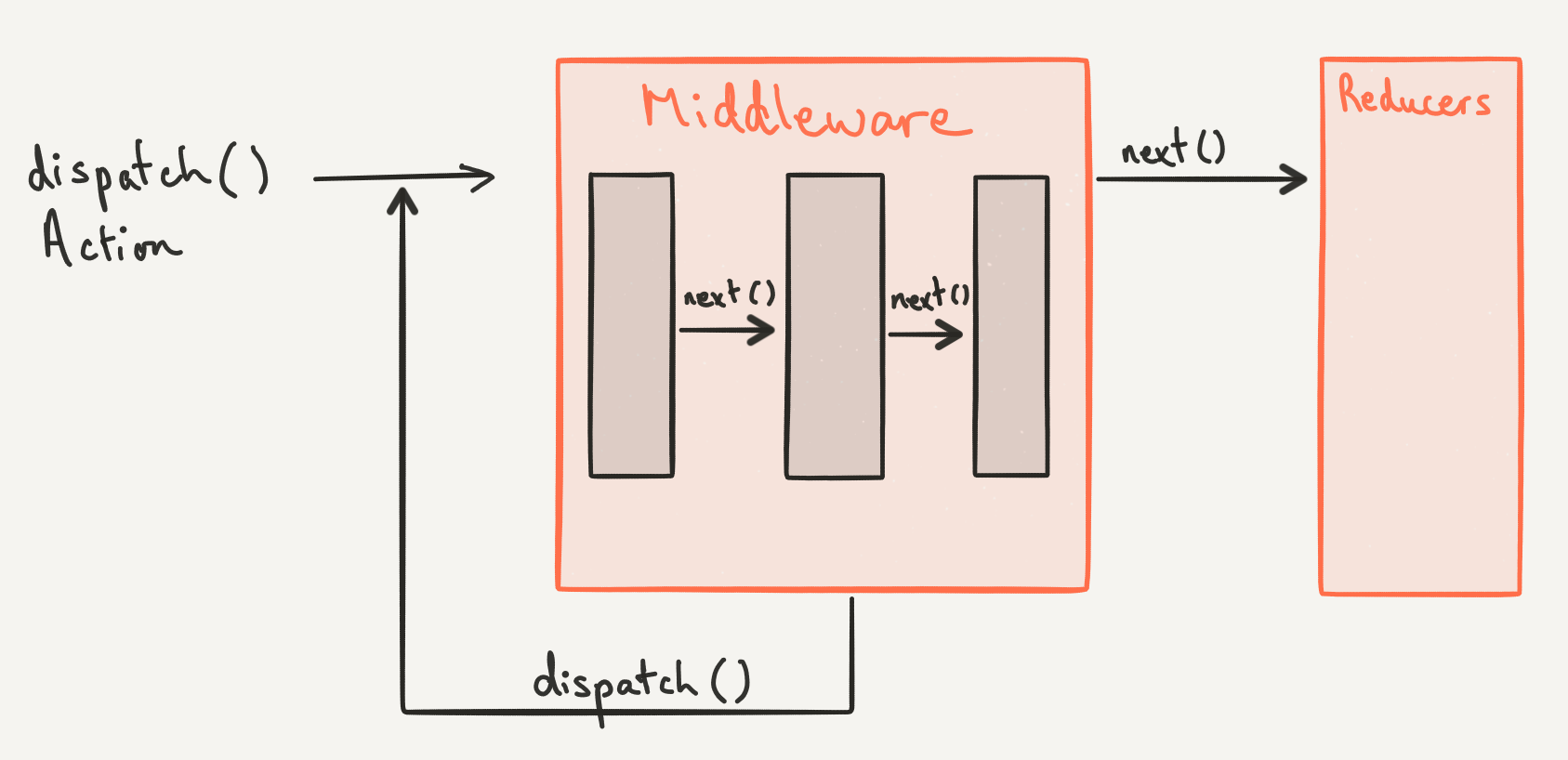
Redux Middleware allows you to intercept every action sent to the reducer so you can make changes to the action or cancel the action. Middleware helps you with logging, error reporting, making asynchronous requests, and a whole lot more. What is the purpose of middleware in Redux?
What is a middleware in Redux?
In the context of redux middleware such as redux-thunk, a middleware helps us deal with asynchronous action creators as that is something that Redux cannot handle out of the box.
Does Redux store support asynchronous data flow without middleware?
According to the docs, "Without middleware, Redux store only supports synchronous data flow". I don't understand why this is the case. Why can't the container component call the async API, and then dispatch the actions?
What is Redux saga and how does it work?
Another interesting approach is Redux Saga which lets you define long-running daemons (“sagas”) that take actions as they come, and transform or perform requests before outputting actions. This moves the logic from action creators into sagas.
How do reducers work in Redux?
It is based on the reduce function in JavaScript, where a single value is calculated from multiple values after a callback function has been carried out. Here is an example of how reducers work in Redux: Reducers take the previous state of the app and return a new state based on the action passed to it.

Why do we need middleware in React?
Middleware allows for side effects to be run without blocking state updates. We can run side effects (like API requests) in response to a specific action, or in response to every action that is dispatched (like logging). There can be numerous middleware that an action runs through before ending in a reducer.
Can we use Redux without middleware?
The answer is Yes! This blog will try to explain on how to implement async action calls in redux without the use of any middlewares. We will just implement two API calls to not to over complicate things. Create a new file called api.
Where is Redux middleware used?
Redux middleware provides a third-party extension point between dispatching an action, and the moment it reaches the reducer. People use Redux middleware for logging, crash reporting, talking to an asynchronous API, routing, and more.
What is Redux Thunk middleware when and why would we need it?
