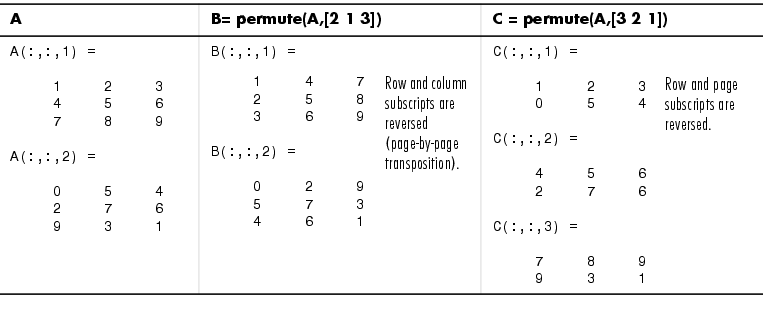
In a data frame the columns contain different types of data, but in a matrix all the elements are the same type of data. A matrix in R is like a mathematical matrix, containing all the same type of thing (usually numbers). R often but not always lets these be used interchangably.
How to create required matrix using Dataframe in R?
data.matrix () function in R Language is used to create a matrix by converting all the values of a Data Frame into numeric mode and then binding them as a matrix. df: Data frame to be converted.
How to combine two data frames in R?
- cbind () – combining the columns of two data frames side-by-side
- rbind () – stacking two data frames on top of each other, appending one to the other
- merge () – joining two data frames using a common column
How to convert list to Dataframe in R?
How to Convert a List to a Data Frame in R
- Method 1: Base R. In this example, sapply converts the list to a matrix, then data.frame converts the matrix to a data frame.
- Method 2: Data Table. This results in a data table with two rows and three columns. ...
- Method 3: Dplyr. This results in a data frame with two rows and three columns. ...
How to create Dataframe from NumPy arrays?
Example 2: Create DataFrame from Numpy Array with Column Names & Index
- Import pandas package and numpy package.
- Initialize a 2D Numpy Array.
- Initialize a List for column name of DataFrame.
- Initialize a List for index of DataFrame.
- Create DataFrame by passing this numpy array object for data parameter to pandas.DataFrame () constructor.
- pandas.DataFrame (ndarray) returns DataFrame.
See more

What's the difference between data frame and matrix?
Both represent 'rectangular' data types, meaning that they are used to store tabular data, with rows and columns. The main difference, as you'll see, is that matrices can only contain a single class of data, while data frames can consist of many different classes of data.
Is matrix faster than Dataframe in R?
Something not mentioned by @Michal is that not only is a matrix smaller than the equivalent data frame, using matrices can make your code far more efficient than using data frames, often considerably so. That is one reason why internally, a lot of R functions will coerce to matrices data that are in data frames.
What is the difference between Dataframe and table in R?
table vs data. frame in R Programming - GeeksforGeeks....Difference Table.data.tabledata.framedata.table is a rewritten form of data.frame in optimized c (or) data.table inherits from data.frame.data.frame is the base class in R and it is the default in R.7 more rows•Jan 20, 2021
Can you convert data frame to matrix in R?
Convert a Data Frame into a Numeric Matrix in R Programming – data. matrix() Function. data. matrix() function in R Language is used to create a matrix by converting all the values of a Data Frame into numeric mode and then binding them as a matrix.
What is the difference between data frame and matrix give example in support of your answer?
DataFrames is heterogeneous. All columns in a matrix must have the same data type (numeric, character, etc.) and the same length. A data frame is more general than a matrix, in that different columns can have different modes (numeric, character, factor, etc.).
What does as matrix do in R?
matrix() function in R Programming Language is used to convert an object into a Matrix.
What is a Dataframe in R?
A data frame is the most common way of storing data in R and, generally, is the data structure most often used for data analyses. Under the hood, a data frame is a list of equal-length vectors. Each element of the list can be thought of as a column and the length of each element of the list is the number of rows.
Is a Dataframe just a table?
