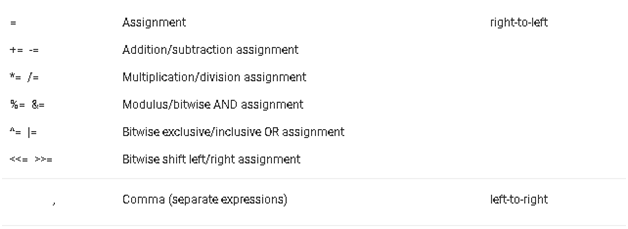
Operators Precedence & Associativity Table
Operator | Meaning of operator | Associativity |
() [] -> . | Functional call Array element reference ... | Left to right |
! ~ + - ++ -- & * sizeof (type) | Logical negation Bitwise (1 's) compleme ... | Right to left |
* / % | Multiply Divide Remainder | Left to right |
+ - | Binary plus (Addition) Binary minus (sub ... | Left to right |
Operator | Description of Operator | Associativity |
---|---|---|
— | Decrement | Right to left |
++ | Increment | Right to left |
* | Pointer reference | Right to left |
& | Dereference (Address) | Right to left |
Why is the associativity of = operator from right to left?
It's because the associativity of the = operator is from right to left. Also, if two operators of the same precedence (priority) are present, associativity determines the direction in which they execute. Let us consider an example: Here, operators == and != have the same precedence. And, their associativity is from left to right.
What is the associativity of = operator in C?
The associativity of = operator is from right to left. So the value of c (i.e. 3) is assigned to b, and then the value of b is assigned to a. So after executing this statement, the values of a, b and c will be 3. The table below shows the associativity of C# operators: C# Associativity of operators.
Is <<= right to left associative in C and C++?
However, some operators in C and C++, are right to left associative. I wrote the following program, to demonstrate that <<= is right to left associative. In the first two examples, the answer 8 is printed. But for the last one, 4 is printed.
What is operator precedence and operator associativity?
Operator precedence specifies the order of operations in expressions that contain more than one operator. Operator associativity specifies whether, in an expression that contains multiple operators with the same precedence, an operand is grouped with the one on its left or the one on its right.
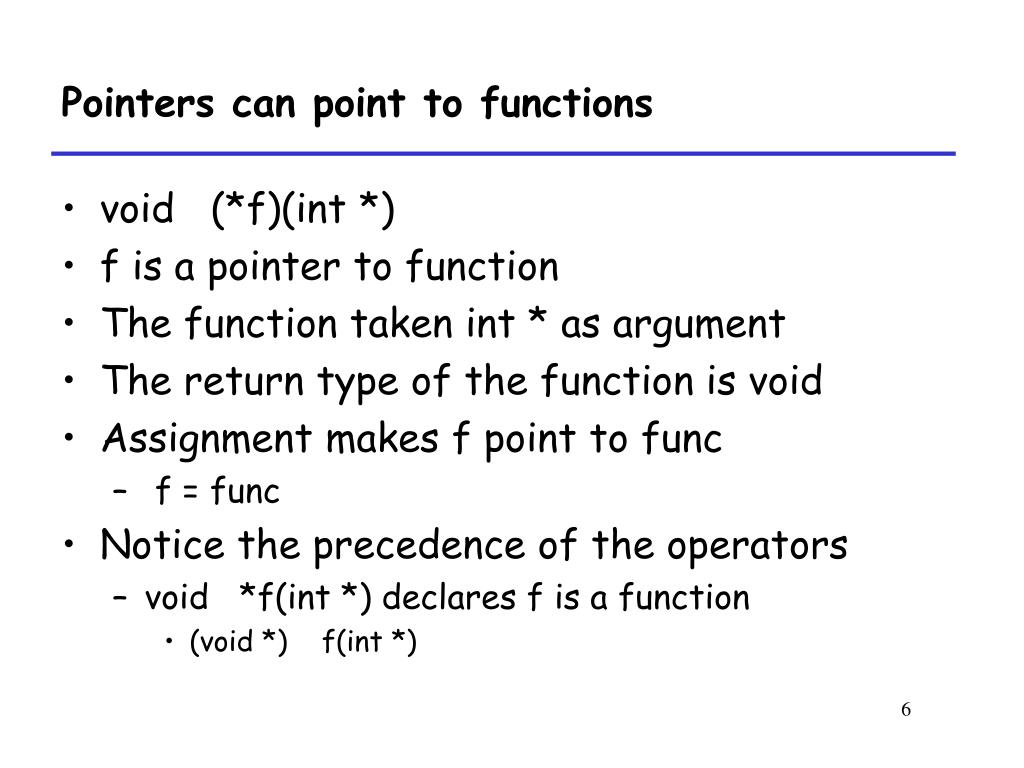
Precedence of operators
The precedence of operators determines which operator is executed first if there is more than one operator in an expression.
Associativity of Operators
The associativity of operators determines the direction in which an expression is evaluated. For example,
What is an infix operator in C++?
An infix operator (or more generally expression type that has unclosed left and right sub-expressions) is left associative if in nested use of this operator (expression type) without explicit parentheses, the implicit parentheses are placed at the left. Since * is left-associative in C++, a*b*c means (a*b)*c. In case of deeper nesting, a cluster of implicit parentheses occurs on the left end: ( ( (a*b)*c)*d)*e.
What does left to right associativity mean?
Left to right associativity of a operator means right side of operator should not have any operator of higher precedece (priority), but it can be of same priority. If there is any operator of higher priority on right side of our operator, then we have to solve it first.example:
What does left associativity mean in math?
