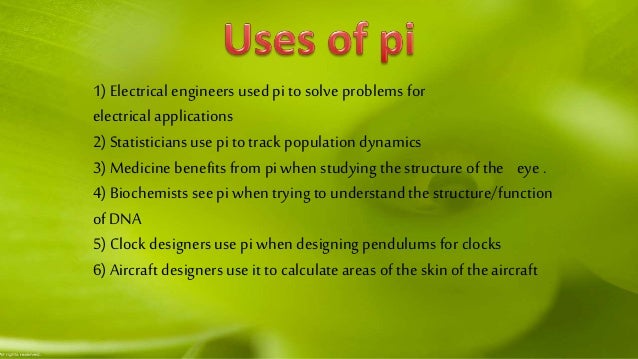
What are the basics of data structures?
Basic data structures every software engineer must know A data structure is a specialized way of organizing data that allows efficiently process it with associated algorithms. Since data is the most crucial entity in computer science, so the true worth of data structures is clear.
What are the types of data structure?
Types of data structures
- Arrays. Arrays store similar items together. ...
- Stacks. Stacks use a last in, first out (LIFO) structure where the computer orders previous work with the last action appearing first.
- Queues. Contrary to stacks, queues follow a first in, first out (FIFO) structure for organizing data. ...
- Linked lists. ...
- Binary trees. ...
- Graphs. ...
- Tries. ...
- Hash tables. ...
- Skip lists. ...
What is basic data structure?
In simple words data structure is the way of organizing data in efficient manner. Data structures are different from abstract data types in the way they are used. Data structures are the implementations of abstract data types in a concrete and physical setting. They do this by using algorithms.
What are data structures and algorithms?
[Course] Data structures and Algorithms mit – Best This Course ” Data structures and Algorithms mit ” provides an introduction to mathematical modeling of computational problems. It covers the common algorithms, algorithmic paradigms, and data structures used to solve these problems.
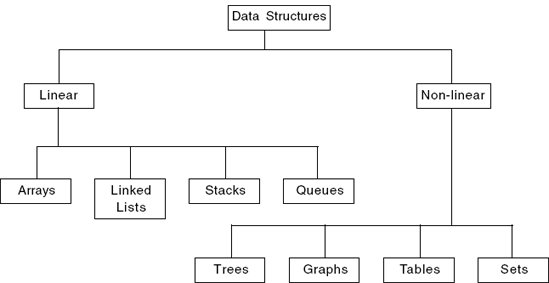
Why is data structure used?
Data structure provides a way of organizing, managing, and storing data efficiently. With the help of data structure, the data items can be traversed easily. Data structure provides efficiency, reusability and abstraction.
Where data structure is used in real life?
To store a set of fixed key words which are referenced very frequently. To store the customer order information in a drive-in burger place. (Customers keep on coming and they have to get their correct food at the payment/food collection window.) To store the genealogy information of biological species.
What are the 4 data structures?
When we think of data structures, there are generally four forms: Linear: arrays, lists. Tree: binary, heaps, space partitioning etc. Hash: distributed hash table, hash tree etc.
What are the 2 main types of data structures?
Basically, data structures are divided into two categories: Linear data structure. Non-linear data structure.
What is data structure example?
Data Structure can be defined as the group of data elements which provides an efficient way of storing and organising data in the computer so that it can be used efficiently. Some examples of Data Structures are arrays, Linked List, Stack, Queue, etc.
Which data structure is best?
An array is the simplest and most widely used data structure. Other data structures like stacks and queues are derived from arrays.
What is data structure explain?
A data structure is a specialized format for organizing, processing, retrieving and storing data. There are several basic and advanced types of data structures, all designed to arrange data to suit a specific purpose. Data structures make it easy for users to access and work with the data they need in appropriate ways.
What are basic data structures?
The data structures used for this purpose are Arrays, Linked list, Stacks, and Queues. In these data structures, one element is connected to only one another element in a linear form. When one element is connected to the 'n' number of elements known as a non-linear data structure. The best example is trees and graphs.