Redux Thunk is a middleware that lets you call action creators that return a function instead of an action object. That function receives the store's dispatch method, which is then used to dispatch regular synchronous actions inside the function's body once the asynchronous operations have been completed.
What is middleware in Redux toolkit?
Overview A Redux middleware that lets you define "listener" entries that contain an "effect" callback with additional logic, and a way to specify when that callback should run based on dispatched actions or state changes.
Can we use multiple middleware in Redux?
Multiple middleware can be combined together, where each middleware requires no knowledge of what comes before or after it in the chain. The most common use case for middleware is to support asynchronous actions without much boilerplate code or a dependency on a library like Rx.
What is the use of middleware?
Middleware is software which lies between an operating system and the applications running on it. Essentially functioning as hidden translation layer, middleware enables communication and data management for distributed applications.
Which Redux middleware is best?
Redux thunk is the most popular middleware that allows you to call action creators, which then returns a function instead of an action object.
How do you call middleware in Redux?
To apply middleware, we can call this applyMiddleware() function in the createStore() method. In our src/redux/configureStore....The steps our API middleware will have to take:Find the request URL and compose request options from meta.Make the request.Convert the request to a JavaScript object.Respond back to Redux/user.
What is Redux promise middleware?
Redux Promise Middleware enables simple, yet robust handling of async action creators in Redux. const asyncAction = () => ({ type: 'PROMISE', payload: new Promise(...), })
Is Redux synchronous or asynchronous?
Redux is the state management library with asynchronous capabilities. In the old days, you could delegate asynchronous behavior through chained callbacks.
Why we use redux-thunk in Redux?
Redux Thunk middleware allows you to write action creators that return a function instead of an action. The thunk can be used to delay the dispatch of an action, or to dispatch only if a certain condition is met. The inner function receives the store methods dispatch and getState as parameters.
What is middleware in Redux?
In these frameworks, middleware is some code you can put between the framework receiving a request, and the framework generating a response. For example, Express or Koa middleware may add CORS headers, logging, compression, and more. The best feature of middleware is that it's composable in a chain. You can use multiple independent third-party middleware in a single project.
What are the benefits of redux?
One of the benefits of Redux is that it makes state changes predictable and transparent. Every time an action is dispatched, the new state is computed and saved. The state cannot change by itself, it can only change as a consequence of a specific action.
Can the second middleware be bound to the original dispatch function?
Then the second middleware will also be bound to the original dispatch function . But there's also a different way to enable chaining. The middleware could accept the next () dispatch function as a parameter instead of reading it from the store instance.
Can you use multiple third party middleware in a single project?
You can use multiple independent third-party middleware in a single project. Redux middleware solves different problems than Express or Koa middleware, but in a conceptually similar way. It provides a third-party extension point between dispatching an action, and the moment it reaches the reducer.
Does middleware take dispatch?
Now middleware takes the next () dispatch function, and returns a dispatch function, which in turn serves as next () to the middleware to the left, and so on. It's still useful to have access to some store methods like getState (), so store stays available as the top-level argument.
What does middleware do in redux?
Adding middleware gives us a place to take in the dispatch actions and lets us run the side effect after the action occurs. So our new redux pattern with middleware will look like : An event takes place. An action is dispatched. Middleware receives the action and runs the side effects. Reducer updates the state.
Why is middleware important?
Middleware is important because it makes synergy and integration across those applications possible. Now, let us look into where middleware in other industries and how it affects it.
Is middleware a tool?
At first, when using middleware in your applications might seem a little confusing but it makes up for a very powerful tool to handle side effects in your application and making you code handling faster and easier.
What Is Redux Middleware?
Redux Middleware allows you to intercept every action sent to the reducer so you can make changes to the action or cancel the action. Middleware helps you with logging, error reporting, making asynchronous requests, and a whole lot more.
How to Create Middleware in React
To create a middleware, we first need to import the applyMiddleware function from Redux like this:
Thanks for reading!
The content of this article is a small preview from my Mastering Redux course.
Why is Redux important?
Redux allows you to manage your app’s state in a single place and keep changes in your app more predictable and traceable. It makes it easier to reason about changes occurring in your app. But all of these benefits come with tradeoffs and constraints.
How does Redux work?
There is a central store that holds the entire state of the application. Each component can access the stored state without having to send down props from one component to another. There are three building parts: actions, store, and reducers. Let’s briefly discuss what each of them does.
What is redux in JavaScript?
Redux is a predictable state container designed to help you write JavaScript apps that behave consistently across client, server, and native environments and are easy to test. While it’s mostly used as a state management tool with React, you can use it with any other JavaScript framework or library.
What is server side rendering?
With it, you can handle the initial render of the app by sending the state of an app to the server along with its response to the server request. The required components are then rendered in HTML and sent to the clients.
What is redux debugging?
Redux makes it easy to debug an application. By logging actions and state, it is easy to understand coding errors, network errors, and other forms of bugs that might come up during production.
What is action in Redux?
Simply put, actions are events. They are the only way you can send data from your application to your Redux store. The data can be from user interactions, API calls, or even form submissions.
Why is the state of a redux always predictable?
In Redux, the state is always predictable. If the same state and action are passed to a reducer, the same result is always produced because reducers are pure functions. The state is also immutable and is never changed. This makes it possible to implement arduous tasks like infinite undo and redo.
What is a redux middleware function?
In this case, Redux middleware function provides a medium to interact with dispatched action before they reach the reducer. Customized middleware functions can be created by writing high order functions (a function that returns another function), which wraps around some logic.
What is reducer in Redux?
As discussed earlier, reducers are the place where all the execution logic is written. Reducer has nothing to do with who performs it, how much time it is taking or logging the state of the app before and after the action is dispatched. In this case, Redux middleware function provides a medium to interact with dispatched action before they reach ...
