
In general, class declarations can include these components, in order:
- Modifiers such as public, private, and a number of others that you will encounter later. ...
- The class name, with the initial letter capitalized by convention.
- The name of the class's parent (superclass), if any, preceded by the keyword extends. ...
- A comma-separated list of interfaces implemented by the class, if any, preceded by the keyword implements. ...
- The class body, surrounded by braces, {}.
- Modifiers such as public, private, and a number of others that you will encounter later. ...
- The class name, with the initial letter capitalized by convention.
- The name of the class's parent (superclass), if any, preceded by the keyword extends.
What are five parts of method declaration?
The syntax of a method declaration consists of the following points:Modifier. We learned about access modifiers in previous articles. ... return type. This is the return type of the method. ... method name. A method name should typically represent what its function is. ... parameter list. ... Exception list. ... Method body. ... Method Signature.
What are the keywords in the class declaration?
The keyword class. An identifier that names the class. An optional extends clause that specifies the superclass of the declared class. An optional implements clause that specifies the interfaces implemented by the declared class.
Which declarations are required in a Java program?
5) What declarations are required for every Java application? Ans: A class and the main( ) method declarations.
What is class definition and class declaration?
A class forward-declaration is a pure declaration (or re-declaration), a class definition is both a (re-)declaration and a definition. A class has to be defined (and therefore, declared) before instances are created from it. – dyp.
What is an example of class declaration?
Thus the simplest class declaration that you can write looks like this: class NameOfClass { . . . } For example, this code snippet declares a new class named ImaginaryNumber. Class names must be a legal Java identifier and, by convention, begin with a capital letter.
What is a class declaration in C++?
A class declaration creates a unique type class name. A class specifier is a type specifier used to declare a class. Once a class specifier has been seen and its members declared, a class is considered to be defined even if the member functions of that class are not yet defined.
What are the 4 types of variables in Java programming language?
In Java, there are different types of variables, for example:String - stores text, such as "Hello". ... int - stores integers (whole numbers), without decimals, such as 123 or -123.float - stores floating point numbers, with decimals, such as 19.99 or -19.99.char - stores single characters, such as 'a' or 'B'.More items...
What are declarations in Java?
To create a variable, you must tell Java its type and name. Creating a variable is also called declaring a variable. When you create a primitive variable Java will set aside enough bits in memory for that primitive type and associate that memory location with the name that you used.
What is declaration in Java program?
The variable declaration means creating a variable in a program for operating different information. The Java variable declaration creates a new variable with required properties. The programming language requires four basic things to declare a variable in the program. Data-type. Variable name.
Which of the following is a valid class declaration?
Which of the following is a valid class declaration? Explanation: A class declaration terminates with semicolon and starts with class keyword. only option (a) follows these rules therefore class A { int x; }; is correct.
How do you declare a class definition?
Declaration and Definition of Class in C++ You can define classes using the keyword 'class' followed by the name of the class. Here, inside the class, there are access-modifiers, data variables, and member functions.
What is a class give a general form of a class declaration?
The general form of a class declaration is shown here. class class-name { private data and functions public: public data and functions } object-list; Here class-name specifies the name of the class. This name becomes a new type name that can be used to create objects of the class.
How do you declare a class definition?
Declaration and Definition of Class in C++ You can define classes using the keyword 'class' followed by the name of the class. Here, inside the class, there are access-modifiers, data variables, and member functions.
What is the basic syntax for class declaration?
The basic syntax is: class MyClass { // class methods constructor() { ... } method1() { ... } method2() { ... }
How will you declare a class in Python?
Python Classes and ObjectsCreate a Class. To create a class, use the keyword class : ... Create Object. Now we can use the class named MyClass to create objects: ... The self Parameter. ... Modify Object Properties. ... Delete Object Properties. ... Delete Objects.
How do you declare a class in C#?
Declaring Classes The name of the class follows the class keyword. The name of the class must be a valid C# identifier name. The remainder of the definition is the class body, where the behavior and data are defined. Fields, properties, methods, and events on a class are collectively referred to as class members.
Where does a class declaration appear in a function?
A class declaration can appear inside the body of a function, in which case it defines a local class. The name of such a class only exists within the function scope, and is not accessible outside. A local class inside a function (including member function) can access the same names that the enclosing function can access.
What is a member specification?
The member specification, or the body of a class definition, is a brace-enclosed sequence of any number of the following: sequence of specifiers. It is only optional in the declarations of constructors, destructors, and user-defined type conversion functions.