What are some applications of data structures?
Data structures, including linked lists for memory allocation, file directory management and file structure trees, as well as process scheduling queues, are used to allow core operating system (OS) resources and functions.
In what areas do data structures are applied?
In general, data structures are used to implement the physical forms of abstract data types. Data structures are a crucial part of designing efficient software. They also play a critical role in algorithm design and how those algorithms are used within computer programs.
What are the 6 applications of stack?
Following are the applications of stack:Expression Evaluation.Expression Conversion. i. Infix to Postfix. ii. Infix to Prefix. iii. Postfix to Infix. iv. Prefix to Infix.Backtracking.Memory Management.
What is an example of an algorithm in real life?
Any step-by-step process that is completed the same way every time is an algorithm. A good example of this in everyday life is tying your shoes. There are a limited number of steps that effectively result in a traditional shoelace know (known as the “bunny rabbit” or “loop, swoop and pull” knot).
Why are data structures important?
Importance of Data Structure 1 Data Structures allow programmers to manage the data in the most efficient manner that enhances the algorithm’s performance. 2 They allow the implementation of a scientific approach as against traditional methodologies. When it comes to aspects such as processing speed, handling or multiple requests and searching through numerous records, data structures prove handy. 3 They allow efficient use of memory. Data structures optimize memory usage. This makes an impact usually in situations that involve the processing of huge datasets. 4 Data structures are reusable. They can be integrated as a package into a library. These libraries can be used in various situations by various stakeholders as needed. 5 They allow multiple solutions for a particular problem, allowing the user to select the data structure to solve the problem in the best way.
What is the basis of data structure?
The data can also be retrieved from the storage space. The basis of any data structure is to arrange data very well. The data can be accessed and worked appropriately in different ways.
How does a binary search tree work?
As the name goes, it works on the idea of two values. Initially, we have a root node that stores some value, say X. In the next level, we have two branches. The left branch leads to nodes representing all values less than x, while the right branch leads to nodes representing all values more than x. The tree’s division into several branches continues to happen based on the binary condition, and we get a binary tree. Data arranged using this data structure allows for efficient search operations.
What is the insertion operation in stacks?
In the case of stacks, the insertion operation is referred to as Push, as the element is pushed into the stack. The stack’s deletion operation is called Pop, as we pop the element up to bring it out. In the case of queues, the insertion operation is referred to as Enqueue; while on the other hand, the deletion operation is called dequeue.
Is a data structure reusable?
Data structures are reusable. They can be integrated as a package into a library. These libraries can be used in various situations by various stakeholders as needed.
Can a task be performed using multiple data structures?
A task can be performed using multiple data structures, but then selecting a particular type of data structure should be based on the context.
What is data structure?
It is a set of algorithms that can be used in any programming language to organize the data in the memory.
Why is data structure important?
With the help of data structure, the data items can be traversed easily. Data structure provides efficiency, reusability and abstraction. It plays an important role in enhancing the performance of a program because the main function of the program is to store and retrieve the user’s data as fast as possible.
What is stack in data?
Stack is a linear data structure that follows a specific order during which the operations are performed. The order could be FILO (First In Last Out) or LIFO (Last In First Out).
What is a queue in data?
Queue is a linear data structure in which elements can be inserted from only one end which is known as rear and deleted from another end known as front. It follows the FIFO (First In First Out) order.
How many types of data structures are there?
There are 2 types of Data Structure :
Is manipulation of large amounts of data easier?
Manipulation of large amounts of data is easier.
What is data structure?
The logical or mathematical model of a particular organization of data is called a data structure. The data structure is a way of storing and accessing the data into an acceptable form for computers. So that a large number of data is processed in a small interval of time. In a simple way, we say that storing the data into computer memory is called a data structure.
Why is data structure important in computer science?
In computer science, the Importance of data structure is everywhere. Data structure provides basic stuff to resolve problems. Its importance can be understood by the following: 1) A software has two parts front end and back end.
What is the logical and mathematical organization of data called?
