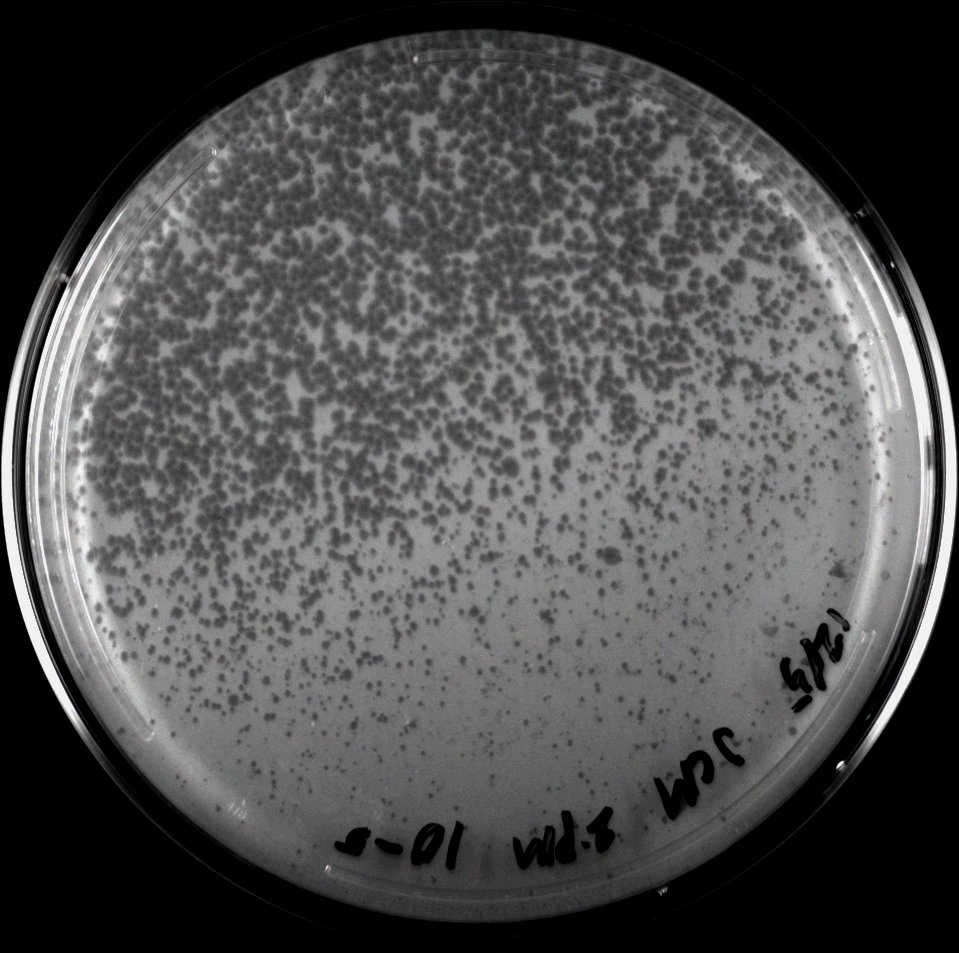
Quick Annotation Overview
- @ApiOperation. The @ApiOperation is used to declare a single operation within an API resource. ...
- @ApiResponses, @ApiResponse. It's a common practice to return errors (or other success messages) using HTTP status codes. ...
- @Authorization, @AuthorizationScope. ...
- @ApiImplicitParam, @ApiImplicitParams
What is the use of @API annotations in Java?
The @API annotations as per the documentation states “The annotation @Api is used to configure the whole API, and apply to all public methods of a class unless overridden by @APIMethod”. Note the words unless overridden. Often you find that you casually go ahead and mark a class with @API.
How do I use @API authorization annotations?
These annotations are used as input to @Api and @ApiOperation only, and not directly on the resources and operations. Once you've declared and configured which authorization schemes you support in your API, you can use this annotation to note which authorization scheme is required on a resource or a specific operation.
What is the use of @apioperation?
The @ApiOperation is used to declare a single operation within an API resource. An operation is considered a unique combination of a path and a HTTP method. Only methods that are annotated with @ApiOperation will be scanned and added the API Declaration.
Why is the @API annotation deprecated in Swagger?
Unfortunately, this solution does not work, see github.com/swagger-api/swagger-core/issues/1476. The reason why it's deprecated is that previous Swagger versions (1.x) used the @Api description annotation to group operations. In the Swagger 2.0 specification, the notion of tags was created and made a more flexible grouping mechanism.
