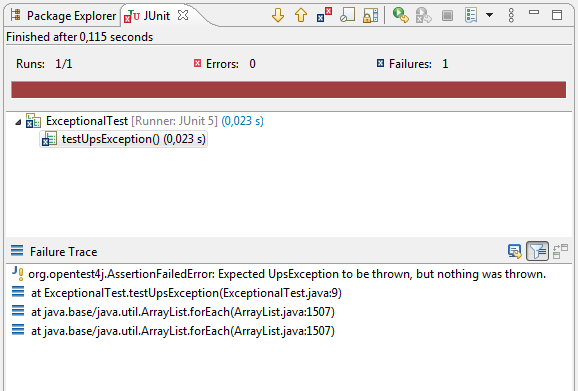
Testing for expected exceptions in JUnit
- 1) @Test (expected…) The @Test annotation has an optional parameter, “expected”, that allows you to specify a subclass of Throwable. If we wanted to verify that canVote () () method above throws the correct exception, we would write: ...
- 2) ExpectedException To use JUnit’s ExpectedException, first you need to declare the ExpectedException: ...
- 3) Try/catch with assert/fail
How do you test for exceptions In JUnit?
JUnit - Exceptions Test. JUnit provides an option of tracing the exception handling of code. You can test whether the code throws a desired exception or not. The expected parameter is used along with @Test annotation.
What happens if the expected exception is thrown in a test?
If the expected exception ( IllegalArgumentException in this example) is thrown, the test succeeded, otherwise it fails. You can see the above code uses an anonymous class of type Executable. Of course you can shorter the code with Lambda syntax:
Why does my JUnit test fail when I try to unit test?
This is because you are expecting an exception from the method you are Unit Testing, otherwise our JUnit test would fail. Example@Test (expected=IllegalArgumentException.class)
What is the expected parameter in exception testing in Java?
By using “expected” parameter, you can specify the exception name our test may throw. In above example, you are using “ IllegalArgumentException” which will be thrown by the test if a developer uses an argument which is not permitted. Let’s understand exception testing by creating a Java class with a method throwing an exception.
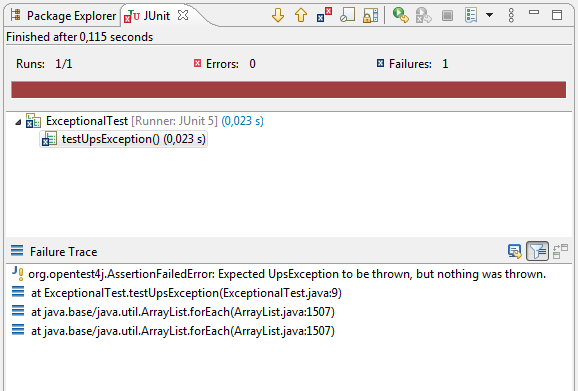
How do I expect an exception in JUnit 5?
In JUnit 5, to write the test code that is expected to throw an exception, we should use Assertions. assertThrows(). In the given test, the test code is expected to throw an exception of type ApplicationException or its subtype. Note that in JUnit 4, we needed to use @Test(expected = NullPointerException.
How do you test exception handling in JUnit?
Create a java class file named TestRunner. java in C:\>JUNIT_WORKSPACE to execute test case(s). Compile the MessageUtil, Test case and Test Runner classes using javac. Now run the Test Runner, which will run the test cases defined in the provided Test Case class.
How do you assert an exception?
1. JUnit5 – assertThrows@Test. void assertNullPointerException() { var message = "test exception"; var expectedException = ... assertThrows( RuntimeException. class, ... @Test(expected = NullPointerException. class) void assertNullPointerException() { ... @Rule. public ExpectedException expectedException = ExpectedException. none();
How do I expect NullPointerException in JUnit?
JUnit 4. Using @Test Expected Attributepackage myunittests;import org. junit. Test;public class ExpectingExceptionTest {@Test(expected = NullPointerException. class)public void testNullPointerException(){String name = getName();System. out. println(name. length());More items...•
How do you throw an exception in a test case?
In order to test the exception thrown by any method in JUnit 4, you need to use @Test(expected=IllegalArgumentException. class) annotation. You can replace IllegalArgumentException. class with any other exception e.g. NullPointerException.
What is exception testing?
Exception testing is a special feature introduced in JUnit4. In this tutorial, you have learned how to test exception in JUnit using @test(excepted) Junit provides the facility to trace the exception and also to check whether the code is throwing exception or not.
How do you expect an exception in JUnit 4?
When using JUnit 4, we can simply use the expected attribute of the @Test annotation to declare that we expect an exception to be thrown anywhere in the annotated test method. In this example, we've declared that we're expecting our test code to result in a NullPointerException.
How do you write JUnit test cases for custom exception class?
In JUnit, there are 3 ways to test the expected exceptions : @Test , optional 'expected' attribute....1. @ Test expected attribute. ... Try-catch and always fail() This is a bit old school, widely used in JUnit 3. ... 3. @ Rule ExpectedException.
