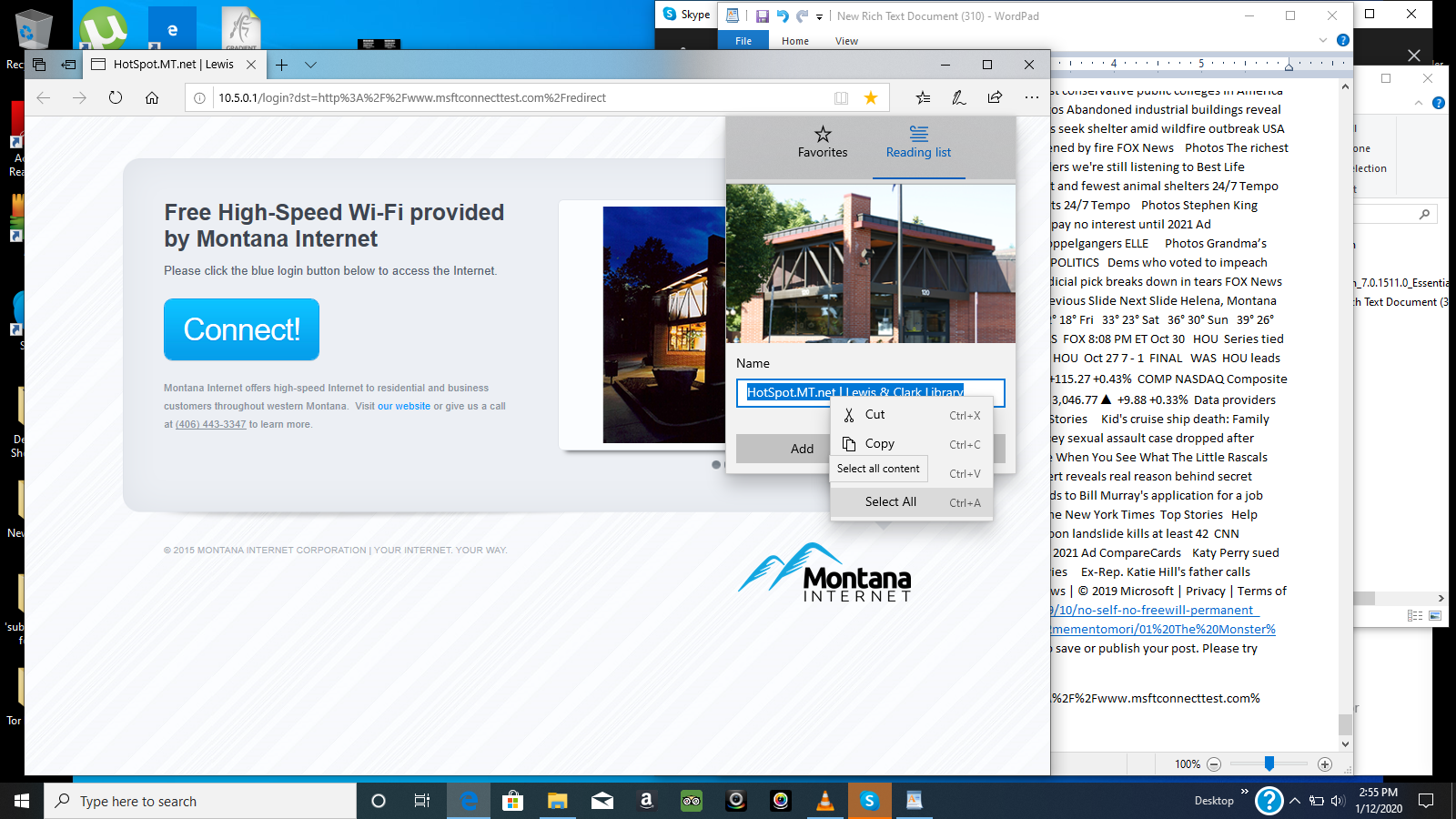
No, it's not necessary for every class to implement an interface. Use interfaces only if they make your code cleaner and easier to write. If your program has no current need for to have more than 1 implementation for a given class, then you don't need an interface.
Does every class have an interface that it implements?
I have seen code where every class has an interface that it implements. Sometimes there is no common interface for them all. They are just there and they are used instead of concrete objects. They do not offer a generic interface for two classes and are specific to the domain of the problem that the class solves.
Should I use interfaces for everything?
