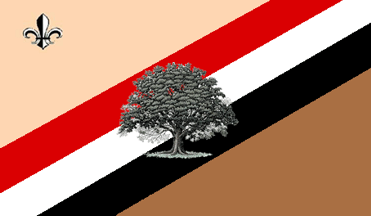
What is red-black tree in Java?
A red-black tree is a self-balancing binary search tree, that is, a binary search tree that automatically maintains some balance. Each node is assigned a color (red or black). A set of rules specifies how these colors must be arranged (e.g., a red node may not have red children).
Which is red-black tree property?
Properties of a red-black tree Each tree node is colored either red or black. The root node of the tree is always black. Every path from the root to any of the leaf nodes must have the same number of black nodes. No two red nodes can be adjacent, i.e., a red node cannot be the parent or the child of another red node.
How do red-black trees form?
The following are some rules used to create the Red-Black tree: If the tree is empty, then we create a new node as a root node with the color black. If the tree is not empty, then we create a new node as a leaf node with a color red. If the parent of a new node is black, then exit.
Where is red-black tree used?
Real-world uses of red-black trees include TreeSet, TreeMap, and Hashmap in the Java Collections Library. Also, the Completely Fair Scheduler in the Linux kernel uses this data structure. Linux also uses red-black trees in the mmap and munmap operations for file/memory mapping.
Who invented red black trees?
Red-black trees were invented in 1978, by two researchers named Leonidas J. Guibas and Robert Sedgewick, at Xerox PARC, a research and development company based in Palo Alto, California.
Why are trees red and black?
A red-black tree is a kind of self-balancing binary search tree where each node has an extra bit, and that bit is often interpreted as the color (red or black). These colors are used to ensure that the tree remains balanced during insertions and deletions.
What is RB tree give example?
A red-black tree is a Binary tree where a particular node has color as an extra attribute, either red or black. By check the node colors on any simple path from the root to a leaf, red-black trees secure that no such path is higher than twice as long as any other so that the tree is generally balanced.
Why red-black tree is useful?
A red black tree is a data structure which allows for Insertion, Deletion and Searching of the tree bounded by O(log(n)) time at the expense of an extra color bit for every node. It is easier to code than an AVL tree (which has near perfect balancing but slightly more overhead).
Is red-black tree AVL tree?
The complexity of tree operation in the red-black tree data structure is the same as the AVL tree. The red-black tree is a self-balancing binary search tree with the same complexity as the AVL tree.
How do you find a red-black tree?
Searching a node in Red Black Tree Perform a binary search on the records in the current node. If a record with the search key is found, then return that record. If the current node is a leaf node and the key is not found, then report an unsuccessful search. Otherwise, follow the proper branch and repeat the process.
What is the use of RB tree?
RB trees are used in functional programming to construct associative arrays. In this application, RB trees work in conjunction with 2-4 trees, a self-balancing data structure where every node with children has either two, three, or four child nodes.
What is a balance binary tree?
A balanced binary tree, also referred to as a height-balanced binary tree, is defined as a binary tree in which the height of the left and right subtree of any node differ by not more than 1.
What is a red black tree?
A red-black tree is a kind of self-balancing binary search tree where each node has an extra bit , and that bit is often interpreted as the colour (red or black). These colours are used to ensure that the tree remains balanced during insertions and deletions. Although the balance of the tree is not perfect, it is good enough to reduce the searching time and maintain it around O (log n) time, where n is the total number of elements in the tree. This tree was invented in 1972 by Rudolf Bayer.
What is the hard part of a red black tree?
In this post, we introduced Red-Black trees and discussed how balance is ensured. The hard part is to maintain balance when keys are added and removed. We have also seen how to search an element from the red-black tree. We will soon be discussing insertion and deletion operations in coming posts on the Red-Black tree.
What is the black depth of a node?
The black depth of a node is defined as the number of black nodes from the root to that node i.e the number of black ancestors.
How many nodes are there in a red black tree?
From property 3 of Red-Black trees, we can claim that the number of black nodes in a Red-Black tree is at least ⌊ n/2 ⌋ where n is the total number of nodes.