A data frame is a table-like data structure available in languages like R and Python. Statisticians, scientists, and programmers use them in data analysis code.
How do I convert a Dataframe to a data table in R?
Method 1 : Using setDT() method The setDT() method can be used to coerce the dataframe or the lists into data. table, where the conversion is made to the original dataframe. The modification is made by reference to the original data structure.
Is a Dataframe a matrix?
DataFrames in R – It is a generalized form of a matrix. It is like a table in excel sheets. It has column and row names.
Can data frame convert to matrix?
Description. Return the matrix obtained by converting all the variables in a data frame to numeric mode and then binding them together as the columns of a matrix. Factors and ordered factors are replaced by their internal codes.
Is a data frame an array in R?
Convert data frame to array in R, A data frame is a table or a two-dimensional array-like structure in which each column has the values of a single variable and each row holds one set of values from each column.
What is the main difference between an array and a matrix in R?
A matrix is a two-dimensional (r × c) object (think a bunch of stacked or side-by-side vectors). An array is a three-dimensional (r × c × h) object (think a bunch of stacked r × c matrices). All elements in an array must be of the same data type (character > numeric > logical).
Why would you use a data frame over a vector to store your data?
One difference is that if we try to get a single row of the data frame, we get back a data frame with one row, rather than a vector. This is because the row may contain data of different types, and a vector can only hold elements of all the same type. Internally, a data frame is a list of column vectors.
What does as matrix () do when applied to a data frame with columns of different types?
matrix() do when applied to a data frame with columns of different types? A: From ?as. matrix : The method for data frames will return a character matrix if there is only atomic columns and any non-(numeric/logical/complex) column, applying as.
Is a DataFrame a matrix?
DataFrames in R – It is a generalized form of a matrix. It is like a table in excel sheets. It has column and row names.
What is a matrix in data structure?
As traditional data structure matrix is an mxn array with similar data type and data frame contains multiple data types in multiple columns called field.
What is a data frame?
A data frame combines features of matrices and lists. In fact, we can think of a data frame as a rectangular list, that is, a list in which all items have the length. The items of the list serve as the columns of the data frame, so every item within a particular column has to be of the same type.
What is tibble in R?
Tibble is a package for manipulating and printing data frames in R. They are a modern reimagining of the data.frame, keeping what time has proven to be effective, and throwing out what is not which means retaining all the important features of a dataframe and excluding all the out dated features.
What is matrix in math?
A matrix is a two-dimensional data structure. All the elements of a matrix must be of the same type (numeric, logical, character, complex). You can create a matrix
What is vector in R?
Vector is the fundamental data structure in R. The elements of a vector must all have the same mode or data type. You can have a vector consisting of three character strings (of mode character) or four integer elements (of mode integer), but not a vector with a mix of integer elements and character string elements.
What does parenthesis mean in R?
Parenthesis indicate a function being performed. For example: sum ( ) is a function. Notice the parenthesis? That tells R that ‘sum’ is not data or a variable, it is a function.
What rows are pulled in R?
Using brackets, we’ve told R to pull just rows 2 through 6, and only from column 2.
What is the difference between a matrix and a data frame?
If all you want to know is why they look different then the answer is that data frames and matrices are different classes and have different internal representations and although many operations have been designed and implemented to paper over the differences in the end matrix indexing and data frame indexing are separately implemented and do not necessarily have to be the same although hopefully they are implemented reasonably consistently. At this point it would likely be unwise to modify R to reduce any inconsistencies given how much code it might break.
Which is faster, matrices or data frames?
Typically operations on matrices are faster than operations on data frames.
Why is it important to be careful when converging a matrix to a data frame?
One has to be careful when convering a matrix to a data frame because if there are character and numeric columns in the data frame then the conversion will force them all to the same type, i.e. all to character.
What is the default for row names in data frame?
According the the documentation for data.frame () the default for row.names = NULL and the row names are set to the integer sequence starting at [1]. And the row names are not preserved by as.matrix (). When passing a data frame to as.matrix () the column names are preserved. Rownames are also automatically assigned as a sequence of integers if unassigned when using matrix ().
