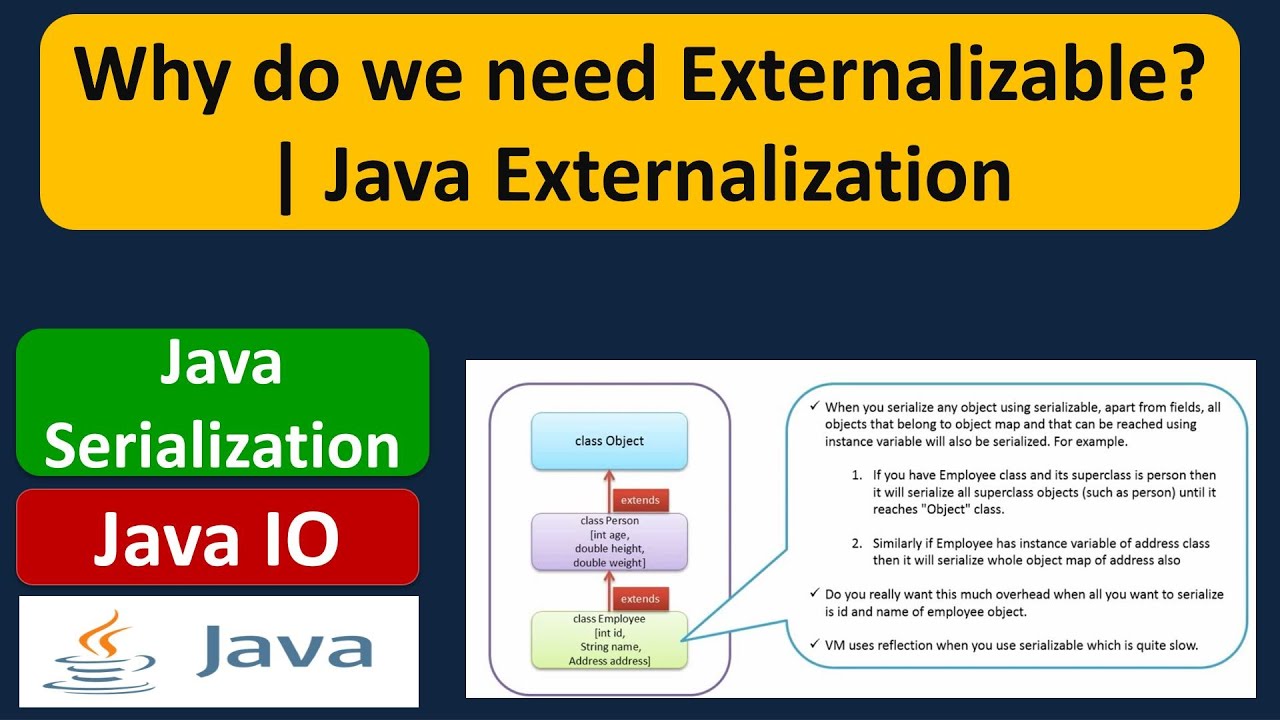
Why is externalization important in Java?
Why is externalization useful?
What is serialization in JMS?
What is serializable interface?
What is writeInt and writeObject?
How to implement externalizable interfaces?
What is the writeExternal method?
See 4 more
About this website
Understanding Java Externalization with Examples
About the Author: Nam Ha Minh is certified Java programmer (SCJP and SCWCD). He started programming with Java in the time of Java 1.4 and has been falling in love with Java since then. Make friend with him on Facebook and watch his Java videos you YouTube.
Difference between Serialization and Externalization in Java
Example of Externalizable class ExternalizableExample implements Externalizable { Integer id; @Override public void writeExternal(ObjectOutput out) throws IOException { out.writeInt( id ); } @Override public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException { this.id = in.readInt(); } }
Difference Between Serializable and Externalizable in Java ...
The process of writing the state of an object to a file is called serialization, but strictly speaking, it is the process of converting an object from java supported form into a file-supported form or network-supported form by using fileOutputStream and objectOutputStream classes we can implement serialization.. But we can serialize only serializable objects.
Externalization in Java | Externalization Interface with Example | Edureka
Java Serialization is not very efficient. If you serialize bloated objects having a lot of attributes and properties, you do not wish to serialize. This is where Externalization in Java comes into the picture. This article will help you understand the working of Externalization.