How to write a binary tree?
If k is 3, then n is at least 7). This expression can also be written as k <= Log 2 (n+1).
Which is better: AVL or Red Black?
The AVL trees are more balanced compared to Red-Black Trees, but they may cause more rotations during insertion and deletion. So if your application involves frequent insertions and deletions, then Red-Black trees should be preferred. And if the insertions and deletions are less frequent and search is a more frequent operation, then AVL tree should be preferred over Red-Black Tree.
What tree does MySQL use?
Moreover, MySQL also uses the Red-Black tree for indexes on tables.
Why do we need a red black tree?
The Red-Black tree is used because the AVL tree requires many rotations when the tree is large, whereas the Red-Black tree requires a maximum of two rotations to balance the tree. The main difference between the AVL tree and the Red-Black tree is that the AVL tree is strictly balanced, while the Red-Black tree is not completely height-balanced. So, the AVL tree is more balanced than the Red-Black tree, but the Red-Black tree guarantees O (log2n) time for all operations like insertion, deletion, and searching.
What is the purpose of each node in a red black tree?
Each node in the Red-black tree contains an extra bit that represents a color to ensure that the tree is balanced during any operations performed on the tree like insertion, deletion , etc. In a binary search tree, the searching, insertion and deletion take O (log2n) time in the average case, O (1) in the best case and O (n) in the worst case.
What is self-balancing in a tree?
Here, self-balancing means that it balances the tree itself by either doing the rotations or recoloring the nodes. This tree data structure is named as a Red-Black tree as each node is either Red or Black in color. Every node stores one extra information known as a bit that represents the color of the node.
What should the values of the nodes in the left subtree be?
In a binary search tree, the values of the nodes in the left subtree should be less than the value of the root node , and the values of the nodes in the right subtree should be greater than the value of the root node.
What happens if the parent of a new node is black?
If the parent of a new node is black, then exit.
What is the color of the root node in a binary tree?
Other information stored by the node is similar to the binary tree, i.e., data part, left pointer and right pointer. In the Red-Black tree, the root node is always black in color. In a binary tree, we consider those nodes as the leaf which have no child.
What color is a node?
As the name suggests that the node is either colored in Red or Black color. Sometimes no rotation is required, and only recoloring is needed to balance the tree.
What is the red black tree algorithm used for?
This algorithm is used for maintaining the property of a red-black tree if the insertion of a newNode violates this property.
What happens if a red node has children?
Red Property: If a red node has children then, the children are always black.
What happens when you insert a new node?
While inserting a new node, the new node is always inserted as a RED node. After insertion of a new node, if the tree is violating the properties of the red-black tree then, we do the following operations.
What is the red property?
Red Property: If a red node has children then, the children are always black. Depth Property: For each node, any simple path from this node to any of its descendant leaf has the same black-depth (the number of black nodes). An example of a red-black tree is: Red Black Tree. Each node has the following attributes: color.
Why is the black depth algorithm implemented when a black node is deleted?
This algorithm is implemented when a black node is deleted because it violates the black depth property of the red-black tree.
What is a red black tree?
Red-Black tree is a self-balancing binary search tree in which each node contains an extra bit for denoting the color of the node, either red or black.
Why is the red black color important?
The red-black color is meant for balancing the tree. The limitations put on the node colors ensure that any simple path from the root to a leaf is not more than twice as long as any other such path. It helps in maintaining the self-balancing property of the red-black tree.
What is the color of the leaf nodes in a tree?
While representing the red black tree color of each node should be shown. In this tree leaf nodes are simply termed as null nodes which means they are not physical nodes. It can be checked easily in the above-given tree there are two types of node in which one of them is red and another one is black in color.
What is the left-right imbalance in the Red Black Tree?
In this Red Black Tree violates its property in such a manner that parent and inserted child will be in a red color at a position of left and right with respect to grandparent. Therefore it is termed as Left Right imbalance.
What is the right left imbalance?
In this red black tree violates its property in such a manner that parent and inserted child will be in a red color at a position of right and left with respect to grandparent. Therefore it is termed as RIGHT LEFT imbalance.
