How is HashSet implemented internally in Java?
How is HashSet implemented internally in Java? 1 HashMap as a Backing DataStructure. HashSet internally uses HashMap as a backing data structure with key as generic type E and value as Object class type. 2 Remove () method. ... 3 Summary and Other Important Points. ... 4 About the Author. ... 5 Comments and Queries
What is the backing data structure of HashSet in Java?
HashSet internally uses HashMap as a backing data structure with key as generic type E and value as Object class type. Have a look at below code snippet of HashSet class from jdk 1.6
What are the methods in HashSet?
Methods in HashSet METHOD DESCRIPTION iterator () Used to return an iterator over the elem ... isEmpty () Used to check whether the set is empty o ... size () Used to return the size of the set. clone () Used to create a shallow copy of the set ... 4 more rows ...
What are the basic properties of HashSet?
Before going into internal implementation HashSet, we should know below two basic properties of HashSet . HashSet doesn't allow duplicate elements. It allows at most one null element. Both of these properties can be achieved using HashMap as HashMap doesn't allow duplicate keys and it also allows only one null key.
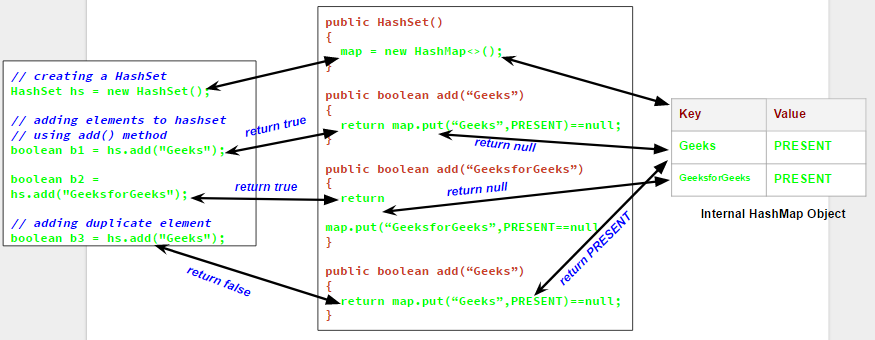
How is a HashSet implemented in Java?
HashSet internally uses HashMap to store it's elements. Whenever you create a HashSet object, one HashMap object associated with it is also created. This HashMap object is used to store the elements you enter in the HashSet. The elements you add into HashSet are stored as keys of this HashMap object.
Does HashSet implement set?
The HashSet class implements the Set interface, backed by a hash table which is actually a HashMap instance. No guarantee is made as to the iteration order of the set which means that the class does not guarantee the constant order of elements over time. This class permits the null element.
How is a set implemented internally?
Now as you can see that whenever we create a HashSet, it internally creates a HashMap and if we insert an element into this HashSet using add() method, it actually call put() method on internally created HashMap object with element you have specified as it's key and constant Object called “PRESENT” as it's value.
How is HashMap implemented?
Hashmap uses the array of Nodes(named as table), where Node has fields like the key, value (and much more). Here the Node is represented by class HashMapEntry. Basically, HashMap has an array where the key-value data is stored. It calculates the index in the array where the Node can be placed and it is placed there.
Does HashSet allow duplicates?
Duplicates: HashSet doesn't allow duplicate values. HashMap stores key, value pairs and it does not allow duplicate keys.
How are elements stored in HashSet?
Whenever an element is added to the HashSet, it is stored in a HashMap internally maintained by the it. The key is the object that we add in the it and value is a dummy object. HashSet is really useful when we want a data structure that contains no duplicate values.
Why HashSet has no get method?
HashSet does not have any method to retrieve the object from the HashSet. There is only a way to get objects from the HashSet via Iterator. When we create an object of HashSet, it internally creates an instance of HashMap with default initial capacity 16.
How are sets implemented in Java?
The Java platform contains three general-purpose Set implementations: HashSet , TreeSet , and LinkedHashSet . HashSet , which stores its elements in a hash table, is the best-performing implementation; however it makes no guarantees concerning the order of iteration.
How are set implemented?
