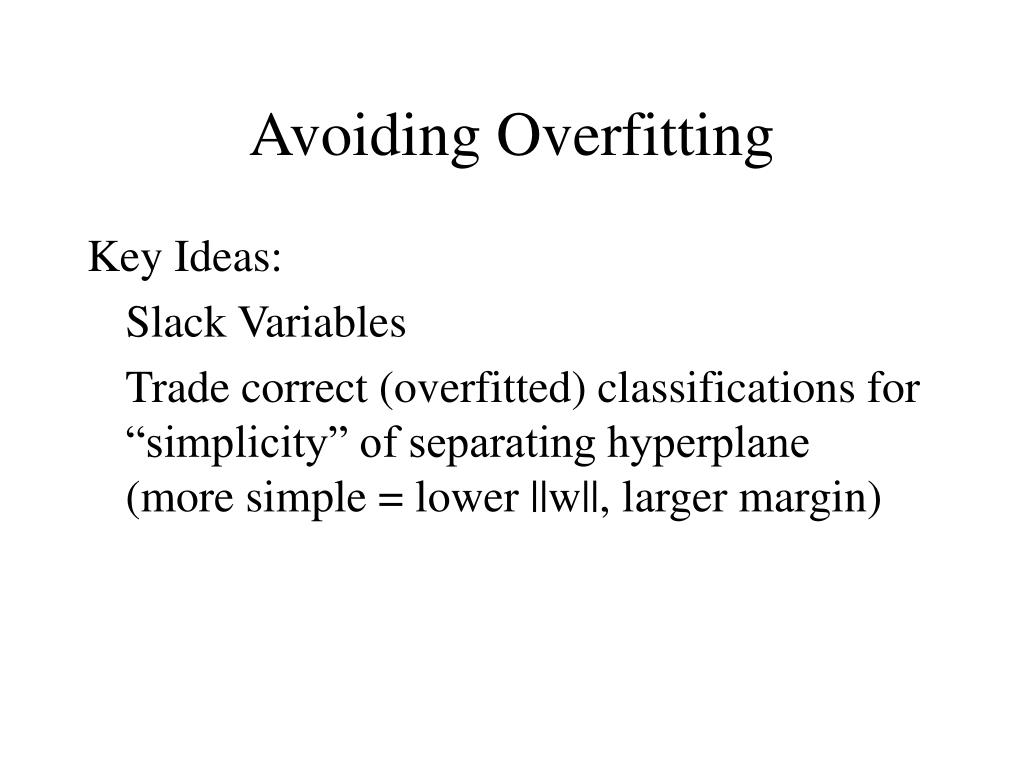
Why static methods Cannot be overridden?
Overloading is the mechanism of binding the method call with the method body dynamically based on the parameters passed to the method call. Static methods are bonded at compile time using static binding. Therefore, we cannot override static methods in Java.
Can static methods be overloaded or overridden?
Can we overload static methods? The answer is 'Yes'. We can have two or more static methods with the same name, but differences in input parameters.
Can static and private methods be overridden?
You cannot override a private or static method in Java. If you create a similar method with same return type and same method arguments in child class then it will hide the super class method; this is known as method hiding. Similarly, you cannot override a private method in sub class because it's not accessible there.
Can we override static method in subclass?
The static method is resolved at compile time cannot be overridden by a subclass. An instance method is resolved at runtime can be overridden. A static method can be overloaded.
Why main method is static?
The main() method is static so that JVM can invoke it without instantiating the class. This also saves the unnecessary wastage of memory which would have been used by the object declared only for calling the main() method by the JVM.
Can we override final method?
Can We Override a Final Method? No, the Methods that are declared as final cannot be Overridden or hidden. For this very reason, a method must be declared as final only when we're sure that it is complete.
Can you override an abstract method?
A subclass must override all abstract methods of an abstract class. However, if the subclass is declared abstract, it's not mandatory to override abstract methods.
Can we override inner class?
No, you cannot override private methods in Java, private methods are non-virtual in Java and access differently than non-private one. Since method overriding can only be done on derived class and private methods are not accessible in a subclass, you just can not override them.
Can constructor be private?
A private constructor in Java is used in restricting object creation. It is a special instance constructor used in static member-only classes. If a constructor is declared as private, then its objects are only accessible from within the declared class. You cannot access its objects from outside the constructor class.
Can we overload the main () method?
Yes, We can overload the main method in java but JVM only calls the original main method, it will never call our overloaded main method.
Can constructor be overridden?
It does not have a return type and its name is same as the class name. But, a constructor cannot be overridden. If you try to write a super class's constructor in the sub class compiler treats it as a method and expects a return type and generates a compile time error.
Can we inherit static method in Java?
Static methods do not use any instance variables of any object of the class they are defined in. Static methods take all the data from parameters and compute something from those parameters, with no reference to variables. We can inherit static methods in Java.
Can we overload static main method in Java?
No, we cannot override main method of java because a static method cannot be overridden. The static method in java is associated with class whereas the non-static method is associated with an object.
Which method Cannot be overridden?
A method declared final cannot be overridden. A method declared static cannot be overridden but can be re-declared. If a method cannot be inherited, then it cannot be overridden. A subclass within the same package as the instance's superclass can override any superclass method that is not declared private or final.
Can we overload static and non static method together?
Yes they can overload each other.
When static methods are loaded in Java?
Static Block in Java It executes whenever the class is loaded in memory. One class can have numerous static blocks, which will be executed in the same sequence in which they are written.
Why use static methods?
Some reasons to use static methods: 1 They are a little bit faster than instance methods. Also see this msdn article which gives performance numbers to back this up (inlined static call avg 0.2 ns, static call avg 6.1ns, inlined instance call avg 1.1 ns, instance call avg 6.8 ns) 2 Less verbose to write out - don't need to instantiate a class to get to them (and instantiation can also affect performance)
What does overriding methods mean?
Mostly overriding methods means you reference a base type and want to call a derived method. Since static's are part of the type and aren't subject to vtable lookups that doesn't make sense. Because statics don't work on instances, you always specify Foo.Bar or Bar.Bar explicitly.
What is static void main?
Another example is 'static void Main', which is the main access point in your program. Basically you use them whenever you don't want or cannot create an object instance before using it. For example, when the static method creates the object.
Do static types have a contract?
This is an important thing to understand: when types inherit from other types, they fulfil a common contract, whereas static types are not bound by any contract (from the pure OOP point of view). There's no technical way in the language to tie two static types together with an "inheritance" contract.
Does static method make sense?
If you think about overriding static methods it, it doesn't really make sense; in order to have virtual dispatch you need an actual instance of an object to check against.
Do static types inherit from other types?
This is an important thing to understand: when types inherit from other types, they fulfil a common contract, whereas static types are not bound by any contract (from the pure OOP point of view). There's no technical way in the language to tie two static types together with an "inheritance" contract. If you would "override" the Log method in two different places.
Can you make a class static?
Note that if you make the classes static, you cannot do this. Static classes have to derive from object. The main difference between this and inheritance is that the compiler can determine at compile-time which method to call when using static.