What is matrix in math?
matrix A matrix is a vector with dimensions.
Do matrix and data frames have row names?
Matrices do not have to have row or column names whereas data frames always do. If a matrix has row and column names then they are represented as a list of two vectors called dimnames (as opposed to a named list with a row.names attribute which is how data frames represent their row names).
Is a matrix numeric or character?
In the usual case all elements of a matrix are numeric, or all are character but for a data frame each column might be different. One column might be numeric whereas another might be character or logical.
Why are data frames useful?
This means, that adding a column is as easy as adding a vector to a list. It also means that while each column has its own data type , the columns can be of different types. This makes data frames useful for data storage.
Do R functions only work on data?
In R, some functions only work on a data.frame and others only on a tibble or a matrix.
What is a data frame in R?
Data Frame in R is a table or two-dimensional array-like structure in which a row contains a set of values, and each column holds values of one variable.
What is matrix in math?
A matrix is a two-dimensional rectangular data structure that can be created using a vector input to the matrix function. The as.matrix () function attempts to turn its argument into the matrix. The is.matrix () function tests if its argument is a (strict) matrix.
What is integer in R?
The integer () method creates or tests for objects of type “integer“. The current implementation of R uses the 32-bit integers for integer vectors. Integer vectors can have values like 21L, 19L, 0L, etc.
What is a raw vector?
The raw vector data type is intended to hold raw bytes. A raw vector is printed with each byte separately represented as a pair of hex digits. If you want to see a character representation (with escape sequences for non-printing characters), use the rawToChar () function.
What is the function as.raw?
The as.raw () function tries to curb its argument to be of raw type. The answer will be 0 unless the coercion succeeds.#N#The is.raw () function returns TRUE if and only if typeof (x) == “raw”.
How to specify complex vector in R?
The complex vector in R can be specified either by providing its length, its real and imaginary parts, or modulus and argument. The complex vector can have values like 1 + 2i, 2 + 5i, 1 + 0i, etc.
How many types of vector objects are there in R?
There are six types of vector objects available in R.
What is the difference between a matrix and a data frame?
Data frame can contain heterogeneous inputs while a matrix cannot. In a matrix, only similar data types can be stored whereas in a data frame there can be different data types like characters, integers, or other data frames. Basically Data Frame is advanced version of a Matrix where you can store various types of data input types.
What type of data is stored in a computer?
The data stored must be numeric, character or factor type.
Can columns be the same data type?
The data stored in columns can be only of same data type. The data stored must be numeric,
What is a matrix in a data frame?
Matrix is a special kind of vector. A matrix is a vector with two additional attributes: the number of rows and the number of columns. Similar to matrix, but arrays can have more than two dimensions. 3. List. List can contain elements of different types. 4. Date Frame. A data frame is used for storing data tables.
What is a data frame?
A data frame is used for storing data tables. It is a list of vectors of equal length.
What are the different types of data in R?
Vector, Array, List and Data Frame are 4 basic data types defined in R. Knowing the differences between them will help you use R more efficiently.
How many dimensions can an array have?
Similar to matrix, but arrays can have more than two dimensions.
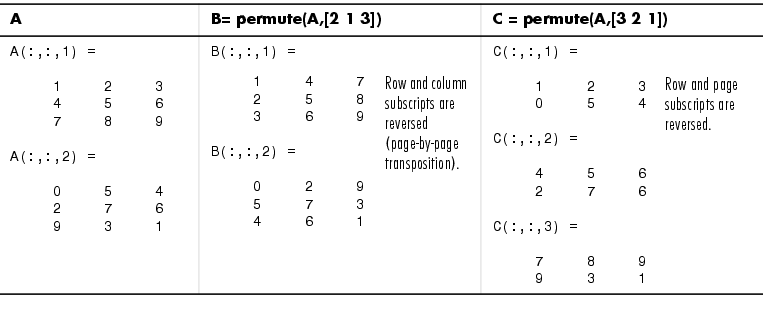