How to test Exception in JUnit?
- Step 1: Open Eclipse > File > New > Maven Project.
- Step 2: Now, you will get a New Maven Project. ...
- Step 3: Now, Enter group id, artifact id as shown below
- Step 4: First, create our JUnit Test case. ...
- Step 5: Now, we will get the default test case generated and we will modify the code as shown below ...
...
With annotation
- Error messages when the code does not throw an exception are automagically handled.
- The readability is improved.
- There is less code to be created.
What is exception test In JUnit?
JUnit - Exceptions Test. JUnit provides an option of tracing the exception handling of code. You can test whether the code throws a desired exception or not.
What is expectedexception and @rule annotation in JUnit?
We will explore this a bit further down with JUnit's ExpectedException and @Rule annotation. JUnit responded back to the users need for exception handling by adding a @Test annotation field "expected". The intention is that the entire test case will pass if the type of exception thrown matched the exception class present in the annotation.
What does @JUnit expect failing tests to do?
JUnit expects failing tests will throw Exceptions, your catching them is just stopping JUnit from being able to report them properly. Also this way the expected property on the @Test annotation will work.
How do you test an exception in test cases?
Testing exceptions is common in automation testing, in unit testing or in testing negative test cases. For example, you try to open a non-existent file with the application and check if it throws or handles the exception case. ( FileNotFoundException) We use the clause @Test (expected=<exception class>) to test the method.
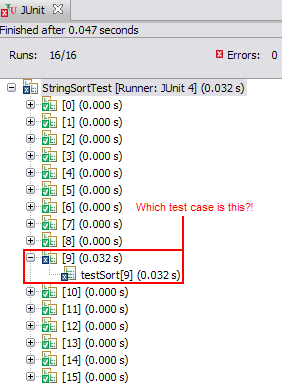
How do you handle exceptions in testing?
Following are the steps involved in the error handling testing:Test Environment Set Up: Test environment is set according to the software testing technique so that the testing process can run smoothly. ... Test Case Generation: ... Test Case Execution: ... Result and Analysis: ... Re-test:
How do you write test cases for exceptions in JUnit 5?
In JUnit 5, to write the test code that is expected to throw an exception, we should use Assertions. assertThrows(). In the given test, the test code is expected to throw an exception of type ApplicationException or its subtype. Note that in JUnit 4, we needed to use @Test(expected = NullPointerException.
How do you handle exceptions in assert?
In order to handle the assertion error, we need to declare the assertion statement in the try block and catch the assertion error in the catch block.
How do you write unit tests for exceptions?
0:167:535 JUnit5 - Writing Unit Test For Exceptions - YouTubeYouTubeStart of suggested clipEnd of suggested clipSo if it if n if the number is less than minus 100 it is going to throw an exception. It's. So runMoreSo if it if n if the number is less than minus 100 it is going to throw an exception. It's. So run tiny exception. But is the exception that is given by the JDK.
How do I fix a NullPointerException in JUnit?
NullPointerException is thrown when a reference variable is accessed (or de-referenced) and is not pointing to any object. This error can be resolved by using a try-catch block or an if-else condition to check if a reference variable is null before dereferencing it.
What happens if exceptions are not handled?
When an exception occurred, if you don't handle it, the program terminates abruptly and the code past the line that caused the exception will not get executed.
Can we catch assertion error?
In order to catch the assertion error, we need to declare the assertion statement in the try block with the second expression being the message to be displayed and catch the assertion error in the catch block.
How do I assert logs in JUnit?
Asserting Log Messages With JUnitIntroduction. In this tutorial, we'll look at how we can cover generated logs in JUnit testing. ... Maven Dependencies. Before we begin, let's add the logback dependency. ... A Basic Business Function. ... Testing the Logs. ... Conclusion.
How do you write JUnit test cases for custom exceptions in Java?
“how to test custom exception class junit 5” Code [email protected] testExpectedException() {Assertions. assertThrows(NumberFormatException. class, () -> {Integer. parseInt("One");});}More items...•
How do you throw an exception in JUnit?
When using JUnit 4, we can simply use the expected attribute of the @Test annotation to declare that we expect an exception to be thrown anywhere in the annotated test method. In this example, we've declared that we're expecting our test code to result in a NullPointerException.
How do you handle and verify exceptions in junit5?
To verify the fields of an exception you'd have to add a try/catch within the test case, and within the catch block perform the additional assertions and then throw the caught exception. When using ExpectedException you have to initially declare it with none() , no exception expected, which is a bit confusing.
What is exception testing?
Exception testing is a special feature introduced in JUnit4. In this tutorial, you have learned how to test exception in JUnit using @test(excepted) Junit provides the facility to trace the exception and also to check whether the code is throwing exception or not.
Create a Class
Create a java class to be tested, say, MessageUtil.java in C:\> JUNIT_WORKSPACE.
Create Test Case Class
Create a java test class called TestJunit.java. Add an expected exception ArithmeticException to the testPrintMessage () test case.
Create Test Runner Class
Create a java class file named TestRunner.java in C:\>JUNIT_WORKSPACE to execute test case (s).
Overview
Let’s learn the steps involved in Testing exceptions using JUnit framework. In this post, we will learn how to test methods that throw exceptions in the code. Testing exceptions is common in automation testing, in unit testing or in testing negative test cases.
JUnit listing
package com.testingdocs.junit; /** * A sample JUnit4 Test demo to test exceptions */ /** * @author testingdocs * */ import static org.junit.Assert.*; import org.junit.Test; public class JUnit4ExceptionTest { @Test (expected=Exception.class) public void exceptionTest () throws Exception { //This test will pass as the method code throws // an exception.
Exercise
Run the below code and fill in the blank. You can neglect the package declaration.
How to handle exceptions in JUnit?
