
What is the difference between try and catch in C++?
The try statement allows you to define a block of code to be tested for errors while it is being executed. The catch statement allows you to define a block of code to be executed, if an error occurs in the try block. The try and catch keywords come in pairs: This will generate an error, because myNumbers [10] does not exist.
What happens when an exception is thrown in a try catch?
When an exception is thrown, the .NET CLR checks the catch block and checks if the exception is handled. One try block can have multiple catch blocks. A try catch statement can have other nested try catch statements. In C#, the try..catch statement is responsible for exception handling. A typical try..catch block looks like Listing 1.
Can a TRY CATCH statement have multiple catch blocks?
One try block can have multiple catch blocks. A try catch statement can have other nested try catch statements. In C#, the try..catch statement is responsible for exception handling. A typical try..catch block looks like Listing 1. The suspect code is placed inside the try block and catch declares an exception. Listing 1.
How does the try/catch block work in Python?
It uses a long jump out of the current function to the try block. The try block then uses an if/else to skip the code block to the catch block which check the local variable to see if it should catch.
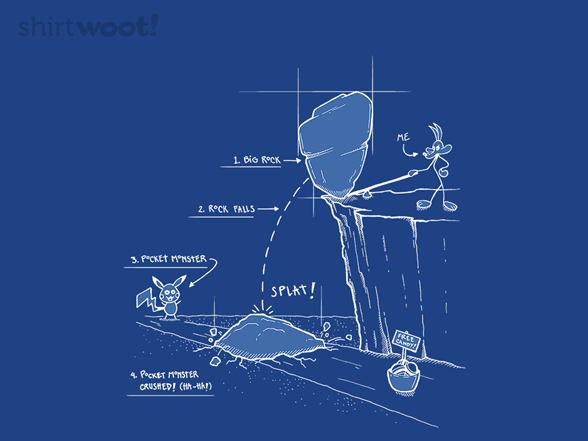
Does try catch exist in C?
Try-Catch mechanisms are common in many programming languages such as Python, C++, and JavaScript.
Does try catch stop execution?
First, the code in try {...} is executed. If there were no errors, then catch (err) is ignored: the execution reaches the end of try and goes on, skipping catch . If an error occurs, then the try execution is stopped, and control flows to the beginning of catch (err) .
Should I wrap everything try catch?
You should catch the exceptions you can handle fully or partially, rethrowing the partial exceptions. You should not catch any exceptions that you can't handle, because that will just obfuscate errors that may (or rather, will) bite you later on.
What happens if try catch block is not used in C++?
The technical term for this is: C++ will throw an exception (error).
Why we use try-catch?
Java try and catch The try statement allows you to define a block of code to be tested for errors while it is being executed. The catch statement allows you to define a block of code to be executed, if an error occurs in the try block.
Is try-catch asynchronous?
The following code reproduces the example. Here a try.. catch block is used to wrap a call to setImmediate() . It is a function that operates asynchronously and schedules the argument callback to be called in the near future, as soon as other operations have finished.
Where do you put try catch?
Put the try-catch where you are sure you won't just swallow the exception. Multiple try-catch blocks in various layers may be OK if you can ensure consistency. For example, you may put a try-catch in your data access layer to ensure you clean up connections properly.
Are variables in try catch local?
Variables declared inside a try or catch block are local to the scope of the block.
What is a checked exception?
A checked exception is a type of exception that must be either caught or declared in the method in which it is thrown. For example, the java.io.IOException is a checked exception.
Can we handle exception without catch block?
throws: The throws keyword is used for exception handling without try & catch block. It specifies the exceptions that a method can throw to the caller and does not handle itself.
How does try catch work in C++?
The try statement allows you to define a block of code to be tested for errors while it is being executed. The throw keyword throws an exception when a problem is detected, which lets us create a custom error. The catch statement allows you to define a block of code to be executed, if an error occurs in the try block.
Can we use catch () without passing arguments in it?
Some exception can not be catch(Exception) catched. Below excecption in mono on linux, should catch without parameter. Otherwise runtime will ignore catch(Exception) statment.
How do you end a try catch block?
Wrap the try/catch in a do{}while(false); loop.
How do you handle execution exception?
Just make your method throws Exception and throw all these with the same line of code. You can catch ExecutionException and just throw e. getCause -- and don't catch anything else, just let it propagate on its own.
What is the difference between exception and runtime exception?
The Runtime Exception is the parent class in all exceptions of the Java programming language that are expected to crash or break down the program or application when they occur. Unlike exceptions that are not considered as Runtime Exceptions, Runtime Exceptions are never checked.
Why does C++ have a try catch?
C++ has a try-catch construct specifically to deal with unexpected errors like this, regardless of the cause of each error. To understand how try-catch works in C++, let’s first take a look at exceptions.
How to use a try block?
To make use of a try block, you must also add a catch clause to your program. A catch block specifies the type of exception it can handle and allows you to define the code executed when the error occurs.
What Are Exceptions in C++?
Errors can appear in code either during the compilation stage or while the program is running. Logical and syntax errors appearing in code during compilation will need to be resolved by the C++ developer in charge. There’s no hope of using your code until these issues have been resolved.
What is exception handling in C++?
In C++, exception handling is a means for code to identify and deal with runtime errors. A C++ program is able to use a unique set of functions called handlers to keep a watchful eye on a particular section of the program’s code.
What is the difference between C and C++?
Although the C and the C++ programming languages have many similarities, exception handling is one significant difference . Handlers and their ability to react to exceptions do not exist in C, and programmers are forced to plan exceptions out of their code instead of being able to react to them if and when they happen.
What is exception handler function?
Using exception handler functions specifically identifies that a block of code is reserved for dealing with errors as these appear in runtime. This makes it easy to locate and maintain exception handling code and gives your overall program a cleaner look.
Why do you use if/else in code?
Managing exceptions with if/else with the normal flow of your code can lead to readability issues and confusion, especially since exception handling code will not always be run. Adding if/else statements to your code can result in duplicate code, something that well-designed exception handlers mitigate.
What is try catch in C#?
C# Try Catch. The try..catch statement in C# is used in exception handling. In this article, I'll explain the use of try-catch in a .NET application including try-catch-finally and try-catch-finally-throw.
What is the purpose of the finally block?
One of the key purposes of the "finally" block is to clean up any resources that were used in the try block. For example, if an IO resource is used to open and read a file, the IO resource must be released.
What is the file stream in Listing 7?
The code in Listing 7 creates a FileStream and reads a file that is not there. Obviously, the code will throw an exception that is not handled. But we want to make sure the FileStream object is released so we call FileStream.Close () method in the finally block.
What is the complete exception handling syntax?
The complete exception handling syntax is try..catch..block that makes sure the exceptions are caught and any resources are cleaned up. Listing 8 is an example of using a try..catch..finally block.
Can C# throw exceptions?
Exceptions are usually thrown by the runtime but C# has the flexibility to throw exceptions manually. A library creator can throw exceptions when certain conditions are not met and a caller program can catch the exceptions.
Can you use the throw keyword in C#?
So far, we’ve seen the use of throw keyword as a statement but starting with C# 7, the throw keyword can also be used as an expression. Now, with the use of an expression, the simple condition can be used in the same line that makes the code more readable and cleaner.
Can you use multiple catch blocks in C#?
C# allows to use multiple catch in a try..catch block to catch specific exceptions. Let’s take at the code in Listing 5, that may generate Stack overflow, divide by zero, and invalid cast exceptions. We can handle this using separate catch blocks for each exception type.