General-Purpose Set Implementations. There are three general-purpose Set implementations — HashSet , TreeSet , and LinkedHashSet . Which of these three to use is generally straightforward. HashSet is much faster than TreeSet (constant-time versus log-time for most operations) but offers no ordering guarantees.
What is difference between HashMap and HashSet?
Hashmap is the implementation of Map interface. Hashset on other hand is the implementation of set interface. Hashmap internally do not implements hashset or any set for its implementation. Hashset internally uses Hashmap for its implementation.
How HashMap is implemented from scratch in Java?
An instance of HashMap can be created with a new operator. Map
How would you implement a HashMap in Java?
Implement the MyHashMap class:MyHashMap() initializes the object with an empty map.void put(int key, int value) inserts a (key, value) pair into the HashMap. ... int get(int key) returns the value to which the specified key is mapped, or -1 if this map contains no mapping for the key .More items...
Is a HashSet a set?
A HashSet is a set where the elements are not sorted or ordered. It is faster than a TreeSet. The HashSet is an implementation of a Set. Set is a parent interface of all set classes like TreeSet, HashSet, etc.
What is difference between HashSet and HashMap?
Hashmap is the implementation of Map interface. Hashset on other hand is the implementation of set interface. Hashmap internally do not implements hashset or any set for its implementation. Hashset internally uses Hashmap for its implementation.
Why HashSet has no get method?
HashSet does not have any method to retrieve the object from the HashSet. There is only a way to get objects from the HashSet via Iterator. When we create an object of HashSet, it internally creates an instance of HashMap with default initial capacity 16.
What is difference between HashSet and Hashtable?
You can use one null key and which kind of null values you want for HashMap but Hashtable does not allow null key or null values. Basically under HashSet is working HashMap where value is Object hence HashSet values are unique because HashMap keys are unique.
What is a hashset?
HashSet extends Abstract Set<E> class and implements Set<E>, Cloneable and Serializable interfaces where E is the type of elements maintained by this set. The directly known subclass of HashSet is LinkedHashSet.
What is a hashset in a collection?
HashSet (Collection): This constructor is used to build a HashSet object containing all the elements from the given collection. In short, this constructor is used when any conversion is needed from any Collection object to the HashSet object.
How does add method work in HashSet?
We can notice that, add () method of HashSet class internally calls the put () method of backing the HashMap object by passing the element you have specified as a key and constant “PRESENT” as its value. remove () method also works in the same manner. It internally calls remove method of Map interface.
How does load factor affect hashset performance?
Effect on performance: Load factor and initial capacity are two main factors that affect the performance of HashSet operations. A load factor of 0.75 provides very effective performance with respect to time and space complexity. If we increase the load factor value more than that then memory overhead will be reduced (because it will decrease internal rebuilding operation) but, it will affect the add and search operation in the hashtable. To reduce the rehashing operation we should choose initial capacity wisely. If the initial capacity is greater than the maximum number of entries divided by the load factor, no rehash operation will ever occur.
What is a hashset class?
The HashSet class implements the Set interface, backed by a hash table which is actually a HashMap instance. No guarantee is made as to the iteration order of the set which means that the class does not guarantee the constant order of elements over time. This class permits the null element. The class also offers constant time performance for the basic operations like add, remove, contains, and size assuming the hash function disperses the elements properly among the buckets, which we shall see further in the article.
How many objects are needed to add to a hashmap?
HashMap requires two objects put (K key, V Value) to add an element to the HashMap object. HashSet internally uses HashMap to add elements. In HashSet, the argument passed in add (Object) method serves as key K. Java internally associates dummy value for each value passed in add (Object) method.
What is the underlying data structure for HashSet?
The underlying data structure for HashSet is Hashtable.
What does "put" mean in a hashmap?
As we know in a HashMap each key is unique and when we call put (Key, Value) method, it returns the previous value associated with key, or null if there was no mapping for key. So in add () method we check the return value of map.put (key, value) method with null value.
Is a set a collection of objects?
As we know that a set is a well-defined collection of distinct objects. Each member of a set is called an element of the set. So in other words, we can say that a set will never contain duplicate elements. But how in java Set interface implemented classes like HashSet, LinkedHashSet, TreeSet etc. achieve this uniqueness. In this post, we will discuss the hidden truth behind this uniqueness.