What is a semicolon in a function?
2) Function definitions, which both declare and define member functions or friend functions. A semicolon after a member function definition is optional. All functions that are defined inside a class body are automaticaly inline .
Can local classes have friend templates?
Local classes cannot have friend templates. Local classes cannot define friend functions inside the class definition. A local class inside a function (including member function) can access the same names that the enclosing function can access. local classes could not be used as template arguments.
What Are Classes in C++?
A class is a user-defined data type representing a group of similar objects, which holds member functions and variables together. In other words, a class is a collection of objects of the same kind. For example, Facebook, Instagram, Twitter, and Snapchat all come under social media class.
What is an object in a class?
Objects of a Class. An object is a recognizable entity having a state and behavior, and these objects hold variables of a class in accordance with the access modifiers. It is also known as an instance of a class. You can call a member function with the help object and use the dot operator.
What is a constructor in a class?
The constructor's name is like that of the class, and it doesn’t have any return type. Even if you do not include a constructor in the class, the compiler creates a default constructor. These are generally used for assigning initial values to variables. Generally, they are of three types: Parameterized constructor.
What is a class in social media?
A class is a user-defined data type representing a group of similar objects, which holds member functions and variables together. In other words, a class is a collection of objects of the same kind. For example, Facebook, Instagram, Twitter, and Snapchat all come under social media class.
Can you access the public members of a class?
Public: You can access the public members from within, as well as from outside of the class.
What is a basic type?
We shall use the following definition of type expressions: • A basic type is a type expression. Typical basic types for a language include boolean, char, integer, float, and void; the latter denotes "the absence of a value.". A type name is a type expression.
How are type expressions formed?
They are the same basic type. They are formed by applying the same constructor to structurally equiv-alent types. One is a type name that denotes the other. If type names are treated as standing for themselves, then the first two condi-tions in the above definition lead to name equivalence of type expressions.
What is a nonterminal D?
Nonterminal D generates a sequence of declarations. Nonterminal T gen-erates basic, array, or record types. Nonterminal B generates one of the basic types int and float. Nonterminal C, for "component," generates strings of zero or more integers, each integer surrounded by brackets. An array type con-sists of a basic type specified by B, followed by array components specified by nonterminal C. A record type (the second production for T) is a sequence of declarations for the fields of the record, all surrounded by curly braces.
How to form a type expression?
A type expression can be formed by applying the array type constructor to a number and a type expression.
What is the width of a type?
The width of a type is the number of storage units needed for objects of that type. A basic type, such as a character, integer, or float, requires an integral number of bytes. For easy access, storage for aggregates such as arrays and classes is allocated in one contiguous block of bytes.4
What is type information?
Type information is also needed to calculate the address denoted by an array reference, to insert explicit type conversions, and to choose the right ver-sion of an arithmetic operator, among other things.
When are two type expressions equivalent?
When are two type expressions equivalent? Many type-checking rules have the form, "if two type expressions are equal t h e n return a certain type else error." Potential ambiguities arise when names are given to type expressions and the names are then used in subsequent type expressions. The key issue is whether a name in a type expression stands for itself or whether it is an abbreviation for another type expression.
Can you take out class D?
If you have access to change the source code of classes C and D, then you can take out class D separately, and enter a synonym for it in class C:
Can you forward declare a nested type?
But you can't forward declare a nested type, the following causes compilation error.
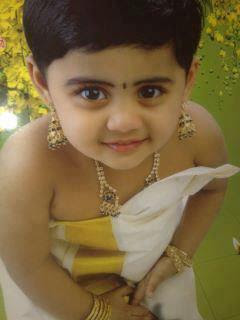
Forward Declaration
- A declaration of the following form Declares a class type which will be defined later in this scope. Until the definition appears, this class name has incomplete type. This allows classes that refer to each other: and if a particular source file only uses pointers and references to the class, this makes it possible to reduce #include dependencies: If forward declaration appears in local scop…
Member Specification
- The member specification, or the bodyof a class definition, is a brace-enclosed sequence of any number of the following:
Local Classes
- A class declaration can appear inside the body of a function, in which case it defines a local class. The name of such a class only exists within the function scope, and is not accessible outside. 1. A local class cannot have static data members 2. Member functions of a local class have no linkage 3. Member functions of a local class have to be def...
Defect Reports
- The following behavior-changing defect reports were applied retroactively to previously published C++ standards.