
Singleton Class in C# A singleton class only allows a single instance of itself to be created and gives easy access to that instance. Typically we cannot specify any parameters while initializing an instance of a singleton class.
What are the properties of a singleton class in C #?
This tutorial will discuss the properties of a Singleton class in C#. A singleton class only allows a single instance of itself to be created and gives easy access to that instance. Typically we cannot specify any parameters while initializing an instance of a singleton class. The instances of the singleton classes must be initialized lazily.
What is the use of singleton class in Python?
It is used where only a single instance of a class is required to control the action throughout the execution. A singleton class shouldn’t have multiple instances in any case and at any cost. Singleton classes are used for logging, driver objects, caching and thread pool, database connections.
What is singleton pattern in Java?
Singleton pattern is a design pattern which restricts a class to instantiate its multiple objects. It is nothing but a way of defining a class. Class is defined in such a way that only one instance of the class is created in the complete execution of a program or project.
How do you access a singleton instance in Java?
Instance should be globally accessible : Instance of singleton class should be globally accessible so that each class can use it. In Java, it is done by making the access-specifier of instance public. Early initialization : In this method, class is initialized whether it is to be used or not.
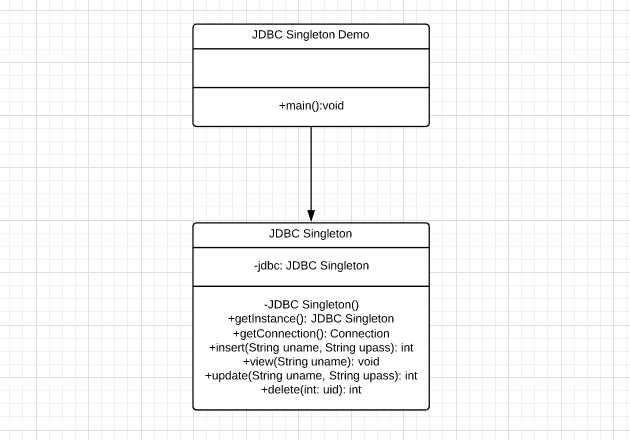
What is the purpose of singleton class?
The Singleton's purpose is to control object creation, limiting the number to one but allowing the flexibility to create more objects if the situation changes. Since there is only one Singleton instance, any instance fields of a Singleton will occur only once per class, just like static fields.
What is singleton class in C?
C # in Telugu Singleton design pattern is a software design principle that is used to restrict the instantiation of a class to one object. This is useful when exactly one object is needed to coordinate actions across the system.
Where is Singleton useful?
Singleton pattern is used for logging, drivers objects, caching and thread pool. Singleton design pattern is also used in other design patterns like Abstract Factory, Builder, Prototype, Facade etc. Singleton design pattern is used in core java classes also, for example java. lang.
What is an example of a singleton?
Example. The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. It is named after the singleton set, which is defined to be a set containing one element. The office of the President of the United States is a Singleton.
Is singleton a static class?
While a static class allows only static methods and and you cannot pass static class as parameter. A Singleton can implement interfaces, inherit from other classes and allow inheritance. While a static class cannot inherit their instance members. So Singleton is more flexible than static classes and can maintain state.
How does a singleton work?
A singleton is a class that allows only a single instance of itself to be created and gives access to that created instance. It contains static variables that can accommodate unique and private instances of itself. It is used in scenarios when a user wants to restrict instantiation of a class to only one object.
What is the advantage of Singleton design pattern?
Advantage of Singleton design pattern Saves memory because object is not created at each request. Only single instance is reused again and again.
How do you implement a singleton?
The most popular approach is to implement a Singleton by creating a regular class and making sure it has: A private constructor. A static field containing its only instance. A static factory method for obtaining the instance.
Are singletons thread safe?
Is singleton thread safe? A singleton class itself is not thread safe. Multiple threads can access the singleton same time and create multiple objects, violating the singleton concept. The singleton may also return a reference to a partially initialized object.
What is a singleton pointer?
A singleton is like a global that will only be created once and can be accessed whenever. Check this for more: infernodevelopment.com/singleton-c. – NKamrath.
What does @singleton annotation do?
The @Singleton annotation is used to declare to Dagger that the provided object is to be only initialized only once during the entire lifecycle of the Component which uses that Module.
How do you implement a singleton?
The most popular approach is to implement a Singleton by creating a regular class and making sure it has: A private constructor. A static field containing its only instance. A static factory method for obtaining the instance.
What is singleton in C++?
