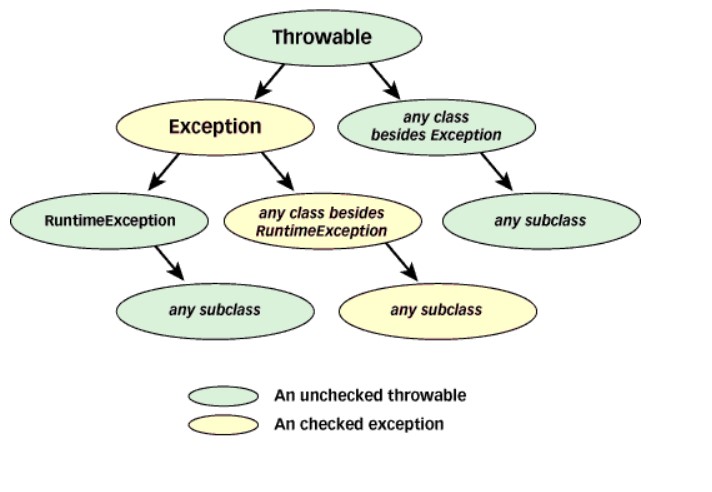
Hence, by not checking for such exceptions at the compile time, the Java compiler does not ask us to handle the unchecked exceptions. Some important points about unchecked exceptions Unlike checked exceptions, possibility of the occurrence of unchecked exceptions are never notified to us at the compile time in the form of compile-time errors.
How to create a custom Unchecked exception in Java?
Java - Creating Custom Exception
- Overview In this article, We'll learn how to create custom exceptions in java. ...
- Understand the need for custom exceptions Java comes with a set of predefined exceptions that cover all or common scenarios. ...
- Java - Custom Checked Exceptions Checked exceptions are one type of exception and there has to be handled by the code explicitly. ...
What is difference between a checked and Unchecked exception?
the difference between checked and unchecked exception is that checked exceptions are those that must be caught and handled by the checks () statement or they will cause a program to terminate and print a stack trace, whereas, unchecked exceptions, on the other hand, should not need to be caught or handled at all, as it is the programmer’s …
Why does Java have checked exceptions?
Why it's so Confusing
- Historical Perspective. Back in the time of the “C” programming language, it was customary to return values such as -1 or NULL from functions to indicate errors.
- Exception Handling in Java. So what is exception handling? ...
- Two Types of Exceptions in Java: Checked and Unchecked. ...
- Checked vs Unchecked Exceptions. ...
- The Java Exception Class Hierarchy. ...
What does Unchecked exception mean in Java?
What is throwing an exception Java?
- throws. The caller must catch the exceptions. Suppose in your java program you using a library method which throws an Exception.
- Throws
- in the. What is throw in exception handling? Only object of Throwable class or its sub classes can be thrown.

Is it good practice to handle unchecked exceptions?
Programmers should properly handle unchecked exceptions when they are expected and are not caused by programming errors.
Can you catch unchecked exceptions?
Yes, you can throw unchecked exceptions with throw . And yes, you can catch unchecked exceptions in a catch block. You can also propagate them with throws explicitly, which is optional, but can make things clearer in the method signature.
Can we use throws for unchecked exception in Java?
The throw keyword in Java is used for explicitly throwing a single exception. This can be from within a method or any block of code. Both checked and unchecked exceptions can be thrown using the throw keyword.
Can we override unchecked exception?
No, you cannot.
Can we handle runtime exception in Java?
Generally the point of a RuntimeException is that you can't handle it gracefully, and they are not expected to be thrown during normal execution of your program.
Can we throw runtime exception?
RunTimeException is an unchecked exception. You can throw it, but you don't necessarily have to, unless you want to explicitly specify to the user of your API that this method can throw an unchecked exception.
Should we handle runtime exceptions?
Having to add runtime exceptions in every method declaration would reduce a program's clarity. Thus, the compiler does not require that you catch or specify runtime exceptions (although you can). One case where it is common practice to throw a RuntimeException is when the user calls a method incorrectly.
Is IOException checked or unchecked?
checked exceptionBecause IOException is a checked exception type, thrown instances of this exception must be handled in the method where they are thrown or be declared to be handled further up the method-call stack by appending a throws clause to each affected method's header.
Can we extend checked exception instead runtime exception?
If you want to write a checked exception that is automatically enforced by the Handle or Declare Rule, you need to extend the Exception class. If you want to write a runtime exception, you need to extend the RuntimeException class.
Can we override static method?
Static methods are bonded at compile time using static binding. Therefore, we cannot override static methods in Java.
Can we throw checked exception in child class?
We need to follow some rules when we overriding a method that throws an Exception. When the parent class method doesn't throw any exceptions, the child class method can't throw any checked exception, but it may throw any unchecked exceptions.
What happen if we override super class static method?
No, we cannot override static methods because method overriding is based on dynamic binding at runtime and the static methods are bonded using static binding at compile time. So, we cannot override static methods. The calling of method depends upon the type of object that calls the static method.
Checked Exceptions in Java
In broad terms, a checked exception (also called a logical exception) in Java is something that has gone wrong in your code and is potentially recoverable. For example, if there’s a client error when calling another API, we could retry from that exception and see if the API is back up and running the second time.
Checked Exception Examples
The code below shows the FileInputStream method from the java.io package with a red line underneath. The red line is because this method throws a checked exception and the compiler is forcing us to handle it. You can do this in one of two ways.
Unchecked Exceptions in Java
An unchecked exception (also known as an runtime exception) in Java is something that has gone wrong with the program and is unrecoverable. Just because this is not a compile time exception, meaning you do not need to handle it, that does not mean you don’t need to be concerned about it.