In JUnit there are 3 popular ways of handling exceptions in your test code: 1 try-catch idiom 2 With JUnit rule 3 With annotation
What happens when an exception isn't thrown?
When the exception isn’t thrown you will get the following message: java.lang.AssertionError: Expected test to throw (an instance of java.lang.IllegalArgumentException and exception with message “negatives not allowed: [-1, -2]”). Pretty nice.
Is it bad to throw an exception in a test?
Sometimes it is tempting to expect general Exception, RuntimeException or even a Throwable. And this is considered as a bad practice, because your code may throw exception in other place than you actually expected and your test will still pass!
Can you use mockito with JUnit?
Actually the solution seems nice at first glance, but it requires your own JUnit runner hence it has disadvantage: you cannot use this annotation with e.g. Mockito runner.
What happens if an expected exception is thrown?
If the expected exception ( IllegalArgumentException in this example) is thrown, the test succeeded, otherwise it fails. You can see the above code uses an anonymous class of type Executable. Of course you can shorter the code with Lambda syntax:
Can you use try catch in JUnit?
In JUnit 3, or more exactly , in any versions of JUnit you can always use Java’s try-catch structure to test exception. Here’s an example:
What happens when an exception isn't thrown?
When the exception isn't thrown you will get the following message: java.lang.AssertionError: Expected test to throw (an instance of java.lang.IllegalArgumentException and exception with message "negatives not allowed: [-1, -2]"). Pretty nice.
What is a catch exception?
In short, catch-exception is a library that catches exceptions in a single line of code and makes them available for further analysis.
What is @RequestBody annotation?
In Spring MVC the @RequestBody annotation indicates a method parameter should be bound to a body of the request. @RequestBody parameter can treated as any other parameter in a @RequestMapping method and therefore it can also be validated by a standard validation mechanism. In this post I will show 3 ways of validating the @RequestBody parameter in your Spring MVC application.
Can you test exceptions in Java 8?
With just couple of lines of code, one can build quite cool code for testing exceptions in JUnit without any additional library. See: JUnit: testing exception with Java 8 and Lambda Expressions
Can you use mockito with JUnit?
Actually the solution seems nice at first glance, but it requires your own JUnit runner hence it has disadvantage: you cannot use this annotation with e.g. Mockito runner.
Is it bad to throw an exception in a test?
Sometimes it is tempting to expect general Exception, RuntimeException or even a Throwable. And this is considered as a bad practice, because your code may throw exception in other place than you actually expected and your test will still pass!
Can JUnit 5 be used in tests?
JUnit 5 brought pretty awesome improvements and it differs a lot from its predecessor. JUnit 5 requires Java 8 at runtime hence Lambda expressions can be used in tests, especially in assertions. One of those assertions is perfectly suited for testing exceptions.
Why test exception flows?
Why test exception flows? Just like with all of your code, test coverage writes a contract between your code and the business functionality that the code is supposed to produce leaving you with a living documentation of the code along with the added ability to stress the functionality early and often. I won't go into the many benefits of testing instead I will focus on just Exception Testing.
What is expected annotation in JUnit?
JUnit responded back to the users need for exception handling by adding a @Test annotation field "expected". The intention is that the entire test case will pass if the type of exception thrown matched the exception class present in the annotation.
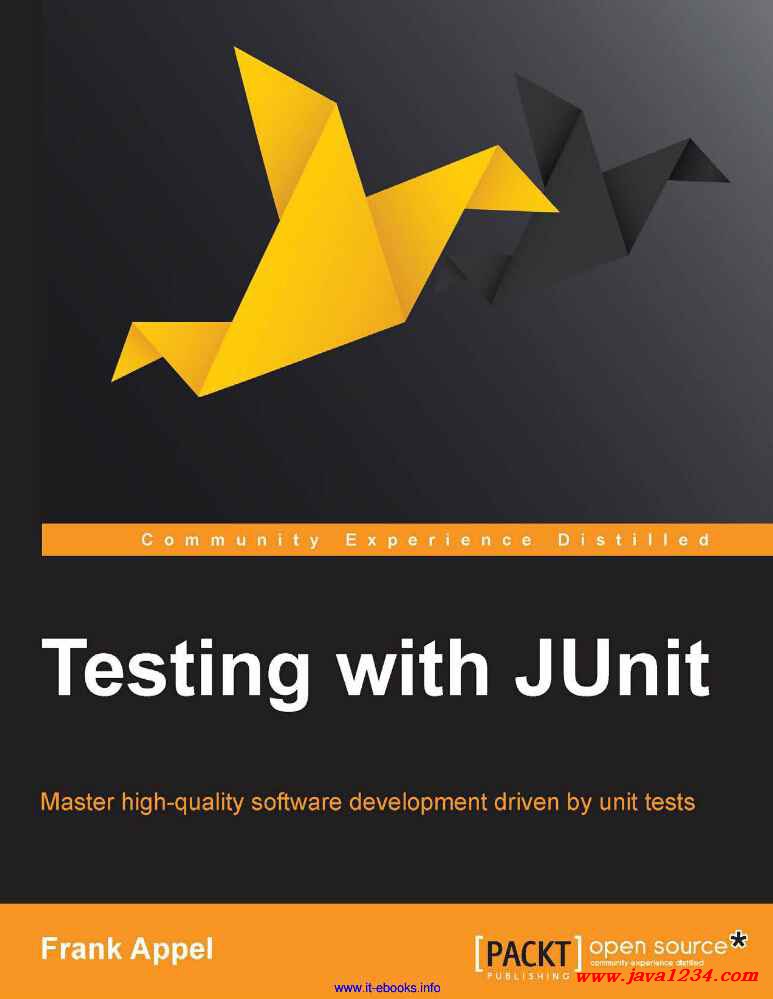