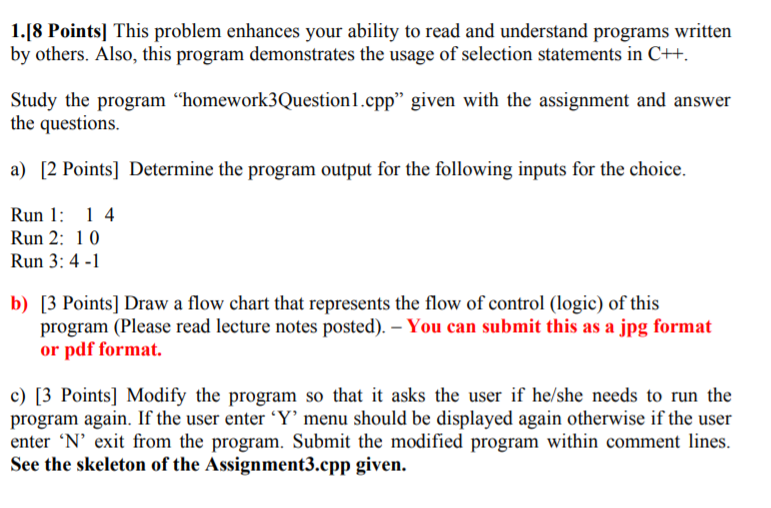
What Is namespace?
Why We Need namespaces?
- Assume there is two libraries named A and B, both have get_data function in it. You included these two libraries in your code. Now you call the get_data function, then compiler won’t know which one to call, and you’ll get an error. Now how we can fix this issue. In C lang we can fix by adding a prefix to start of every function like A_get_data and B_get_data,but is painful. The soluti…
Why We Mention This in Our Code?
- Now suppose in the above example, if you are going to use bunch of code of A library then its going to boring to add prefix every time while calling the functions of A library. Then what is the solution of this problem here is the solution you can add “using namespace A”at the top of your file, and then just call get_data without using A prefix for the rest of code.
Is ‘Using Namespace STD’ Is Necessary to Mention?
- The answer is big NO. The std namespaceis special, The built in C++ library routines are kept in the standard namespace. That includes stuff like cout, cin, string, vector, map, etc. Because these tools are used so commonly, it’s popular to add “using namespace std” at the top of your source code so that you won’t have to type the std:: prefix cons...
Drawbacks of ‘Using namespace’
- Professional programmers considered that it is bad manners to put “using namespace” declarations in header files. It forces all includers of that header file to use that namespace, which might result in naming ambiguities that are hard to fix. This practice is called namespace pollution. Instead, always use the fully prefixed names in header files (std::string not string) and …