Edit: Personally, I like the notion of using Interfaces for everything as a methodology and habit, even if it's not clearly beneficial. Eclipse automatically created a class file with all the methods, so it doesn't waste any time anyway. You don't need to create an interface if you are not going to use it.
What is the purpose of interfaces in Java?
Edit: Interfaces provide a sort of abstraction. They are particularly useful if you want to interchange between different implementations (many classes implementing the same interface). If it is just a single class, then there is no gain.
Is it possible to have a generic interface for two classes?
They do not offer a generic interface for two classes and are specific to the domain of the problem that the class solves. Is there any reason to do that? Show activity on this post. No.
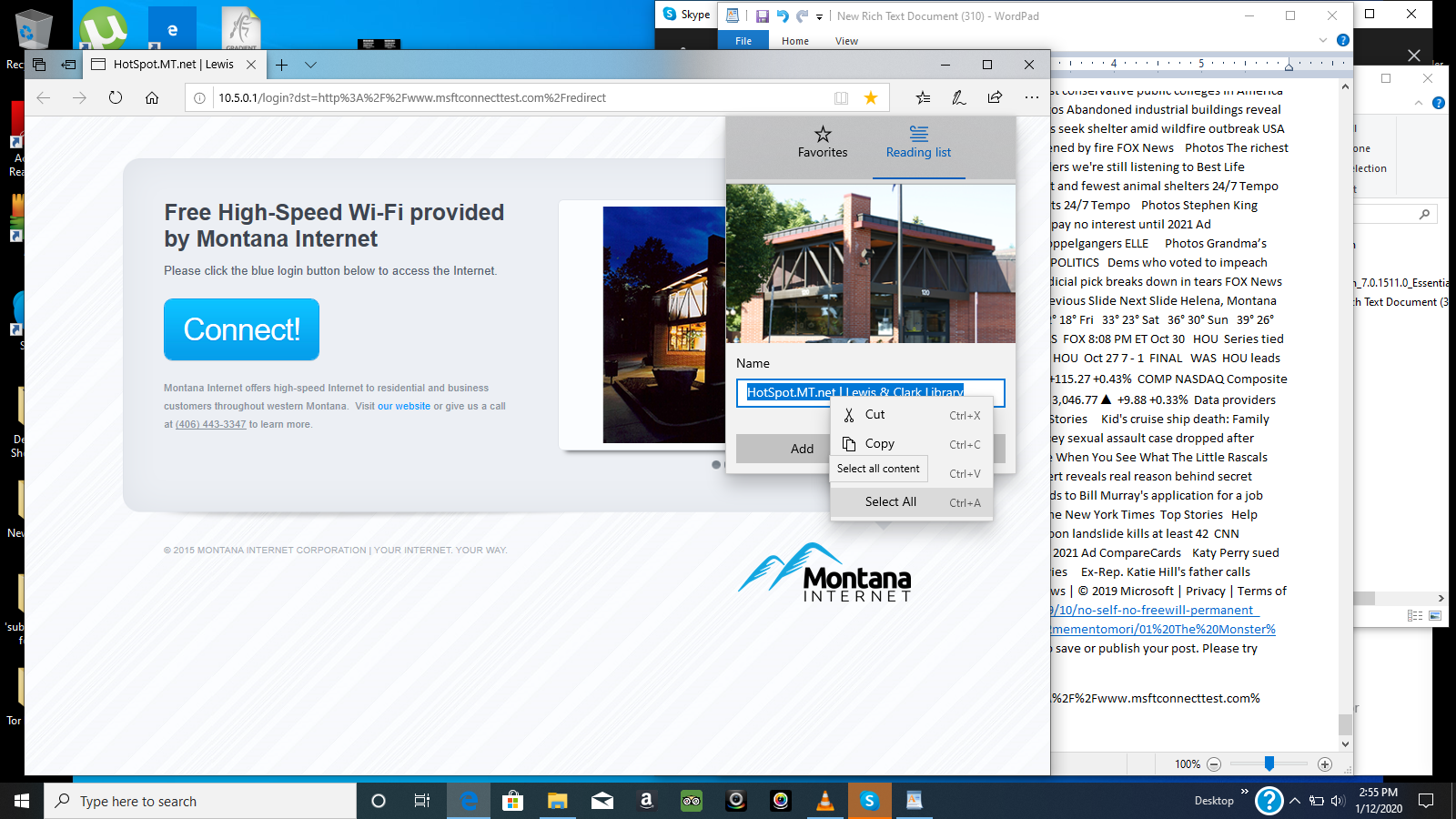
How many interfaces should a class implement?