What color is LR imbalancing?
For LR imbalancing recolor u to black and recolor p and g to red.
What are the properties of a red black tree?
Properties of Red Black Tree. The root node should always be black in color. Every null child of a node is black in red black tree. The children of a red node are black. It can be possible that parent of red node is black node. All the leaves have the same black depth.
When does imbalancing occur?
The imbalancing can also be occurred when the child of grandparent i.e. uncle node is black. Then know also four cases will be arises and in this case imbalancing can be removed by using rotation technique.
What is a red black tree?
A Red Black Tree is a type of self-balancing binary search tree, in which every node is colored with a red or black. The red black tree satisfies all the properties of the binary search tree but there are some additional properties which were added in a Red Black Tree. The height of a Red-Black tree is O (Logn) where ...
What is a red black tree?
A Red Black Tree could be a sort of self-balancing binary search tree, during which each node is colored with a red or black. The red black tree satisfies all the properties of the binary search tree however their square measure some further properties that were supplemental during a Red-Black Tree. the peak of a Red-Black tree is O (Logn) wherever (n is that the variety of nodes within the tree).
What tree is every null kid in?
Every null kid of a node is black in a red-black tree.
Which node contains a similar variety of black nodes?
Every straightforward path from the basis node to the (downward) leaf node contains a similar variety of black nodes.
Does each insertion cause imbalance?
Not each insertion causes imbalance however if imbalancing happens then it is often removed, relying upon the configuration of the tree before the new insertion is formed.
Is Piyush a good learner?
Piyush is a good learner & innovative. He is passionate about coding and likes to watch cricket & listening music. He has worked on projects like ZipPosRepots, ZipOrdering.
What is a red black tree?
A red black tree is a self balancing binary search tree with an extra attribute, which is that each node is either red or black.
What kernel uses a red tree?
The Completely Fair Scheduler of the Linux 2.6+ kernel uses a Red Black Tree implementation.
What are the attributes of each node in a binary search tree?
Each node of the tree now contains the attributes color, key, left, right, and p. If a child or the parent of a node does not exist, the corresponding pointer attribute of the node contains the value NIL. We shall regard these NILs as being pointers to leaves (external nodes) of the binary search tree and the normal, key-bearing nodes as being internal nodes of the tree.
What are the attributes of each node in a tree?
Each node of the tree now contains the attributes color, key, left, right, and p. If a child or the parent of a node does not exist, the corresponding pointer attribute of the node contains the value NIL. We shall regard these NILs
How many black nodes are there in a simple path?
5. For each node, all simple paths from the node to descendant leaves contain the same number of black nodes.
Why do B+ trees win?
B+ trees win them all, since tree depth is so much lower, causing much fewer random accesses to disk. Even more so, since the first two levels of the B+tree sit in the disk cache.
Is AVL better than red black?
AVL trees are more rigidly balanced than red black trees, leading to slower insertion and removal but faster retrieval. Red Black trees are good if there are similar number of insertions, deletions and lookups. For much larger number of lookups than insertions, you might want to look at the AVL tree.
Are we missing a good definition for Red-Black Tree? Don't keep it to yourself..
The ASL fingerspelling provided here is most commonly used for proper names of people and places; it is also used in some languages for concepts for which no sign is available at that moment.
Definitions & Translations
Get instant definitions for any word that hits you anywhere on the web!
