
Important Points About Delegates:
- Provides a good way to encapsulate the methods.
- Delegates are the library class in System namespace.
- These are the type-safe pointer of any method.
- Delegates are mainly used in implementing the call-back methods and events.
- Delegates can be chained together as two or more methods can be called on a single event.
What is the use of delegate in C++?
Delegates are similar to C++ function pointers, but are type safe. Delegates allow methods to be passed as parameters. Delegates can be used to define callback methods. Delegates can be chained together; for example, multiple methods can be called on a single event. Methods don't need to match the delegate signature exactly.
When to use delegates in an event?
You can use the delegates if the caller of the method has no need to access any other methods, properties of the object. Lets discuss the point with the above code example. Caller (Publisher) in the above code is only interested in only one method of the Subscriber. The method is the handler (Container_MyEvent) of the event.
How do you declare a delegate in a class?
A delegate is a type that represents a method with a specific signature and return type. The declaration of a delegate looks exactly like the declaration of a method, except with the keyword delegate in front of it. Just like classes and interfaces, we can declare delegates outside of classes or nested within classes.
What is the use of delegate in Salesforce?
Delegates are mainly used in implementing the call-back methods and events. Delegates can be chained together as two or more methods can be called on a single event. It doesn’t care about the class of the object that it references.

When should we use delegate?
When to use delegates?These are used to represent or refer to one or more functions.These can only be used to define call-back methods.In order to consume a delegate, we need to create an object to delegate.
What are the benefits of delegates in C#?
Advantages to using them in design: Allow you to develop libraries and classes that are easily extensible, since it provides an easy way to hook in other functionality (for example, a where clause in LINQ can use a delegate [Func
What is the delegate and its benefits Why do we need C# delegates?
Delegates allow methods to be passed as parameters. Delegates are type safe function pointer. Delegate instances attach or detach a method at run time making it more dynamic and flexible to use. Delegates can invoke more than one method using the Multicast feature.
What is Delegation in C?
C# delegates are similar to pointers to functions, in C or C++. A delegate is a reference type variable that holds the reference to a method. The reference can be changed at runtime. Delegates are especially used for implementing events and the call-back methods. All delegates are implicitly derived from the System.
What is advantage of delegate?
Benefits of Delegating Gives you the time and ability to focus on higher-level tasks. Gives others the ability to learn and develop new skills. Develops trust between workers and improves communication. Improves efficiency, productivity, and time management.
Is delegate a callback?
This callback can be synchronous or asynchronous. So, in this way large piece of the internal behavior of a method from the outside of a method can be controlled. It is basically a function pointer that is being passed into another function. Delegate is a famous way to implement Callback in C#.
What is a delegate in Objective-C?
An Objective-C delegate is an object that has been assigned to the delegate property another object. To create one, you define a class that implements the delegate methods you're interested in, and mark that class as implementing the delegate protocol.
What is delegation work?
Delegation refers to the transfer of responsibility for specific tasks from one person to another. From a management perspective, delegation occurs when a manager assigns specific tasks to their employees.
Can delegates return type?
delegate: It is the keyword which is used to define the delegate. return_type: It is the type of value returned by the methods which the delegate will be going to call. It can be void. A method must have the same return type as the delegate.
What is a delegate in computer?
Delegation (computer security), one user or process allowing another user or process to use their credentials or permissions. Delegate (CLI), a form of type-safe function pointer used by the Common Language Infrastructure (CLI), specifying both a method to call and optionally an object to call the method on.
Why delegates are type-safe?
Delegates are not type-safe. Delegate is a user-defined type. Only one method can be bound with one delegate object. Delegates can be used to implement callback notification.
What is delegates and its types?
Definition. A delegate(known as function pointer in C/C++) is a references type that invokes single/multiple method(s) through the delegate instance. It holds a reference of the methods. Delegate types are sealed and immutable type.
What is difference between event and delegate?
A delegate specifies a TYPE (such as a class , or an interface does), whereas an event is just a kind of MEMBER (such as fields, properties, etc). And, just like any other kind of member an event also has a type. Yet, in the case of an event, the type of the event must be specified by a delegate.
What are the types of delegates in C#?
What are types of delegates in C#? There are two types of delegates, singlecast delegates, and multiplecast delegates. Singlecast delegate point to single method at a time. In this the delegate is assigned to a single method at a time.
What is delegate in C# with real time example?
It is a reference type. It is a function pointer or it holds a reference (pointer) to a function (method). It is type safe. Delegates are mainly used for the event handling and the callback methods.
Can delegate be static in C#?
Static delegates are not without limitations. They can only refer to static functions; member methods on objects are not permitted because there is no place to store the pointer to the object. Furthermore, static delegates cannot be chained to other delegates.
How to use delegate in C#?
Use and Need of Delegates in C# 1 An eventing design pattern is used. 2 It is desirable to encapsulate a static method. 3 The caller has no need to access other properties, methods, or interfaces on the object implementing the method. 4 Easy composition is desired. 5 A class may need more than one implementation of the method.
Can a caller access other properties?
