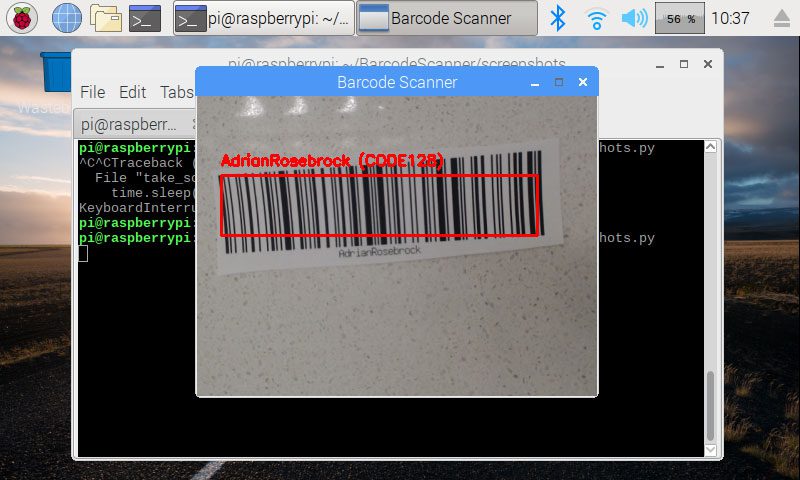
How to implement passwordencoder that uses the bcrypt strong hashing function?
Implementation of PasswordEncoder that uses the BCrypt strong hashing function. Clients can optionally supply a "version" ($2a, $2b, $2y) and a "strength" (a.k.a. log rounds in BCrypt) and a SecureRandom instance. The larger the strength parameter the more work will have to be done (exponentially) to hash the passwords. The default value is 10.
What is the difference between bcrypt and other password encoders?
Older implementations – such as SHAPasswordEncoder – would require the client to pass in a salt value when encoding the password. BCrypt, however, will internally generate a random salt instead.
What is bcrypt and how does it work?
BCrypt, however, will internally generate a random salt instead. This is important to understand because it means that each call will have a different result, and so we need to only encode the password once. To make this random salt generation work, BCrypt will store the salt inside the hash value itself.
What is the length of a bcrypt password?
Also, be aware that the BCrypt algorithm generates a String of length 60, so we need to make sure that the password will be stored in a column that can accommodate it. A common mistake is to create a column of a different length and then get an Invalid Username or Password error at authentication time. 3. Encode the Password on Registration

How do I use BCryptPasswordEncoder in spring boot?
Bootstrap: @Autowired private BCryptPasswordEncoder bCryptPasswordEncoder; @GetMapping("/test") public void fillDatabse() { String encodedPw=bCryptPasswordEncoder. encode("test"); Password p = new Password(encodedPw);
What is BCryptPasswordEncoder in Java?
BCrypt is a one-way salted hash function based on the Blowfish cipher. It provides several enhancements over plain text passwords (unfortunately this still happens quite often) and traditional hashing algorithms (md5).
Can we decode BCryptPasswordEncoder?
BCryptPasswordEncoder is a single-way password encoder. The one-way encoding algorithm is used to encrypt a password. There's no way to decrypt the password. Alternatively, the one-way password encoder returns the same encrypted string if you call the encoding algorithm with the same password.
What is spring boot salt?
A salt is a sequence of randomly generated bytes that is hashed along with the password. The salt is stored in the storage and doesn't need to be protected. Whenever the user tries to authenticate, the user's password is hashed with the saved salt and the result should match the stored password.
Is Bcryptpasswordencoder thread safe?
First, it's not documented as thread-safe, so for all intents and purposes, it's not. And, on further investigation, it's definitely not: It turns out that, while there are some instance fields, there is no instance of BCrypt exposed; it tries to do everything through static methods. It may not be thread safe.
How does bcrypt salt work?
The bcrypt utility itself does not appear to maintain a list of salts. Rather, salts are generated randomly and appended to the output of the function so that they are remembered later on (according to the Java implementation of bcrypt ). Put another way, the "hash" generated by bcrypt is not just the hash.
Can you reverse BCrypt?
You can't decrypt the hash, because - as you said - hash functions can't be reversed. You should still change your passwords.
Is BCrypt secure?
The result of bcrypt achieves core properties of a secure password function as defined by its designers: It's preimage resistant. The salt space is large enough to mitigate precomputation attacks, such as rainbow tables.
Is BCrypt a hash or encryption?
password-hashing functionbcrypt is a password-hashing function designed by Niels Provos and David Mazières, based on the Blowfish cipher and presented at USENIX in 1999.
What is the use of salting?
Salting refers to adding random data to a hash function to obtain a unique output which refers to the hash. Even when the same input is used, it is possible to obtain different and unique hashes. These hashes aim to strengthen security, protect against dictionary attacks, brute-force attacks, and several others.
How are passwords stored in databases?
The password entered by user is concatenated with a random generated salt as well as a static salt. The concatenated string is passed as the input of hashing function. The result obtained is stored in database. Dynamic salt is required to be stored in the database since it is different for different users.
What is Spring vault?
Spring Vault provides familiar Spring abstractions and client-side support for accessing, storing and revoking secrets. It offers both low-level and high-level abstractions for interacting with Vault, freeing the user from infrastructural concerns.
What is BCrypt in spring?
Class BCrypt This password hashing system tries to thwart off-line password cracking using a computationally-intensive hashing algorithm, based on Bruce Schneier's Blowfish cipher. The work factor of the algorithm is parameterised, so it can be increased as computers get faster. Usage is really simple.
What is Spring Security in Java?
Spring Security is a powerful and highly customizable authentication and access-control framework. It is the de-facto standard for securing Spring-based applications. Spring Security is a framework that focuses on providing both authentication and authorization to Java applications.
How do I use WebSecurityConfigurerAdapter?
WebSecurityConfigurerAdapterRequire the user to be authenticated prior to accessing any URL within our application.Create a user with the username “user”, password “password”, and role of “ROLE_USER”Enables HTTP Basic and Form based authentication.More items...•
What is Jasypt spring boot starter?
Jasypt (Java Simplified Encryption) Spring Boot provides utilities for encrypting property sources in Boot applications.