
Request bodies are typically used with “create” and “update” operations (POST, PUT, PATCH). For example, when creating a resource using POST or PUT, the request body usually contains the representation of the resource to be created. OpenAPI 3.0 provides the requestBody keyword to describe request bodies.
What is the use of @requestbody and @responsebody annotations?
@RequestBody and @ResponseBody annotations are used to bind the HTTP request/response body with a domain object in method parameter or return type. Behind the scenes, these annotation uses HTTP Message converters to convert the body of HTTP request/response to domain objects.
What is @requestbody in Spring Boot?
import org.springframework.web.bind.annotation.*; In the preceding controller class, the @RequestBody annotation is specified on the registerUserCredential () method. This annotation informs Spring to deserialize an incoming request body to the User domain object.
What does it mean 'HTTP request body'?
What Does it mean " HTTP request body".? HTTP Body Data is the data bytes transmitted in an HTTP transaction message immediately following the headers if there is any (in the case of HTTP/0.9 no headers are transmitted). Most HTTP requests are GET requests without bodies.
What is the difference between @requestbody and @responsebody in Salesforce?
By using @RequestBody annotation you will get your values mapped with the model you created in your system for handling any specific call. While by using @ResponseBody you can send anything back to the place from where the request was generated. Both things will be mapped easily without writing any custom parser etc.
What parameter is the body of web request assigned to?
What is @request body in Spring?
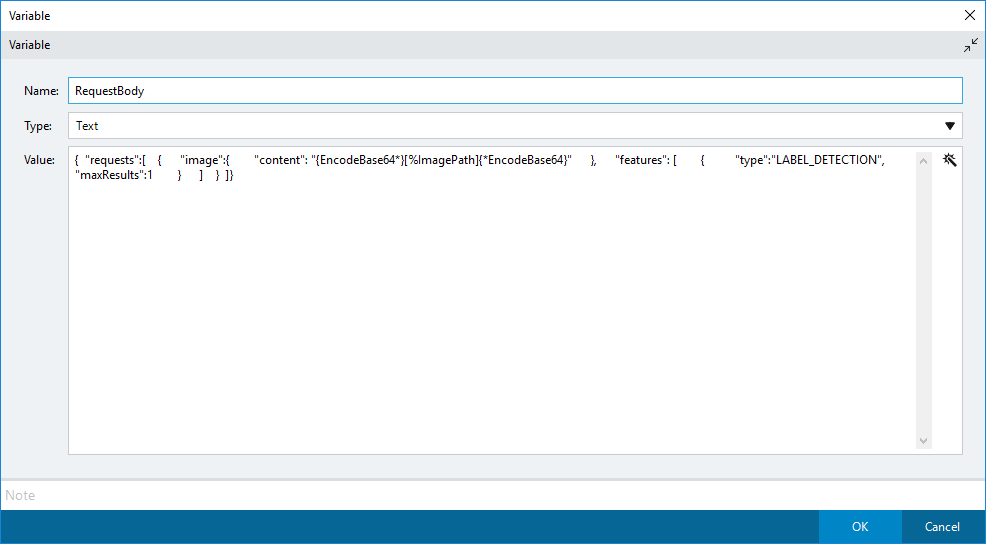
What is the use of @RequestBody annotation in Spring boot?
The @RequestBody annotation allows us to retrieve the request's body. We can then return it as a String or deserialize it into a Plain Old Java Object (POJO). Spring has built-in mechanisms for deserializing JSON and XML objects into POJOs, which makes this task a lot easier as well.
What is @RequestBody in rest API?
A request body is data sent by the client to your API. A response body is the data your API sends to the client. Your API almost always has to send a response body. But clients don't necessarily need to send request bodies all the time.
What is @RequestBody in Spring MVC?
The @RequestBody annotation is applicable to handler methods of Spring controllers. This annotation indicates that Spring should deserialize a request body into an object. This object is passed as a handler method parameter.
Is @RequestBody mandatory?
requestBody consists of the content object, an optional Markdown-formatted description , and an optional required flag ( false by default). content lists the media types consumed by the operation (such as application/json ) and specifies the schema for each media type. Request bodies are optional by default.
What is @RequestBody and @ResponseBody in Spring boot?
@RequestBody and @ResponseBody annotations are used to bind the HTTP request/response body with a domain object in method parameter or return type. Behind the scenes, these annotation uses HTTP Message converters to convert the body of HTTP request/response to domain objects.
Why do we use @autowired annotation?
The @Autowired annotation provides more fine-grained control over where and how autowiring should be accomplished. The @Autowired annotation can be used to autowire bean on the setter method just like @Required annotation, constructor, a property or methods with arbitrary names and/or multiple arguments.
What is @RequestBody and @ResponseBody?
By using @RequestBody annotation you will get your values mapped with the model you created in your system for handling any specific call. While by using @ResponseBody you can send anything back to the place from where the request was generated. Both things will be mapped easily without writing any custom parser etc.
What is @configuration in Spring boot?
Spring @Configuration annotation is part of the spring core framework. Spring Configuration annotation indicates that the class has @Bean definition methods. So Spring container can process the class and generate Spring Beans to be used in the application.
Is @RestController a stereotype?
The @RestController annotation is a specialized version of the @Controller that does more than just mark a component as being used for REST requests; thus it is not considered a stereotype annotation, and was declared in a different package: org. springframework.
What is RequestBody in swagger?