Can we catch assertion error?
In order to catch the assertion error, we need to declare the assertion statement in the try block with the second expression being the message to be displayed and catch the assertion error in the catch block.
How do you cause NullPointerException?
What Causes NullPointerExceptionCalling methods on a null object.Accessing a null object's properties.Accessing an index element (like in an array) of a null object.Passing null parameters to a method.Incorrect configuration for dependency injection frameworks like Spring.Using synchronized on a null object.More items...•
How do you assert null in JUnit?
Assert class in case of JUnit 4 or JUnit 3 to assert using assertNull method. Assertions. assertNull() checks that object is null. In case, object is not null, it will through AssertError.
Why do we get NullPointerException?
NullPointerException is thrown when program attempts to use an object reference that has the null value. These can be: Invoking a method from a null object. Accessing or modifying a null object's field.
How do you write JUnit test cases for custom exceptions in java?
“how to test custom exception class junit 5” Code [email protected] testExpectedException() {Assertions. assertThrows(NumberFormatException. class, () -> {Integer. parseInt("One");});}More items...•
How do you handle exceptions in Mockito?
ExampleStep 1 − Create an interface called CalculatorService to provide mathematical functions.Step 2 − Create a JAVA class to represent MathApplication.Step 3 − Test the MathApplication class. ... Step 4 − Execute test cases. ... Step 5 − Verify the Result.
What happens if the executable code does not throw an exception?
message – If the executable code does not throw any exception, this message will be printed along with FAIL result.
What happens if no exception is thrown from the executable block?
If no exception is thrown from the executable block then assertThrows () will FAIL.
Why does the test pass if we are expecting an illegalArgumentException?
For example, if we are expecting IllegalArgumentException and the test throws NumberFormatException then also the test will PASS because NumberFormatException extends IllegalArgumentException class.
Example
import com.w3spoint.business.*; import static org. junit. Assert. *; import org.junit.Test; /** * This is test case class for division method.
Output
Download this example. Next Topic: Junit ignore test. Previous Topic: Junit basic annotation example.
How to declare an expected exception in a test case?
In your test case you declare an ExpectedException annotated with @Rule, and assign it a default value of ExpectedException.none (). Then in your test that expects an exception you replace the value with the actual expected value. The advantage of this is that without using the ugly try/catch method, you can further specify what the message within the exception was
What is the advantage of ExpectedException?
ADDITION Another advantage of using ExpectedException is that you can more precisely scope the exception within the context of the test case.
Can you throw an exception if the gold isn't greater than or equal to zero?
I wouldn't throw an Exception if the gold isn't greater than or equal to zero. I would throw an IllegalArgumentException. It certainly sounds like it's illegal your Pirate to have a negative amount of gold.
Can you use expected in @test annotation?
You could either use expected in @Test annotation or provide an explicit catch block and issue a fail if the program flow is not as expected.
Can you test for generic exceptions?
Using this method, you might be able to test for the message in the generic exception to be something specific.
Does JUnit 5 support expected exceptions?
They are replaced with the new assertThrows (), which requires the use of Java 8 and lambda syntax. ExpectedException is still available for use in JUnit 5 through JUnit Vintage. Also JUnit Jupiter will also continue to support JUnit 4 ExpectedException through use of the junit-jupiter-migrationsupport module, but only if you add an additional class-level annotation of @EnableRuleMigrationSupport.
What happens if an expected exception is thrown?
If the expected exception ( IllegalArgumentException in this example) is thrown, the test succeeded, otherwise it fails. You can see the above code uses an anonymous class of type Executable. Of course you can shorter the code with Lambda syntax:
Can you use try catch in JUnit?
In JUnit 3, or more exactly , in any versions of JUnit you can always use Java’s try-catch structure to test exception. Here’s an example:
What is junit testing?
Junit is a unit testing framework for the Java programming language. If you want to read about best practices followed for junit testing then here is an excellent guide for your reference.
Can you throw the same exception twice in the same flow?
If the unit under test can throw the same exception twice in the same flow (e.g. NullpointerExceptions can usually be thrown almost enywhere). Then you don’t know which exception it was that caused the test to pass.
When using JUnit 4, can we use the expected attribute of the @Test annotation?
When using JUnit 4, we can simply use the expected attribute of the @Test annotation to declare that we expect an exception to be thrown anywhere in the annotated test method.
What happens if you pass an exception as the expected exception type?
This means that if we pass Exception as the expected exception type, any exception thrown will make the assertion succeed since Exception is the super-type for all exceptions. If we change the test above to expect a RuntimeException, this will also pass:
Create a Class
Create a java class to be tested, say, MessageUtil.java in C:\> JUNIT_WORKSPACE.
Create Test Case Class
Create a java test class called TestJunit.java. Add an expected exception ArithmeticException to the testPrintMessage () test case.
Create Test Runner Class
Create a java class file named TestRunner.java in C:\>JUNIT_WORKSPACE to execute test case (s).