Unchecked Exception Examples
Because we live in a world where systems are built from lots of small micro services doing their own thing all talking to each other, generally over HTTP, this exception is popping up more and more. There isn’t a lot you can do about it other than find a free port.
Checked Exceptions During Runtime
Below is an example that is very commonly used in micro service architecture. If we received a request and we cannot, say, read data from our database needed for this request, the database will throw us a checked exception, maybe an SQLException or something similar. Because this data is important, we cannot fulfil this request without it.
Difference Between Checked and Unchecked Exceptions in Java
To summarize, the difference between a checked and unchecked exception is:
Track, Analyze and Manage Java Errors With Rollbar
Managing errors and exceptions in your code is challenging. It can make deploying production code an unnerving experience. Being able to track, analyze, and manage errors in real-time can help you to proceed with more confidence. Rollbar automates Java error monitoring and triaging, making fixing errors easier than ever. Try it Today!
What is the difference between unchecked and checked exceptions?
The only difference between checked and unchecked exceptions is that checked ones have to be either caught or declared in the method signature using throws, whereas with unchecked ones this is optional.
What does it mean when an exception is checked?
If an exception is a checked exception, the compiler will check that your code either throws the exception or handles it in a try/catch block at compile-time. For unchecked exceptions, the compiler won't do such a check. You can handle checked/unchecked exceptions the same way (with try/catch/throws), the difference just lies in the checks ...
Is an exception checked or unchecked?
If a client cannot do anything to recover from the exception, then it's ok to have it as an unchecked exception.
What are some common checked exceptions in Java?
Some common checked exceptions in Java are IOException, SQLException, and ParseException. The Exception class is the superclass of checked exceptions. Therefore, we can create a custom checked exception by extending Exception: 3.
Why do we use exceptions in Java?
It's a good practice to use exceptions in Java so that we can separate error-handling code from regular code. However, we need to decide which type of exception to throw. The Oracle Java Documentation provides guidance on when to use checked exceptions and unchecked exceptions:
What happens if a program throws an unchecked exception?
If a program throws an unchecked exception, it reflects some error inside the program logic. For example, if we divide a number by 0, Java will throw ArithmeticException: Java does not verify unchecked exceptions at compile-time. Furtheremore, we don't have to declare unchecked exceptions in a method with the throws keyword.
Does Java check unchecked exceptions?
Java does not verify unchecked exceptions at compile-time. Furtheremore, we don't have to declare unchecked exceptions in a method with the throws keyword. And although the above code does not have any errors during compile-time, it will throw ArithmeticException at runtime. Some common unchecked exceptions in Java are NullPointerException, ...
Can you use a try catch block to handle a checked exception?
We can also use a try-catch block to handle a checked exception: Some common checked exceptions in Java are IOException, SQLException, and ParseException. The Exception class is the superclass of checked exceptions. Therefore, we can create a custom checked exception by extending Exception: 3. Unchecked Exceptions.
What are Unchecked Exceptions?
"Unchecked Exceptions" are named so because they are left unchecked by the Java Compiler at the compile time of a program. Hence, by not checking for such exceptions at the compile time, the Java compiler does not ask us to handle the unchecked exceptions.
Some important points about unchecked exceptions
Unlike checked exceptions, possibility of the occurrence of unchecked exceptions are never notified to us at the compile time in the form of compile-time errors.
Output
Here in this code above, an integer (100) is divided by a zero value. Division of an integer by zero is an illegal operation in Java and it leads to an unchecked exception of type ArithmeticException.
What does exception mean in Java?
Dictionary Meaning: Exception is an abnormal condition. In Java, an exception is an event that disrupts the normal flow of the program. It is an object which is thrown at runtime.
Why is exception handling important?
The core advantage of exception handling is to maintain the normal flow of the application. An exception normally disrupts the normal flow of the application that is why we use exception handling. Let's take a scenario:
What are the advantages of exception handling?
The core advantage of exception handling is to maintain the normal flow of the application. An exception normally disrupts the normal flow of the application; that is why we need to handle exceptions. Let's consider a scenario: 1 statement 1; 2 statement 2; 3 statement 3; 4 statement 4; 5 statement 5; 6 statement 6; 7 statement 7; 8 statement 8; 9 statement 9; 10 statement 10;
What is the use of the "try" keyword in Java?
There are 5 keywords which are used in handling exceptions in Java. The "try" keyword is used to specify a block where we should place exception code. The try block must be followed by either catch or finally. It means, we can't use try block alone. The "catch" block is used to handle the exception.
What is the final block in Java?
The "finally" block is used to execute the important code of the program. It is executed whether an exception is handled or not. The "throw" keyword is used to throw an exception. The "throws" keyword is used to declare exceptions.
What does "finally" mean in Java?
The "catch" block is used to handle the exception. It must be preceded by try block which means we can't use catch block alone. It can be followed by finally block later. finally. The "finally" block is used to execute the important code of the program.
What is a checked exception in Java?
Checked vs Unchecked Exceptions in Java. In Java, there are two types of exceptions: 1) Checked: are the exceptions that are checked at compile time. If some code within a method throws a checked exception, then the method must either handle the exception or it must specify the exception using throws keyword. ...
Should we make exceptions checked or unchecked?
If a client cannot do anything to recover from the exception, make it an unchecked exception.
Is an exception unchecked in C++?
In C++, all exceptions are unchecked, so it is not forced by the compiler to either handle or specify the exception. It is up to the programmers to be civilized, and specify or catch the exceptions. In Java exceptions under Error and RuntimeException classes are unchecked exceptions, everything else under throwable is checked. ...