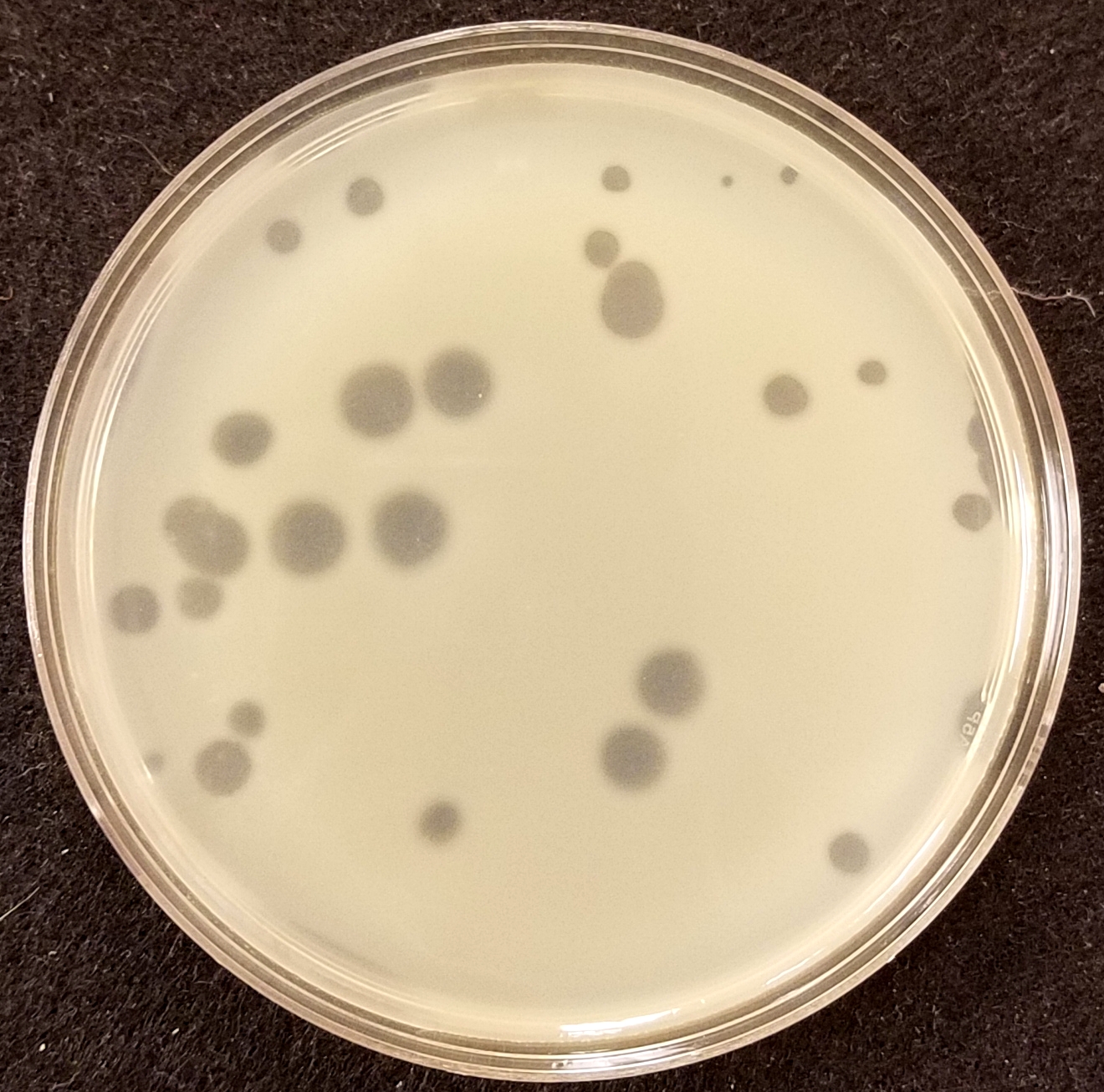
What is annotations in API?
The Annotations API is an extension to the Europeana REST API which allows you to create, retrieve and manage annotations on Europeana objects. Annotations are user-contributed or system-generated enhancements, additions or corrections to (or a selection of) metadata or media.
What is the use of API annotation in spring boot?
The @API annotations as per the documentation states “The annotation @Api is used to configure the whole API, and apply to all public methods of a class unless overridden by @APIMethod”.
What are the uses of annotation?
Annotations have a number of uses, among them: Information for the compiler — Annotations can be used by the compiler to detect errors or suppress warnings. Compile-time and deployment-time processing — Software tools can process annotation information to generate code, XML files, and so forth.
What are annotations used in REST API?
Annotations are like meta-tags that you can add to the code and apply to package declarations, type declarations, constructors, methods, fields, parameters, and variables.
Where @autowired can be used?
You can use @Autowired annotation on setter methods to get rid of the
What is @controller and @RestController?
@Controller is used to mark classes as Spring MVC Controller. @RestController annotation is a special controller used in RESTful Web services, and it's the combination of @Controller and @ResponseBody annotation. It is a specialized version of @Component annotation.
What are 3 types of annotations?
Types of AnnotationsDescriptive.Evaluative.Informative.Combination.
What is an example of annotation?
But the term can be used in a more general way to refer to a note added to any text. For example, a note that you scribble in the margin of your textbook is an annotation, as is an explanatory comment that you add to a list of tasks at work. Something that has had such notes added to it can be described as annotated.
Why do we need annotations in Java?
Java annotations are metadata (data about data) for our program source code. They provide additional information about the program to the compiler but are not part of the program itself. These annotations do not affect the execution of the compiled program.