The caller has no need to access other properties, methods, or interfaces on the object implementing the method. You can use the delegates if the caller of the method has no need to access any other methods, properties of the object. Lets discuss the point with the above code example.
My Experience with Delegates
In my all years of experience I have used delegate several times and noticed that after I am done with development who ever over takes that code from me is not able to grasp that particular delegate logic.
Purpose
Invoke is the method which takes Action<TService> type argument, in .Net Action delegate's return type is void.
What is a delegate in C#?
A delegate is an object which refers to a method or you can say it is a reference type variable that can hold a reference to the methods. Delegates in C# are similar to the function pointer in C/C++. It provides a way which tells which method is to be called when an event is triggered. For example, if you click an Button on a form (Windows Form ...
What happens after declaring a delegate?
After declaring a delegate, a delegate object is created with the help of new keyword. Once a delegate is instantiated, a method call made to the delegate is pass by the delegate to that method.
What is multicasting delegate?
Multicasting of delegate is an extension of the normal delegate (sometimes termed as Single Cast Delegate). It helps the user to point more than one method in a single call.
Can delegate methods be chained together?
Delegates can be chained together as two or more methods can be called on a single event. It doesn’t care about the class of the object that it references. Delegates can also be used in “anonymous methods” invocation. Anonymous Methods (C# 2.0) and Lambda expressions (C# 3.0) are compiled to delegate types in certain contexts.
Does multicasting of delegate throw a runtime exception?
Note: Remember, multicasting of delegate should have a return type of Void otherwise it will throw a runtime exception. Also, the multicasting of delegate will return the value only from the last method added in the multicast. Although, the other methods will be executed successfully.
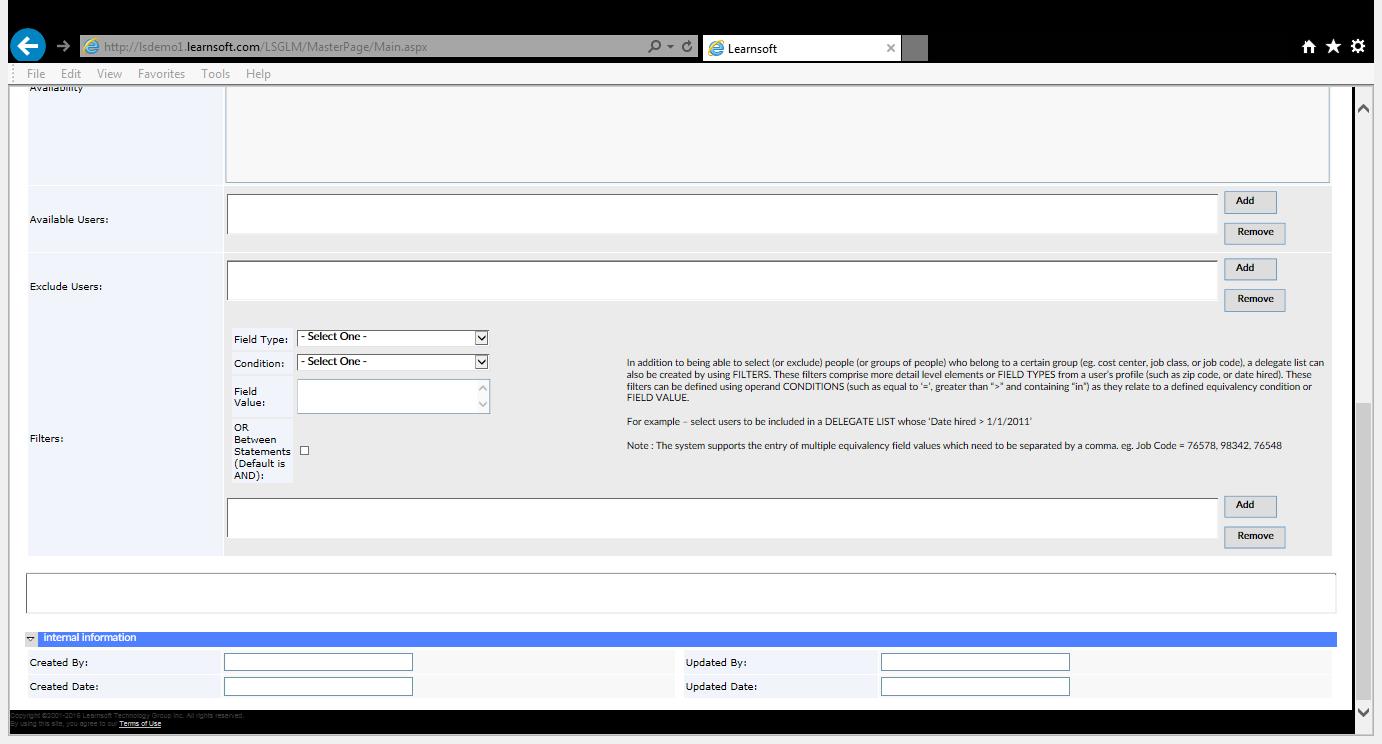