A class can implement more than one interface at a time. A class can extend only one class, but implement many interfaces. An interface can extend another interface, in a similar way as a class can extend another class.
When should a class implement an interface?
A class implements an interface if it declares the interface in its implements clause, and provides method bodies for all of the interface's methods. So one way to define an abstract data type in Java is as an interface, with its implementation as a class implementing that interface.
What is the point of having every service class have an interface?
If, as the OP claims, you could just as easily write the program without the interface your tests would be just as valid or invalid depending only upon the choices made by the architect.
Do I need to use an interface when only one class will ever implement it?
Interfaces can be implemented by multiple classes. There is no rule that only one class can implement these. Interfaces provide abstraction to the java.
Why are interfaces better than classes?
Differences between a Class and an Interface: A class can be instantiated i.e, objects of a class can be created. An Interface cannot be instantiated i.e, objects cannot be created. Classes does not support multiple inheritance. Interface supports multiple inheritance.
Why interface is important in OOP?
Interfaces are useful in object-oriented programming because they specify the behavior that an object must implement. An interface is a contract between an object and its code. If an object implements an interface, it is guaranteed to support the behavior specified by that interface.
Why do we need interface in spring?
Using Interfaces allows your classes to extend from some other classes if required. Your Interfaces can have multiple implementations and you can switch between any of them without changing the client code.
How do you declare an interface?
To declare an interface, use the interface keyword. It is used to provide total abstraction. That means all the methods in an interface are declared with an empty body and are public and all fields are public, static, and final by default.
Is Java interface necessary?
In Java, an interface specifies the behavior of a class by providing an abstract type. As one of Java's core concepts, abstraction, polymorphism, and multiple inheritance are supported through this technology. Interfaces are used in Java to achieve abstraction.
Why interface is necessary in any team?
These interactions between your system and others are interfaces. Identifying interfaces helps you to define your system's boundaries. Identifying interfaces also helps you understand the dependencies your system has with other systems and dependencies other systems have with your system.
Can a class implement 2 interfaces?
Your class can implement more than one interface, so the implements keyword is followed by a comma-separated list of the interfaces implemented by the class.
When should interface be used in Java?
The interface in Java is a mechanism to achieve abstraction. There can be only abstract methods in the Java interface, not the method body. It is used to achieve abstraction and multiple inheritance in Java. In other words, you can say that interfaces can have abstract methods and variables.
When should we use interface in Java?
In Java, an interface specifies the behavior of a class by providing an abstract type. As one of Java's core concepts, abstraction, polymorphism, and multiple inheritance are supported through this technology. Interfaces are used in Java to achieve abstraction.
Which class can implement an interface?
The implements keyword is used to implement an interface . The interface keyword is used to declare a special type of class that only contains abstract methods. To access the interface methods, the interface must be "implemented" (kinda like inherited) by another class with the implements keyword (instead of extends ).
When we implement an interface method it must be declared as?
Explanation: In Java, when we implement an interface method, it must be declared as Public.
What are some examples of classes that do not implement interfaces?
You will find many examples of classes that do not implement any interfaces, or that are meant to be used directly, such as java.util.StringTokenizer.
Why are interfaces useful?
Edit: Interfaces provide a sort of abstraction. They are particularly useful if you want to interchange between different implementations (many classes implementing the same interface). If it is just a single class, then there is no gain.
Why are interfaces not contracts?
If you see a significant increase in complexity due to separating interface and implementation then you are probably not using interfaces as contracts. Interfaces reduce complexity. From the consumer's perspective, components become commodities that fulfil the terms of a contract instead of entities that have sophisticated implementation details in their own right.
Why is it important to define public methods in a class?
This allows for easy inversion of control, and it also facilitates unit testing with mocking and stubbing. It also gives you the liberty of replacing the implementation with some other class that implements that interface, so if you are into TDD it may make things easier (or more contrived if you are a critic of TDD)
What happens if you add a service provider to an abstract class?
1. If you use Service Provider Interface pattern in your application interfaces are harder to extend than abstract classes. If you add method to interface, all service providers must be rewritten. But if you add non-abstract method to the abstract class, none of the service providers must be rewritten.
Why is it preferable for classes meant for public consumption outside your package/project to implement interfaces?