In C++, singletons are a way of defining a class such that only a single instance of a class is created in the whole program. In this article, you will learn what is singleton class in C++ and how to create its design patterns.
How to make a singleton class in C++?
In C++ you can create a singleton class using four different methods: the classic implementation using a private constructor, the making of get-instant () synchronized, the eager instantiation, and the double checked locking method.
How To Implement the Singleton Class Design Pattern in C++?
In C++ to create a singleton class design pattern the program is divided into the following three parts:
What is lazy initialization?
This type of initialization saves memory and time. It is the concept that is commonly used to initialize the object at the point when it is needed. Lazy initialization is applied when the object is very rarely used.
Why should singleton classes be global?
This is only possible if we make a static method and create an access specifier public.
What keyword is used to reduce overhead of calling a synchronized method every time?
In the code presented above, to reduce the overhead of calling the synchronized method every time, we used the volatile keyword for the object.
Which method is best for creating a singleton design pattern?
Among all four methods, the best and most preferred method is creating a singleton design pattern using the double-checked locking method. This method overcomes all the drawbacks of other methods.
What is singleton in C#?
In other words, a singleton is a class that allows only a single instance of itself to be created and usually gives simple access to that instance. There are various ways to implement a singleton pattern in C#. The following are the common characteristics of a singleton pattern. Private and parameterless single constructor.
What are the advantages of singleton pattern?
The advantages of a Singleton Pattern are, Singleton pattern can implement interfaces. Can be lazy-loaded and has Static Initialization. It helps to hide dependencies. It provides a single point of access to a particular instance, so it is easy to maintain.
What is Singleton Design Pattern?
In other words, a singleton is a class that allows only a single instance of itself to be created and usually gives simple access to that instance.
How many times does a static constructor execute?
This type of implementation has a static constructor, so it executes only once per Application Domain .
Can you pass a delegate to a constructor?
You can pass a delegate to the constructor that calls the Singleton constructor, which is done most easily with a lambda expression.
Can a static class be extended?
A Static Class cannot be extended whereas a singleton class can be extended. A Static Class cannot be initialized whereas a singleton class can be. A Static class is loaded automatically by the CLR when the program containing the class is loaded.
Where to Use Singleton Class?
There are situations where creating multiple instances of a class is not a good idea. It might create problem also.
Author: Srikanta
I write here to help the readers learn and understand computer programing, algorithms, networking, OS concepts etc. in a simple way. I have 20 years of working experience in computer networking and industrial automation. View all posts by Srikanta
What is singleton class?
A singleton class only allows a single instance of itself to be created and gives easy access to that instance. Typically we cannot specify any parameters while initializing an instance of a singleton class. The instances of the singleton classes must be initialized lazily. It means that an instance must only be initialized when it is first needed.
Is it advisable to use singleton in C#?
Normally, it is never advisable to use the singleton pattern in C#. It is because, no matter what our situation is, there is always a better and more elegant solution or approach available in C#. The singleton pattern is one of those things that we should be aware of but never use in our applications. Contribute.
Why use singleton class?
Singleton classes are also used to prevent concurrent access of class. Practically singleton can be used in case external hardware resource usage limitation required e.g. Hardware printers where the print spooler can be made a singleton to avoid multiple concurrent accesses and creating deadlock.
What are the applications of singleton pattern?
There is a lot of applications of singleton pattern like cache-memory, database connection, drivers, logging. Some major of them are :-
Why is the constructor private in Java?
This is done by making the constructor private in java so that no class can access the constructor and hence cannot instantiate it. Instance should be globally accessible : Instance of singleton class should be globally accessible so that each class can use it.
What is singleton pattern?
Singleton pattern is a design pattern which restricts a class to instantiate its multiple objects. It is nothing but a way of defining a class. Class is defined in such a way that only one instance of the class is created in the complete execution of a program or project. It is used where only a single instance of a class is required to control ...
Why use configuration file in singleton?
Configuration File: This is another potential candidate for Singleton pattern because this has a performance benefit as it prevents multiple users to repeatedly access and read the configuration file or properties file. It creates a single instance of the configuration file which can be accessed by multiple calls concurrently as it will provide static config data loaded into in-memory objects. The application only reads from the configuration file for the first time and thereafter from second call onwards the client applications read the data from in-memory objects.
How many instances should a singleton class have?
An implementation of singleton class should have following properties: It should have only one instance : This is done by providing an instance of the class from within the class. Outer classes or subclasses should be prevented to create the instance.
When to use lazy initialization?
It can save you from instantiating the class when you don’t need it. Generally, lazy initialization is used when we create a singleton class.