Externalizable interface in Java - GeeksforGeeks
Implementation : Unlike Serializable interface which will serialize the variables in object with just by implementing interface, here we have to explicitly mention what fields or variables you want to serialize. Methods : Serializable is marker interface without any methods. Externalizable interface contains two methods: writeExternal() and readExternal().
What is Externalizable in Java? - tutorialspoint.com
Externalization is used whenever we need to customize serialization mechanism. If a class implements an Externalizable interface then, object serialization will be done using writeExternal() method.. Whereas at receiver’s end when an Externalizable object is a reconstructed instance will be created using no argument constructor and then the readExternal() method is called.
What is serialization in Java?
In serialization, the Java Virtual Machine is totally responsible for the process of writing and reading objects. This is useful in most cases, as the programmers do not have to care about the underlying details of the serialization process. However, the default serialization does not protect sensitive information such as passwords and credentials, or what if the programmers want to secure some information during the serialization process? Thus externalization comes to give the programmers full control in reading and writing objects during serialization.
What is write external?
writeExternal (ObjectOutput out): The object implements this method to save its contents by calling the methods of DataOutput for its primitive values or calling the writeObject method of ObjectOutput for objects, strings, and arrays.
Does the password get blank after de-serialization?
As you can see in the output, the password gets blank and social security number is reset after de-serialization.
Who is Nam Ha Minh?
Nam Ha Minh is certified Java programmer (SCJP and SCWCD). He started programming with Java in the time of Java 1.4 and has been falling in love with Java since then. Make friend with him on Facebook and watch his Java videos you YouTube.
The Serializable interface
You can easily serialize any object if it implements the Serializable interface. This interface does not contain any methods, so it's just a sign for the compiler and the Java Virtual Machine (JVM) that this class is serializable.
Find the class
Let's assume you transfer an object into another host. Can it really be used there based on the information received from the network? Yes, it can, but only if the JVM knows about the class of the object.
The Externalizable interface
There might be times when you have special requirements for the serialization of an object. For example, you may have some security-sensitive parts of the object, like passwords, which you do not want to keep and transfer somewhere.
The "transient" keyword
If you don't need to save and restore any member variable (e.g., the password kept in a String object), the private modifier will not help you. Serialized information can be read in a file or in a captured network packet. You may implement the Externalizable interface, which is demonstrated in the previous paragraph.
Externalizing is faster
During serialization the JVM will always first check if the class is Externalizable. If that's the case then it will use the read/writeExternal methods. (makes sense, right)
Externalized output is more compact
If you would compare the actual output, it would look something like this: The header of the object contains a flag that marks if the class is just Serializable or maybe also Externalizable.
Externalizing is more flexible
If you save a shopping list, then you only want the product names, right ?
Design considerations
However, there are some dangers as well. Externalizable objects can only be implemented for objects with a public default constructor (a public constructor without arguments).
Hidden features
Just to be complete, there is one more thing which makes this topic a bit more complicated. It's actually possible to use read/write methods without using the Externalizable interface at all. Before Externalizable was introduced, it was possible to define private writeObject and readObject methods. But really, you shouldn't use that method anymore.
Why is externalization important in Java?
Externalization in Java is used to customize the serialization mechanism. Java serialization is not much efficient. When we have bloated objects that hold several attributes and properties, it is not good to serialize them. Here, the externalization will be more efficient.
Why is externalization useful?
The externalization is useful if we want to serialize a part of an object. We can serialize only the required fields of an object.
What is serialization in JMS?
Serialization is a mechanism of writing the state of an object into a byte-stream. The serialization is mainly used in Hibernate, RMI, JPA, EJB and JMS technologies. The mechanism that reverses the process of serialization is called deserialization. These processes are platform-independent means we can serialize objects in one platform and can deserialize them on other platforms.
What is serializable interface?
The Serializable interface is implemented to serialize the objects in Java.
What is writeInt and writeObject?
From the above code snippet, the writeInt () and writeObject () methods are used to serialize the code, name, password, and birthday values.
How to implement externalizable interfaces?
The Externalizable interfaces' objects are implemented using the readExternal () method. It restores objects by calling the methods of DataInput for primitive types. It can call readObject for objects, strings, and arrays data type.
What is the writeExternal method?
The writeExternal () method of the Externalizable interface is used to save the contents by calling the methods of dataOutput for primitive values. For the objects, strings, and arrays, call the writeObject method of ObjectOutput.