Generally speaking, it is preferable for classes meant for public consumption outside your package/project to implement interfaces so that your users are less coupled to your implementation du jour.
What happens if you don't implement an interface?
If you don't implement an interface (and use some kind of factory for object creation) then certain kinds of changes will force you to break the Open-Closed Principle. In some situations this is commercially acceptable, in others it isn't.
Why is an interface useful?
An interface can be useful here, because it reduces the size of the touch point between modules. Notice the weasel words above: "may" be a candidate, "can be" useful. There is no hard and fast rule that says "yes" or "no" for a given situation. That being said, having an interface for everything is most likely overkill.
What is an interface in Java?
From a purely object-oriented perspective, every class already has an interface. An interface is nothing more than the public-facing methods and data members of a class. We typically use "interface" to mean "a Java class defined using the interface keyword" or "a C++ class with only public, pure virtual functions.".
How to test multilayer application?
If you want to test a multi-layer application without having to craft in objects for the various layers, you'll undoubtedly want to use mocking frameworks to mock out the layers. Interfaces are used extensively here.
Can you send a "reader" to a method?
For instance, you may send a "Reader" to a method, so that such a called method can use it's "read ()" method. By declaring an interface "Reader" you can make any object conform to this contract, by implementing the methods it specifies.
Do not take things you read as a rule?
Don't take things you read as a rule. People tend to read something, which most of the time doesn't fit her/his context, then they want to apply that new knowledge, which is fine, but you will eventually question. If you are against using it, but your team requires it, because is a "convention", then, move on.
Is having an interface for everything overkill?
That being said, having an interface for everything is most likely overkill. If you see this pattern, you are going to far:
What is implicit interface in a class?
A class always has an implicit interface, consisting of all its public methods. This is how the class will be known to other classes who use it.
Why add an interface to a collaboration?
By the way, adding an interface for those collaborating objects also helps the user to decorate existing implementations of the interface by using object composition.
How to make an implicit interface explicit?
An implicit interface can easily be turned into an explicit one by collecting all those public methods (except for the constructor, which should not be considered a regular method), stripping the method bodies and copying the remaining method signatures into an interface file.
Why is the commandbus class a class?
The package ships with a CommandBus class. It's a class, because its implicit interface isn't larger than its explicit interface would be - the only public method is handle ().
What is the principle of interface segregation?
You may know this strategy from the Interface segregation principle, which tells you not to let clients depend on methods they don't use (or shouldn't use!).
Why is abstraction used in programming?
Such an interface is often called an "abstraction", because it abstracts away the details that don't matter to the client of that interface.
Can you mock a final class?
Candidates for just being a final class are fairly broad, but obviously cannot be mocked without some hacky workaround ( https://github.com/dg/bypas...
What happens if you add an interface to a class?
If you add an interface to such class and then inject this interface, you have absolutely no control of how it would be used. Especially when you are making an API or a library used by another application.
What are interfaces used for?
Interfaces have great value and they should be used in every application. Thanks to interfaces, you can use polymorphism, add many patterns such us Strategy, Factory, Command and so on. You can inverse dependencies (D in SOLID) so if your domain uses repositories or adapters, you can inject interfaces and the implementation should be in a separate layer.
Why is initialization class bigger?
The bigger a project is, the more entries are added when registering IoC Container. Initialization classes become bigger and harder to maintain. If you need to register dependencies in two different scopes (e.g., request scope for WebApi and Named scope for some asynchronous endless loop), you need to register your dependencies twice and it is a disaster.
Why use interfaces instead of concrete implementations?
Using interfaces instead of concrete implementations reduce coupling and that's a fact. But registering interface in the IoC Container adds complexity and lowers maintainability so if you use it only once in the application, it equalizes pros and cons in my opinion.
What is the first use case of an interface?
The very first use case of an interface is to describe behavior of an object that implements it. If a class Dog implements interface IAnimal, we are assured it (not always) can Move and Eat. Of course, a Dog can also Bark or Sit, but this is specific implementation that differs from a Bird that can Fly.
When should an IOC container resolve a class?
IoC Container should resolve a class when it contains a public constructor with all parameters that can be resolved by IoC Container. If you add an interface JUST for a test - don't. Your production code should not be written just to satisfy tests.
Do you need to register classes in IoC container?
What is more, if you use IoC container (e.g., Ninject), you need to register all classes with their interfaces. This is another thing to remember and it may look like this:
