
What are the different types of JPA queries?
There are three basic types of JPA Queries: Query, written in Java Persistence Query Language (JPQL) syntax NativeQuery, written in plain SQL syntax Criteria API Query, constructed programmatically via different methods
How do I create a typedquery in hibernate?
In Hibernate, you use session.createQuery ()“. TypedQuery gives you an option to mention the type of entity when you create a query and therefore any operation thereafter does not need an explicit cast to the intended type. Whereas the normal Query API does not return the exact type of Object you expect and you need to cast.
What is the difference between native query and namedquery?
Similar to how the constant is defined. NamedQuery is the way you define your query by giving it a name. You can define this in mapping files of hibernate and also use annotations at the entity level. Native query refers to actual SQL queries (referring to actual database objects).
Can a typedquery have a generic parameter?
The TypedQuery can have generic parameter. The following code is from Professor.java. The following code is from PersonDaoImpl.java.
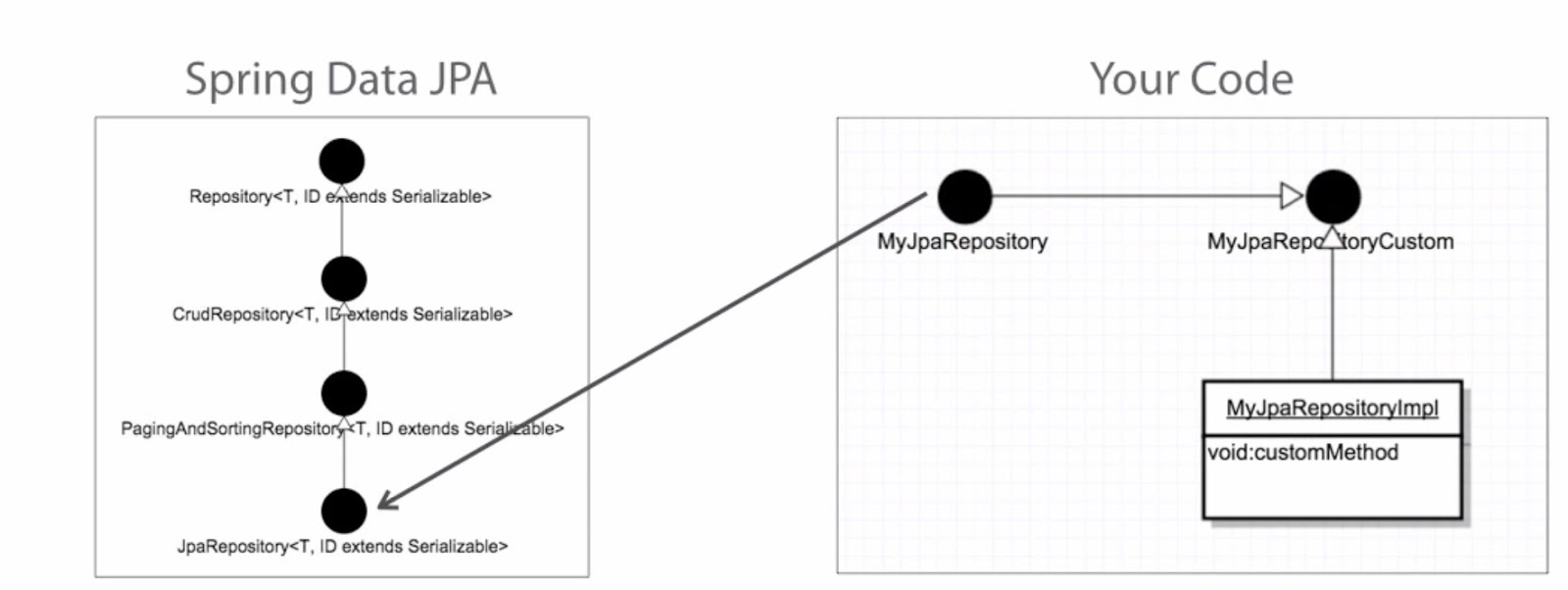
What is TypedQuery in Hibernate?
You could define this in mapping file in hibernate or also using annotations at entity level. TypedQuery. TypedQuery gives you an option to mention the type of entity when you create a query and therefore any operation thereafter does not need an explicit cast to the intended type.
What is difference between native and named query?
Native query refers to actual sql queries (referring to actual database objects). These queries are the sql statements which can be directly executed in database using a database client. 2. Named query is the way you define your query by giving it a name.
How do you use typed queries?
Execute a SELECT query and return the query results as a typed List. Execute a SELECT query that returns a single result. Set the position of the first result to retrieve. Set the flush mode type to be used for the query execution.
What is the difference between native query and JPQL?
There are three basic types of JPA Queries: Query, written in Java Persistence Query Language (JPQL) syntax. NativeQuery, written in plain SQL syntax. Criteria API Query, constructed programmatically via different methods.
What is Createnativequery in JPA?
Imagine having a tool that can automatically detect JPA and Hibernate performance issues.
Is native query faster than JPA?
