
What does the Java Operator New do?
The new operator in Java. The new operator is used in Java to create new objects. It can also be used to create an array object. Let us first see the steps when creating an object from a class −. Declaration − A variable declaration with a variable name with an object type. Instantiation − The 'new' keyword is used to create the object.
How to put new line in Java?
There are many ways to print new line in string been illustrated as below:
- Using System.lineSeparator () method
- Using platform-dependent newline character
- Using System.getProperty () method
- Using %n newline character
- Using System.out.println () method
Is Java a new COBOL?
Java will not become the new COBOL and neither will anything else. COBOL belongs to an era when there was no Network and a centralised mainframe only needed one or two high level languages to program it. Those days are gone and COBOL is hanging on currently purely because of its legacy applications. Even these are being slowly replaced. The ...
Does a new operator execute construvtor?
The constructor function is a regular JavaScript function that contains a recipe to create a new object. When we invoke it using the new operator it creates a new instance of the object and returns it. By Convention, we capitalize the first letter of the constructor function name.
See more
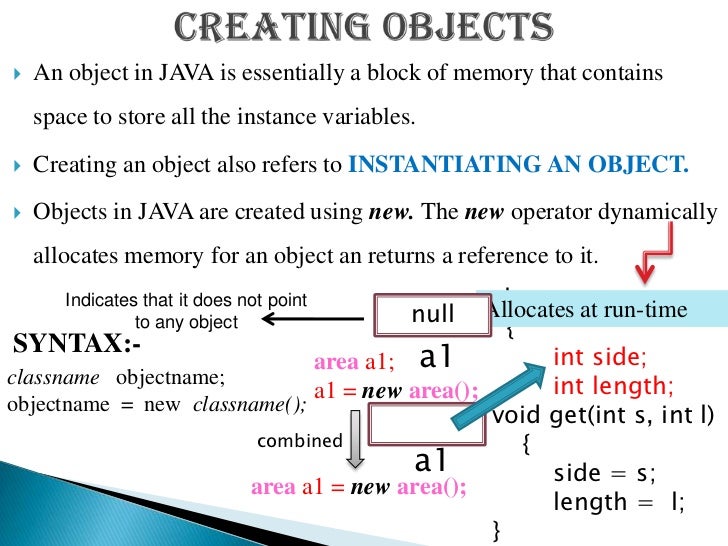
Example
public class Puppy { public Puppy(String name) { // This constructor has one parameter, name. System.out.println("Passed Name is : " + name ); } public static void main(String []args) { // Following statement would create an object myPuppy Puppy myPuppy = new Puppy( "jackie" ); } }
Output
Now, let us see an example to create an array using the new operator −
How to tell the difference between a constructor and a method call?
Constructors only initialise pre-existing objects. The way to tell the difference between a constructor and a method call is the new keyword. e.g. you can have a method called Object in the class Object but this might not create anything. When you have sub-classes this is even more confusing.
What is the point of the new keyword?
The point of the new keyword is to make it clear when a new object is created. BTW You can have a factory method which returns a new object as you suggest, however making it explicit may be considered clearer as to what it is actually doing. This works like it does to avoid name collisions.
Can you have a factory method that returns a new object?
BTW You can have a factory method which returns a new object as you suggest, however making it explicit may be considered clearer as to what it is actually doing.
How does a compiler use Lex?
Be a Compiler: Compiler in our systems uses lex tool to match the greatest match when generating tokens. This creates a bit of a problem if overlooked. For example, consider the statement a=b+++c;, to many of the readers this might seem to create compiler error. But this statement is absolutely correct as the token created by lex are a, =, b, ++, +, c. Therefore this statement has a similar effect of first assigning b+c to a and then incrementing b. Similarly, a=b+++++c; would generate error as tokens generated are a, =, b, ++, ++, +, c. which is actually an error as there is no operand after second unary operand.
What is the rule for associativity and precedence?
There is a golden rule to follow in these situations. If the operators have different precedence, solve the higher precedence first. If they have same precedence, solve according to associativity, that is either from right to left or from left to right. Explanation of below program is well written in comments withing the program itself.
What is the meaning of :Unary minus?
They are used to increment, decrement or negate a value. – :Unary minus, used for negat ing the values. + :Unary plus, indicates positive value (numbers are positive without this, however). It performs an automatic conversion to int when the type of its operand is byte, char, or short.
What is a ternary operator?
Ternary operator : Ternary operator is a shorthand version of if-else statement. It has three operands and hence the name ternary. General format is-
What is the golden rule for solving associativity?
If the operators have different precedence, solve the higher precedence first. If they have same precedence, solve according to associativity, that is either from right to left or from left to right.