What is @RestController in Spring boot?
Spring RestController annotation is a convenience annotation that is itself annotated with @Controller and @ResponseBody . This annotation is applied to a class to mark it as a request handler. Spring RestController annotation is used to create RESTful web services using Spring MVC.
What are JPA annotations?
JPA annotations are used in mapping java objects to the database tables, columns etc. Hibernate is the most popular implement of JPA specification and provides some additional annotations. Today we will look into JPA annotations as well as Hibernate annotations with brief code snippets.
What is RESTful API?
RESTful API is an interface that two computer systems use to exchange information securely over the internet. Most business applications have to communicate with other internal and third-party applications to perform various tasks.
What is @required annotation in Spring?
The @Required annotation applies to bean property setter methods and it indicates that the affected bean property must be populated in XML configuration file at configuration time. Otherwise, the container throws a BeanInitializationException exception.
What is @GetMapping annotation in spring boot?
@GetMapping annotation maps HTTP GET requests onto specific handler methods. It is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod. GET) .
What is @data annotation in spring boot?
@Data is a convenient shortcut annotation that bundles the features of @ToString , @EqualsAndHashCode , @Getter / @Setter and @RequiredArgsConstructor together: In other words, @Data generates all the boilerplate that is normally associated with simple POJOs (Plain Old Java Objects) and beans: getters for all fields, ...
What is the use of @ApiResponse?
Annotation Type ApiResponse. Describes a possible response of an operation. This can be used to describe possible success and error codes from your REST API call.
Annotating Text
Imagine a use case where one wants to add some metadata to a chunk of text, where various parts of the text have some semantics that we want to express.
Alternate subtrees
For the examples above, the purpose of the annotator has been to express the structure of the original HTML document, as well as adding some semantics to the tree. The HTML structure is fairly unambiguous (let's assume valid HTML for now). However, there are many other use cases where the source text allows for multiple interpretations, i.e.
Manipulating a Span Tree
The previous section focused mainly on building a span tree over an input string. In many cases though, like when using the docproc framework, a document processor reads a span tree created by some previous process, manipulates it, and passes it on.
Annotation Inheritance
Annotation types can inherit from each other. This is particularly useful when given e.g. a document processor (along with its configuration of annotation types and document types) from some external entity, and one wants to extend these annotation types with some additional information. Review the below example:
Where can I read more about storing definitions in SwaggerHub with Gradle or Maven?
SwaggerHub offers two core plugins for automating the export of generated OAS definitions into the platform.
We use the Swagger Codegen project for generating server stubs, is there a reason we should be using SwaggerHub to do this?
SwaggerHub was created by the same team behind the Swagger Codegen project. In fact, the code generation functionality in SwaggerHub runs on the contributions of the open source project.
What languages are supported for OAS 3.0 code generation in SwaggerHub?
We are continuing to roll out support for new languages in SwaggerHub. Here is the latest update on supported languages for OAS 3.0:
What happens to the specification when there are changes to the API, does it automatically update the existing spec document in SwaggerHub?
By connecting source code to a platform like SwaggerHub – it's possible to keep an external specification in line with the generated version that is part of the build process. Beyond this SwaggerHub has a robust versioning system, allowing multiple teams, projects or changes to be intelligently catalogued and stored in a single source of truth.
Regarding API Gateway deployments, do you have the ability within the definition to add in validation directly alongside being added to the spec?
This functionality typically doesn’t fall into the definition itself – we would hand the OAS that is generated off to a file that would execute a series of requests, and validate them against the defined responses.
Is there a way to convert SwaggerHub comments to JIRA tasks?
At this time we don’t have a direct integration to JIRA. The SwaggerHub team is constantly adding to the list of supported tools and platforms as we take on feedback from users and organizations.

Annotating Text
- Using Annotations as Simple Labels
Imagine a use case where one wants to add some metadata to a chunk of text, where various parts of the text have some semantics that we want to express. This can be done by marking up the text with spans - where a span is identified by a start character index, and a length, and grou… - Annotation Trees
The annotated spans shown above might be fine for the simple cases where one wants to just annotate some text. However, let's imagine that one wants to not only identify markup from text, but also create a structure over the markup. In such a case, we can build a tree of spans using S…
Alternate Subtrees
- For the examples above, the purpose of the annotator has been to express the structure of the original HTML document, as well as adding some semantics to the tree. The HTML structure is fairly unambiguous (let's assume valid HTML for now). However, there are many other use cases where the source text allows for multiple interpretations, i.e. where there is not one unambiguou…
Manipulating A Span Tree
- The previous section focused mainly on building a span tree over an input string. In many cases though, like when using the docproc framework, a document processor reads a span tree created by some previous process, manipulates it, and passes it on.
Annotation Inheritance
- Annotation types can inherit from each other. This is particularly useful when given e.g. a document processor (along with its configuration of annotation types and document types) from some external entity, and one wants to extend these annotation types with some additional information. Review the below example: This annotation type, person, comes...