This test run has 11008 records in the Order table, 22008 records in the LineItem table, and 44000 records in the Customer table. It shows that JPA queries are as fast as their equivalent JDBC queries....Breadcrumbs.Query TypesTime in secondsJPA Native Queries53JDBC Queries622 more rows•Aug 2, 2010
What is Criteriabuilder in JPA?
CriteriaBuilderJPA interfaceUsed to construct criteria queries, compound selections, expressions, predicates, orderings. See JavaDoc Reference Page... interface serves as the main factory of criteria queries and criteria query elements. It can be obtained either by the EntityManagerFactory. persistence.
How do I write a JPA query?
Creating SQL Queries Add a query method to our repository interface. Annotate the query method with the @Query annotation, and specify the invoked query by setting it as the value of the @Query annotation's value attribute. Set the value of the @Query annotation's nativeQuery attribute to true.
Can getResultList return null?
getResultList() returns an empty list instead of null .
What is the difference between @query and Namedquery?
Testplan > Mark by Query or Testplan > Mark by Named Query both create queries, however, Mark by Named Query provides extra features, like the ability to combine queries or to create a query without running it immediately....The Differences between Query and Named Query Commands.Mary by QueryMark by Named QueryCannot combine queries.Can combine queries into a new query.4 more rows
Does JPA use JPQL?
JPQL is Java Persistence Query Language defined in JPA specification. It is used to create queries against entities to store in a relational database.
What is the full form of JPQL?
The Java Persistence query language (JPQL) is used to define searches against persistent entities independent of the mechanism used to store those entities.
What is a named query?
A named query is a SQL expression represented as a table. In a named query, you can specify an SQL expression to select rows and columns returned from one or more tables in one or more data sources.
What are native sql queries?
Native SQL is the SQL that the data source uses, such as Oracle SQL. Use Native SQL to pass the SQL statement that you enter to the database. IBM® Cognos® Analytics may add statements to what you enter. You can not use native SQL in a query subject that references more than one data source in the project.
What is native query in spring boot?
We can use @Query annotation to specify a query within a repository. Following is an example. In this example, we are using native query, and set an attribute nativeQuery=true in Query annotation to mark the query as native. We've added custom methods in Repository in JPA Custom Query chapter.
What is the use of native query in Hibernate?
You can use native SQL to express database queries if you want to utilize database-specific features such as query hints or the CONNECT keyword in Oracle. Hibernate 3. x allows you to specify handwritten SQL, including stored procedures, for all create, update, delete, and load operations.
What is the difference between query, native query, named query and typed query
Here is a quick tutorial on stating the differences between the query, native query, named query, and typed query.
Query
Query refers to JPQL/HQL query with syntax similar to SQL generally used to execute DML statements (CRUD operations).
TypedQuery
TypedQuery gives you an option to mention the type of entity when you create a query and therefore any operation thereafter does not need an explicit cast to the intended type. Whereas the normal Query API does not return the exact type of Object you expect and you need to cast.
NamedQuery
Similar to how the constant is defined. NamedQuery is the way you define your query by giving it a name. You can define this in mapping files of hibernate and also use annotations at the entity level.
NativeQuery
Native query refers to actual SQL queries (referring to actual database objects). These queries are the SQL statements that can be directly executed in the database using a database client.
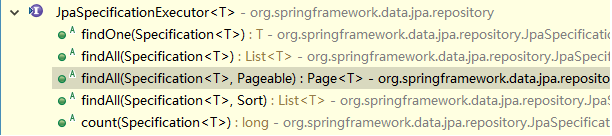
Overview
Setup
- Firstly, let's define the UserEntityclass we'll use for all examples in this article: There are three basic types of JPA Queries: 1. Query, written in Java Persistence Query Language (JPQL) syntax 2. NativeQuery, written in plain SQL syntax 3. Criteria API Query, constructed programmatically via different methods Let's explore them.
Query
- A Queryis similar in syntax to SQL, and it's generally used to perform CRUD operations: This Query retrieves the matching record from the users table and also maps it to the UserEntityobject. There are two additional Querysub-types: 1. TypedQuery 2. NamedQuery Let's see them in action.
Nativequery
- A NativeQueryis simply an SQL query. These allow us to unleash the full power of our database, as we can use proprietary features not available in JPQL-restricted syntax. This comes at a cost. We lose the database portability of our application with NativeQuerybecause our JPA provider can't abstract specific details from the database implementation or vendor anymore. Let's see how t…
Query, Namedquery, and Nativequery
- So far, we've learned about Query, NamedQuery, and NativeQuery. Now, let's revisit them quickly and summarize their pros and cons.
Criteria API Query
- Criteria API queriesare programmatically-built, type-safe queries – somewhat similar to JPQL queries in syntax: It can be daunting to use Criteria API queries first-hand, but they can be a great choice when we need to add dynamic query elements or when coupled with the JPA Metamodel.
Conclusion
- In this quick article, we learned what JPA Queries are, along with their usage. JPA Queries are a great way to abstract our business logic from our data access layer as we can rely on JPQL syntax and let our JPA provider of choice handle the Querytranslation. All code presented in this article is available over on GitHub.