What is an instance of operator?
instance of operator : Instance of operator is used for type checking. It can be used to test if an object is an instance of a class, a subclass or an interface. General format-
What is shift operator?
Shift Operators : These operators are used to shift the bits of a number left or right thereby multiplying or dividing the number by two respectively. They can be used when we have to multiply or divide a number by two. General format-
Example 1
Let's see a simple example to create an object using new keyword and invoking the method using the corresponding object reference.
Example 2
Let's see a simple example to create an object using new keyword and invoking the constructor using the corresponding object reference.
Example 3
Here, we create an object using new keyword and invoke the parameterized constructor.
What is assignment operator?
Assignment operators are used to assign values to variables.
What is an operator used for?
Operators are used to perform operations on variables and values.
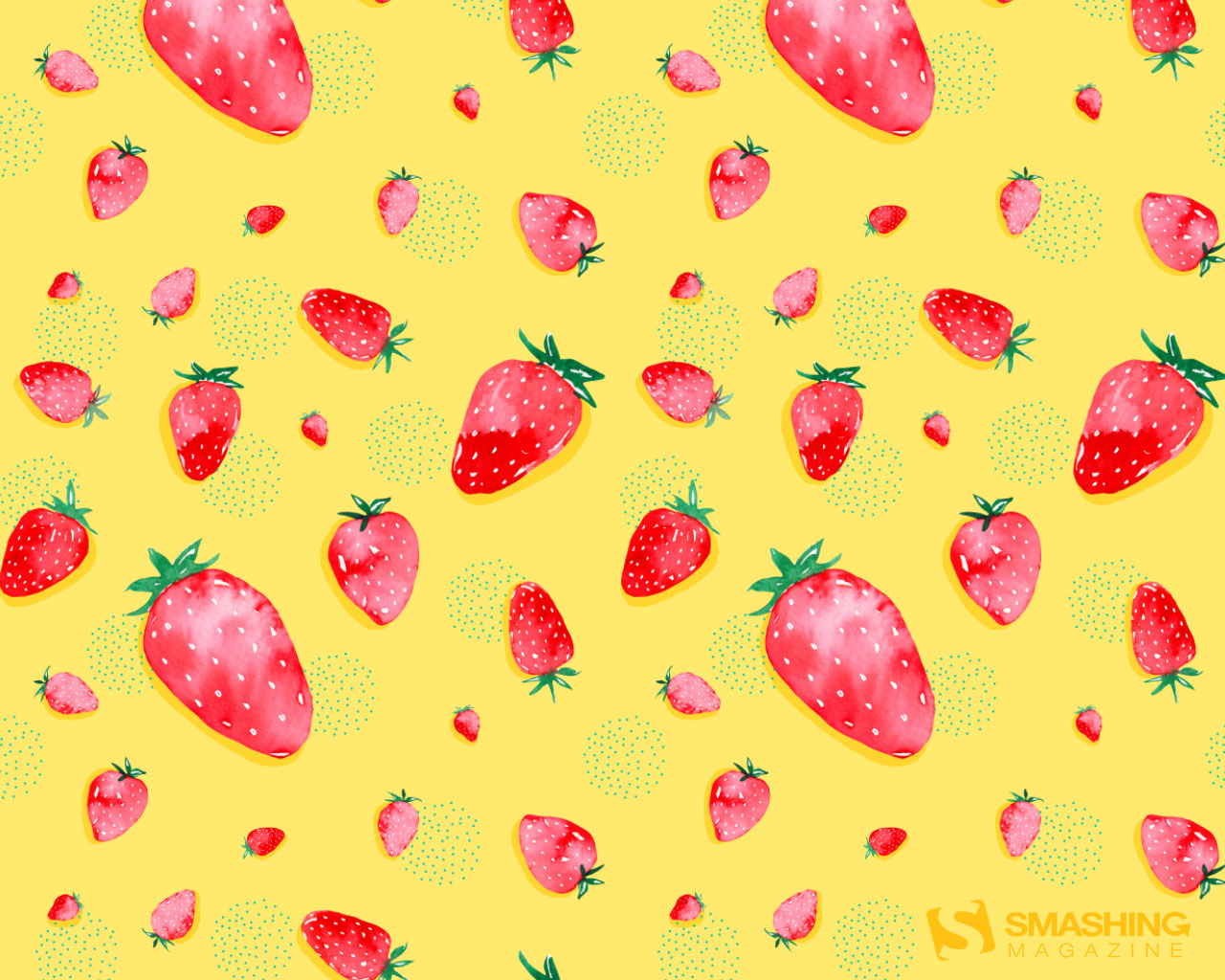