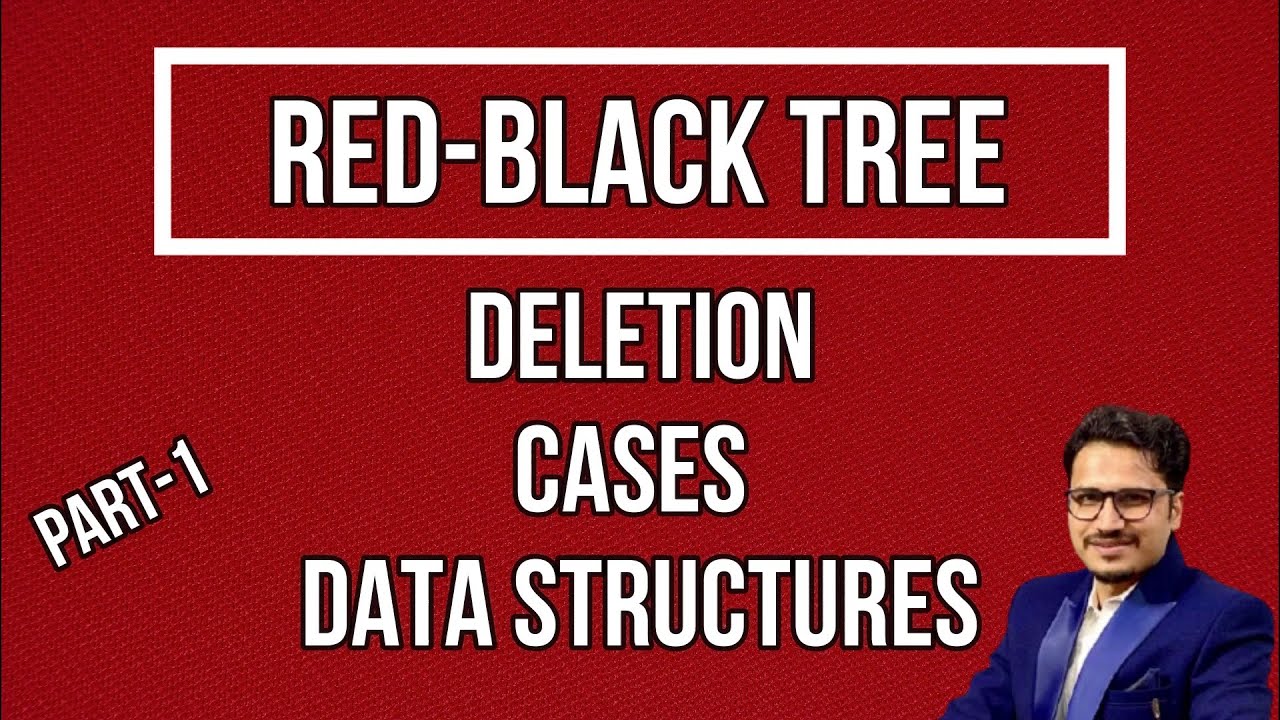
Properties of Red-Black Tree
- It is a self-balancing Binary Search tree. Here, self-balancing means that it balances the tree itself by either doing the rotations or recoloring the nodes.
- This tree data structure is named as a Red-Black tree as each node is either Red or Black in color. Every node stores one extra information known as a bit that represents the color of the node. For...
- It is a self-balancing Binary Search tree. Here, self-balancing means that it balances the tree itself by either doing the rotations or recoloring the nodes.
- This tree data structure is named as a Red-Black tree as each node is either Red or Black in color. Every node stores one extra information known as a bit that represents the color of the node. For...
- In the Red-Black tree, the root node is always black in color.
- In a binary tree, we consider those nodes as the leaf which have no child. In contrast, in the Red-Black tree, the nodes that have no child are considered the internal nodes and these nodes are con...
Is Every AVL Tree Can Be A Red-Black Tree?
- Yes, every AVL tree can be a Red-Black tree if we color each node either by Red or Black color. But every Red-Black tree is not an AVL because the AVL tree is strictly height-balanced while the Red-Black tree is not completely height-balanced.
Insertion in Red Black Tree
- The following are some rules used to create the Red-Black tree: 1. If the tree is empty, then we create a new node as a root node with the color black. 2. If the tree is not empty, then we create a new node as a leaf node with a color red. 3. If the parent of a new node is black, then exit. 4. If the parent of a new node is Red, then we have to check the color of the parent's sibling of a new nod…
Deletion in Red Back Tree
- Let's understand how we can delete the particular node from the Red-Black tree. The following are the rules used to delete the particular node from the tree: Step 1:First, we perform BST rules for the deletion. Step 2: Case 1:if the node is Red, which is to be deleted, we simply delete it. Let's understand case 1 through an example. Suppose we want to delete node 30 from the tree, whic…