In Swagger terms, the request body is called a body parameter. There can be only one body parameter, although the operation may have other parameters (path, query, header).
Can we send request body?
Yes, you can send a request body with GET but it should not have any meaning.
What is the use of @RestController in Spring boot?
Spring RestController annotation is used to create RESTful web services using Spring MVC. Spring RestController takes care of mapping request data to the defined request handler method. Once response body is generated from the handler method, it converts it to JSON or XML response.
What is @RequestBody and @ResponseBody?
By using @RequestBody annotation you will get your values mapped with the model you created in your system for handling any specific call. While by using @ResponseBody you can send anything back to the place from where the request was generated. Both things will be mapped easily without writing any custom parser etc.
What is GET VS POST?
POST. HTTP POST requests supply additional data from the client (browser) to the server in the message body. In contrast, GET requests include all required data in the URL.
What is the difference between POST and put?
The difference between POST and PUT is that PUT requests are idempotent. That is, calling the same PUT request multiple times will always produce the same result. In contrast, calling a POST request repeatedly have side effects of creating the same resource multiple times.
What is @configuration in Spring boot?
Spring @Configuration annotation is part of the spring core framework. Spring Configuration annotation indicates that the class has @Bean definition methods. So Spring container can process the class and generate Spring Beans to be used in the application.
Spring's RequestBody and ResponseBody Annotations | Baeldung
Simply put, the @RequestBody annotation maps the HttpRequest body to a transfer or domain object, enabling automatic deserialization of the inbound HttpRequest body onto a Java object. First, let's have a look at a Spring controller method: @PostMapping("/request") public ResponseEntity postController( @RequestBody LoginForm loginForm) { exampleService.fakeAuthenticate(loginForm); return ...
Spring @RequestBody - binding method parameters to request ... - ZetCode
Spring @RequestBody example. The application binds request body parameters of a form POST and JSON post request to mapped method arguments. pom.xml src ├───main │ ├───java │ │ └───com │ │ └───zetcode │ │ ├───bean │ │ │ User.java │ │ ├───config │ │ │ MyWebInitializer.java │ │ │ WebConfig.java ...
What is the body part of an HTTP request?
The message body part is optional for an HTTP message but if it is available then it is used to carry the entity-body associated with the request or response. If entity body is associated then usually Content-Type and Content-Length headers lines specify the nature of the body associated.
What is HTTP request?
Most HTTP requests are GET requests without bodies. However, simulating requests with bodies is important to properly stress the proxy code and to test various hooks working with such requests. Most HTTP requests with bodies use POST or PUT request method. Message Body.
What is body data in HTTP?
HTTP Body Data is the data bytes transmitted in an HTTP transaction message immediately following the headers if there is any ( in the case of HTTP/0.9 no headers are transmitted).
What is a message body?
A message body is the one which carries actual HTTP request data (including form data and uploaded etc.) and HTTP response data from the server ( including files , images etc). Following is a simple content of a message body:
What are single resource bodies?
Single-resource bodies, consisting of one single file, defined by the two headers: Content-Type and Content-Length.
1. Introduction
In this quick tutorial, we provide a concise overview of the Spring @RequestBody and @ResponseBody annotations.
Guide to Spring Handler Mappings
The article explains how HandlerMapping implementation resolve URL to a particular Handler.
Quick Guide to Spring Controllers
A quick and practical guide to Spring Controllers - both for typical MVC apps and for REST APIs.
4. Conclusion
We've built a simple Angular client for the Spring app that demonstrates how to use the @RequestBody and @ResponseBody annotations.
What is response body annotation?
The @ResponseBody annotation tells a controller that the object returned is automatically serialized into JSON and passed back into the HttpResponse object.
What is the @RestController annotation?
Spring 4.0 introduced @RestController, a specialized version of the controller which is a convenience annotation that does nothing more than adding the @Controller and @ResponseBody annotations. By annotating the controller class with @RestController annotation, you no longer need to add @ResponseBody to all the request mapping methods. The @ResponseBody annotation is active by default.
What parameter is the body of web request assigned to?
Now the body of web request will be assigned to book parameter.
What is @request body in Spring?
This page will walk through Spring @RequestBody annotation example. The @RequestBody is annotated at method parameter level to indicate that this method parameter will bound to web request body. The consumes attribute of @RequestMapping can specify the media types acceptable to @RequestBody parameter. The @RequestBody can be used with HTTP methods POST, PUT etc. Hence we can use @RequestBody parameter in the methods annotated with @PostMapping and @PutMapping etc.
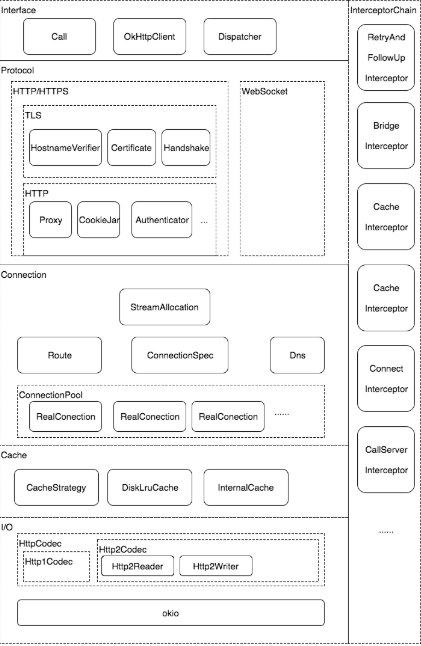