We know that data may be organised in many different ways, the logical & mathematical organization of data is called data structure . When we apply this data structure it comes up with the implementation of stack, queue, list, trees, sorting and searching of data.
What is the method of arranging data alphabetically?
5) If data is to be arranged alphabetically or numerically then it is done by sorting method which is an operation on the data structure.
Why is queue important?
Queue: Implementation of the queue is also an important application of data structure. Nowadays computer handles multiuser, multiprogramming environment. In this environment a system (computer) handles several jobs at a time, to handle these jobs the concept of a queue is used.
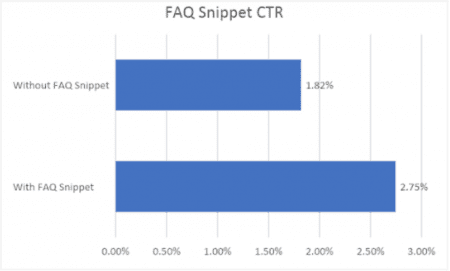
Types of Data Structures
Importance of Data Structure
- Data Structures allowprogrammers to manage the data in the most efficient manner that enhances the algorithm’s performance.
- They allow the implementation of a scientific approach as against traditional methodologies. When it comes to aspects such as processing speed, handling or multiple requests and searching through n...
- Data Structures allowprogrammers to manage the data in the most efficient manner that enhances the algorithm’s performance.
- They allow the implementation of a scientific approach as against traditional methodologies. When it comes to aspects such as processing speed, handling or multiple requests and searching through n...
- They allow efficient use of memory. Data structures optimize memory usage. This makes an impact usually in situations that involve the processing of huge datasets.
- Data structures are reusable. They can be integrated as a package into a library. These libraries can be used in various situations by various stakeholders as needed.
Conclusion
- Working with data structures helps the user perform data operations effectively. Various operations, such as insertion, deletion, searching, etc., can be performed using them. A task can be performed using multiple data structures, but then selecting a particular type of data structure should be based on the context.
Recommended Articles
- This is a guide to What is Data Structure?. Here we discuss the different types of Data Structure and Importance along with Examples. You can also go through our suggested articles to learn more – 1. Free Data Analysis Tools 2. Types of Data Analysis Techniques 3. Data Analytics vs Data Analysis 4. What is Data Integration?
Need of Data Structure
Types of Linear Data Structure –
- 1] Arrays – An array is a collection of similar data elements stored at contiguous memory locations. It is the simplest data structure where each data element can be accessed directly by only using its index number. 2] Linked List – A linked list is a linear data structure that is used to maintain a list-like structure in the computer memory. It is a group of nodes that are not stored a…
Types of Non-Linear Data Structure
- 1] Tree – A tree is a multilevel data structure defined as a set of nodes. The topmost node is named root node while the bottom most nodes are called leaf nodes. Each node has only one parent but can have multiple children.
Types of Trees in Data Structure
- General Tree
- Binary Tree
- Binary Search Tree
- AVL Tree
Types of Graph
- Finite Graph
- Infinite Graph
- Trivial Graph
- Simple Graph
Classification of Data Structure
- Data Structure can be further classified as 1. Static Data Structure 2. Dynamic Data Structure Static Data Structure Static Data Structures are data structures where the size is allocated at the compile time. Hence, the maximum size is fixed and cannot be changed. Dynamic Data Structure Dynamic Data Structures are data structures where the size is allocated at the run time. Hence, t…
Data Structure Operations –
- The common operations that can be performed on the data structures are as follows : 1. Searching – We can easily search for any data element in a data structure. 2. Sorting – We can sort the elements either in ascending or descending order. 3. Insertion – We can insert new data elements in the data structure. 4. Deletion – We can delete the data elements from the data stru…
Advantages of Data Structure –
- Data structures allow storing the information on hard disks.
- An appropriate choice of ADT (Abstract Data Type) makes the program more efficient.
- Data Structures are necessary for designing efficient algorithms.
- It provides reusability and abstraction.