Back to the example from your question, left-associativity (or grouping left-to-right) means that your matrix-vector product is parsed as ( (M1*M2)*M3)*v. Although mathematically equivalent, it is virtually impossible that this gets executed as M1* (M2* (M3*v)), even though that is more efficient. The reason is that floating-point multiplication is not truly associative (just approximately), nor is therefore floating-point matrix multiplication; the compiler therefore cannot transform one expression into the other. Note that in ( (M1*M2)*M3)*v one cannot say which of the matrices gets applied to a vector first, because none of them is: the matrix of the composite linear maps gets computed first, and the that matrix gets applied to the vector. The result will be approximately equal to that of M1* (M2* (M3*v)) in which M3 is applied, then M2 and finally M1. But if you want things to happen like that, you have to write those parentheses.
Why does addition matter in math?
For addition in mathematics it does't matter too much. You always get the same result either way. This is because addition is associative . To say an operation is associative means left to right and right to left association are the same thing. For addition in programming it still matters because of overflows and floating point arithmetic (but won't for normal-sized integers in any reasonable language), so when you have a 2 AM bug with large numbers and flippant use of a+b and b+a, remember what order the addition happened in.
Why does GLM associate left to right?
glm's matrix multiplcation associates left-to-right because it overloads the * operator. The issue here is about the meaning of the matrix multiplications in terms of geometrical transformations, rather than the mathematical associativity.
Which operator takes its immediate neighbours as operands?
Consequently, in a nested use without explicit parentheses, the leftmost operator takes its immediate neighbours as operands, while the other instances take as left operand the (result of) the expression formed by everything to their left.
What is the meaning of "back up"?
Making statements based on opinion; back them up with references or personal experience.
Precedence and associativity
Operator precedence specifies the order of operations in expressions that contain more than one operator. Operator associativity specifies whether, in an expression that contains multiple operators with the same precedence, an operand is grouped with the one on its left or the one on its right.
Alternative spellings
C++ specifies alternative spellings for some operators. In C, the alternative spellings are provided as macros in the <iso646.h> header. In C++, these alternatives are keywords, and use of <iso646.h> or the C++ equivalent <ciso646> is deprecated.
What is operator precedence in C#?
Operator precedence is a set of rules which defines how an expression is evaluated. In C#, each C# operator has an assigned priority and based on these priorities, the expression is evaluated.
What is operand 3?
The operand 3 is associated with + and *. As stated earlier, multiplication has a higher precedence than addition. So, the operation 3 * 5 is carried out instead of 4 + 3. The value of variable x will be 19.
What is the precedence of + in the next expression?
In the next expression, the precedence of + is higher than >=. So, c and a is added first and the sum is compared with b to produce false.
Which operator has the lowest precedence?
The assignment operators have the lowest precedence while the postfix increment and decrement operators have the highest precedence.
What is the value of x if addition has a higher precedence?
If addition would have a higher precedence, 4 + 3 would be evaluated first and the value of x would be 35 .
Does a * and / have the same precedence?
Here, both * and / have the same precedence. But since the associativity of these operators is from left to right, a * b is evaluated first and then division is carried out. The final result of this expression will be 10.
Is the expression inside parentheses always evaluated first?
The expression inside parentheses is always evaluated first no matter what the precedence of operators outside it is .
Which operator evaluates left to right?
Second, the conditional operator evaluates left to right. First it checks the condition item (i.e. the IF portion). Then depending on the value there it either continues to the THEN portion or
What does it mean when an operator is left to right?
When an operator has left-to-right associativity, that means its operands group left to right. For example, the addition operator, + is left-to-right associative. By “grouping left to right,” the intuition here is that you evaluate the expressions that are the same precedence left to right.
What is associativity in programming?
In programming, associativity means which operands are bound to an operator (“are associated to it”) when parentheses are missing. This boils down the question in which order to evaluate the expression.
What is conditional operator?
A conditional operator in a programming language helps us to execute a set of commands on the basis of few conditions .
What are conditional operators used for in lambda?
Conditional operators can also be used in lambda exnsessions and field initializers that are part of complicated statements that would be difficult to break apart into an if/else statement.
What is Aspose.TeX for C++?
Aspose.TeX for C++ is flexible and easy to use library to typeset TeX files.
Who said "I had confused myself by thinking that associativity specifies the order in which the operands?
This statement from Mr. Sergey Zubkov clarified the doubt in my Mind!#N#I had confused myself by thinking that associativity specifies the order in which the operands of an operator are 'evaluated'!#N#This was where I was going wrong. Knowing this, Now All My doubts and confusions got cleared!
What is the right to left associativity in C++?
Explanation: There are many rights to left associativity operators in C++, which means they are evaluation is done from right to left. Type Cast is one of them. Here is a link of the associativity of operators.
What is the operator of &&?
Explanation: && is called as Logical AND operator, if there is no zero in the operand means, it will be true otherwise false.
Which direction does the flow of execution go in assignment operation?
Explanation: In assignment operation, the flow of execution will be from right to left only.
When we want to modify the value of a variable by performing an operation on the value currently stored, we will use?
Explanation: When we want to modify the value of a variable by performing an operation on the value currently stored, We will use compound assignment statement. In this option, a -=5 is equal to a = a-5.
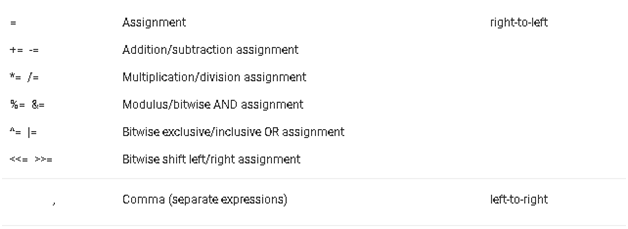